


What are the trade-offs between using for...range and a traditional for loop in Go?
What are the trade-offs between using for...range and a traditional for loop in Go?
When considering the use of for...range
versus a traditional for
loop in Go, several trade-offs come into play:
Readability and Simplicity:
- For...range: This construct is more readable and concise, especially when iterating over slices, arrays, strings, maps, or channels. It abstracts away the index or key management, making the code cleaner and less error-prone.
- Traditional for loop: This requires manual index management, which can lead to more verbose code. However, it provides more control over the iteration process.
Performance:
-
For...range: Generally,
for...range
is slightly slower than a traditionalfor
loop due to the overhead of managing the iteration internally. However, the difference is often negligible for most use cases. -
Traditional for loop: Offers better performance in scenarios where every bit of speed counts, as it allows direct access to elements without the overhead of the
for...range
mechanism.
Flexibility:
- For...range: Less flexible as it follows a predefined pattern of iteration. It's ideal for straightforward iterations but can be limiting if you need to manipulate the iteration process.
- Traditional for loop: More flexible, allowing you to control the iteration in any way you see fit, including skipping elements, iterating in reverse, or modifying the collection during iteration.
Safety:
- For...range: Safer to use, especially with slices and arrays, as it avoids common errors like off-by-one errors or out-of-bounds access.
- Traditional for loop: More prone to errors if not managed carefully, particularly with manual index management.
In summary, for...range
offers simplicity and safety at the cost of slight performance overhead, while a traditional for
loop provides more control and potentially better performance but requires more careful management.
Which loop construct in Go, for...range or traditional for loop, offers better performance for large data sets?
For large data sets, a traditional for
loop typically offers better performance compared to a for...range
loop. The reason is that a traditional for
loop allows direct access to elements without the additional overhead that comes with the for...range
construct. This overhead includes the internal management of the iteration process, which, while small, can add up when dealing with large data sets.
However, it's important to note that the performance difference may not be significant enough to justify the use of a traditional for
loop in all cases. Benchmarking your specific use case is recommended to determine if the performance gain is worth the potential trade-offs in readability and safety.
In what scenarios would a traditional for loop be more suitable than a for...range loop in Go?
A traditional for
loop would be more suitable than a for...range
loop in the following scenarios:
Need for Fine-Grained Control:
- When you need to manipulate the iteration process, such as skipping elements, iterating in reverse, or modifying the collection during iteration. A traditional
for
loop provides the flexibility to do so.
Performance-Critical Applications:
- In applications where every bit of performance counts, a traditional
for
loop can offer a slight performance advantage due to its direct access to elements without the overhead offor...range
.
Complex Indexing or Multiple Iterators:
- When you need to use multiple iterators or complex indexing patterns that are not easily achievable with
for...range
.
Modifying the Collection During Iteration:
- If you need to modify the collection (e.g., a slice or array) while iterating over it, a traditional
for
loop is necessary becausefor...range
does not allow safe modification of the collection during iteration.
Iterating Over Non-Collection Types:
- When you need to iterate over a range of numbers or other non-collection types, a traditional
for
loop is more appropriate.
When should you prefer using a for...range loop over a traditional for loop in Go for iterating over collections?
You should prefer using a for...range
loop over a traditional for
loop in Go for iterating over collections in the following scenarios:
Iterating Over Slices, Arrays, Strings, Maps, or Channels:
-
For...range
is designed to work seamlessly with these data structures, providing a clean and concise way to iterate over them without worrying about index management.
Readability and Maintainability:
- When code readability and maintainability are priorities,
for...range
offers a more straightforward and less error-prone way to iterate over collections.
Avoiding Common Errors:
- To avoid common errors such as off-by-one errors or out-of-bounds access,
for...range
is safer and more reliable.
Simple Iterations:
- For straightforward iterations where you don't need to manipulate the iteration process,
for...range
is the preferred choice due to its simplicity and ease of use.
Concurrent Programming:
- When working with channels in concurrent programming,
for...range
provides a clean way to iterate over received values without the need for manual management.
In summary, for...range
should be your go-to choice for iterating over collections when simplicity, readability, and safety are more important than the slight performance advantage offered by a traditional for
loop.
The above is the detailed content of What are the trade-offs between using for...range and a traditional for loop in Go?. For more information, please follow other related articles on the PHP Chinese website!
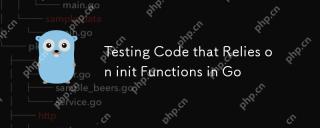
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
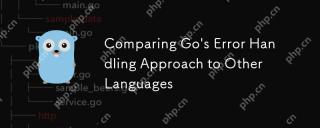
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
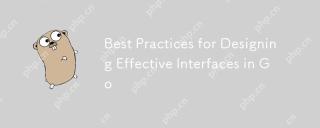
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
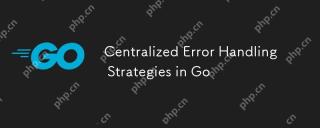
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.
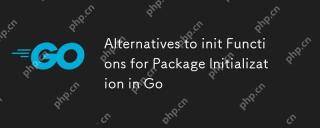
InGo,alternativestoinitfunctionsincludecustominitializationfunctionsandsingletons.1)Custominitializationfunctionsallowexplicitcontroloverwheninitializationoccurs,usefulfordelayedorconditionalsetups.2)Singletonsensureone-timeinitializationinconcurrent
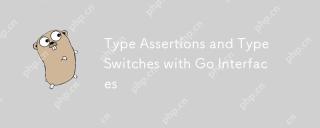
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
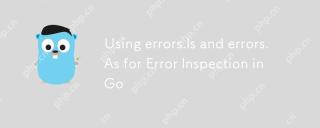
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
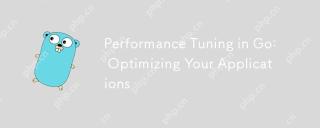
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
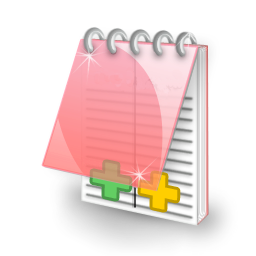
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
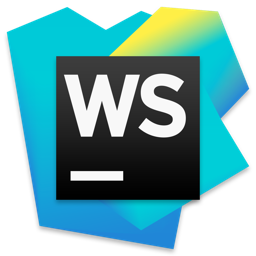
WebStorm Mac version
Useful JavaScript development tools
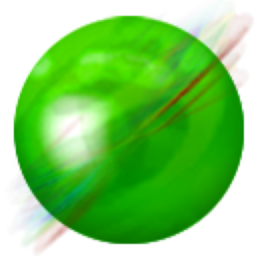
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
