


How do you approach designing a new system or feature in Python?
When designing a new system or feature in Python, I follow a structured approach to ensure that the end result is both functional and maintainable. Here are the steps I typically take:
- Define Requirements: The first step is to clearly define the requirements of the system or feature. This involves understanding the problem domain, identifying the key functionalities, and setting clear objectives. I often use user stories or requirement documents to capture these details.
- Research and Planning: Once the requirements are clear, I conduct research to understand existing solutions, best practices, and any relevant libraries or frameworks that could be utilized. This phase also involves sketching out high-level designs and planning the overall architecture.
- Prototyping: I create a prototype to test the feasibility of the design. This could be a simple script or a more complex mock-up, depending on the complexity of the system. Prototyping helps in identifying potential issues early in the development process.
- Detailed Design: With the prototype in hand, I move on to the detailed design phase. This involves creating detailed diagrams (such as UML diagrams), defining the data structures, and outlining the algorithms to be used. I also consider the modularity of the code and how different components will interact.
- Implementation: The actual coding begins once the design is finalized. I follow best practices such as writing clean, modular code, and adhering to PEP 8 style guidelines. I also ensure that the code is well-documented and includes appropriate comments.
- Testing and Refinement: After the initial implementation, I conduct thorough testing to ensure that the system or feature meets the defined requirements. This includes unit testing, integration testing, and possibly user acceptance testing. Based on the test results, I refine the design and implementation as needed.
- Review and Iteration: Finally, I conduct a review of the entire process, gather feedback, and iterate on the design if necessary. This iterative approach helps in continuously improving the system or feature.
What are the key considerations when planning the architecture of a Python project?
When planning the architecture of a Python project, several key considerations come into play:
- Scalability: The architecture should be designed to handle growth in terms of data volume, user base, and functionality. This might involve using scalable data storage solutions, implementing efficient algorithms, and designing for horizontal scaling.
- Modularity: A modular architecture allows for easier maintenance and updates. This can be achieved by breaking down the system into smaller, independent components or modules that can be developed, tested, and maintained separately.
- Reusability: Designing for reusability helps in reducing redundancy and improving efficiency. This involves creating reusable components and libraries that can be used across different parts of the project or even in other projects.
- Performance: The architecture should be optimized for performance, considering factors such as response times, resource utilization, and throughput. This might involve choosing the right data structures, algorithms, and possibly using asynchronous programming techniques.
- Security: Security considerations are crucial, especially for systems that handle sensitive data. This includes implementing proper authentication and authorization mechanisms, securing data at rest and in transit, and following security best practices.
- Maintainability: The architecture should be easy to maintain and update. This involves writing clean, well-documented code, following design patterns, and using tools that support code quality and maintainability.
- Integration: Consider how the system will integrate with other systems or services. This might involve designing APIs, using microservices architecture, or ensuring compatibility with existing infrastructure.
- Testing: The architecture should facilitate testing, including unit testing, integration testing, and possibly automated testing. This involves designing the system in a way that makes it easy to isolate and test individual components.
How do you ensure your Python code remains maintainable and scalable as the project grows?
Ensuring that Python code remains maintainable and scalable as the project grows involves several strategies:
- Adherence to Best Practices: Following best practices such as writing clean, modular code, adhering to PEP 8 style guidelines, and using meaningful variable and function names helps in maintaining code quality.
- Modular Design: Breaking down the system into smaller, independent modules makes it easier to maintain and update individual components without affecting the entire system. This also facilitates parallel development and testing.
- Documentation: Writing comprehensive documentation, including docstrings and comments, helps other developers understand the code and its purpose. This is crucial for maintaining the codebase over time.
- Code Reviews: Regular code reviews help in identifying and fixing issues early, ensuring that the code adheres to the project's standards and best practices. This also promotes knowledge sharing among team members.
- Refactoring: Regularly refactoring the code to improve its structure and efficiency helps in keeping the codebase clean and maintainable. This involves removing redundant code, simplifying complex logic, and optimizing performance.
- Testing: Implementing a robust testing strategy, including unit tests, integration tests, and possibly automated tests, ensures that changes to the code do not introduce new bugs. This also helps in maintaining the scalability of the system.
- Continuous Integration and Deployment (CI/CD): Using CI/CD pipelines helps in automating the testing and deployment process, ensuring that changes are thoroughly tested before being deployed to production. This also helps in maintaining the scalability of the system.
- Performance Monitoring: Regularly monitoring the performance of the system helps in identifying bottlenecks and areas for improvement. This involves using tools to track metrics such as response times, resource utilization, and throughput.
What tools or methodologies do you use to test and refine your Python designs during development?
To test and refine Python designs during development, I use a combination of tools and methodologies:
-
Unit Testing: I use the
unittest
module or third-party frameworks likepytest
to write and run unit tests. Unit tests help in verifying that individual components of the system work as expected. -
Integration Testing: For testing how different components interact, I use integration tests. This can be done using frameworks like
pytest
with plugins such aspytest-django
for Django projects. - Automated Testing: I set up automated testing pipelines using tools like Jenkins, Travis CI, or GitHub Actions. These pipelines run tests automatically whenever code changes are pushed to the repository, ensuring that the system remains stable.
-
Code Coverage Tools: I use tools like
coverage.py
to measure the code coverage of my tests. This helps in identifying areas of the code that are not adequately tested and need more attention. -
Static Code Analysis: Tools like
pylint
,flake8
, andmypy
help in identifying potential issues in the code, such as style violations, bugs, and type errors. These tools help in maintaining code quality and catching issues early. -
Profiling and Performance Testing: For performance testing, I use tools like
cProfile
orline_profiler
to identify bottlenecks and optimize the code. This helps in refining the design to improve performance. - User Acceptance Testing (UAT): For systems that involve user interaction, I conduct UAT to ensure that the system meets the user's needs and expectations. This involves creating test scenarios and getting feedback from actual users.
- Agile Methodologies: I follow agile methodologies such as Scrum or Kanban to iteratively develop and refine the design. This involves regular sprints, stand-ups, and retrospectives to continuously improve the system.
- Design Patterns and Refactoring: I use design patterns and refactoring techniques to improve the design of the system. This involves applying patterns like Singleton, Factory, or Observer to solve common design problems and refactoring the code to improve its structure and efficiency.
By combining these tools and methodologies, I ensure that the Python designs are thoroughly tested and refined during development, leading to a robust and maintainable system.
The above is the detailed content of How do you approach designing a new system or feature in Python?. For more information, please follow other related articles on the PHP Chinese website!
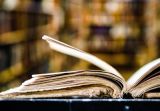
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
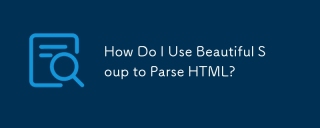
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
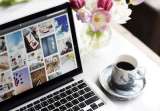
Dealing with noisy images is a common problem, especially with mobile phone or low-resolution camera photos. This tutorial explores image filtering techniques in Python using OpenCV to tackle this issue. Image Filtering: A Powerful Tool Image filter
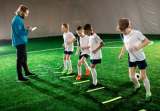
Python, a favorite for data science and processing, offers a rich ecosystem for high-performance computing. However, parallel programming in Python presents unique challenges. This tutorial explores these challenges, focusing on the Global Interprete
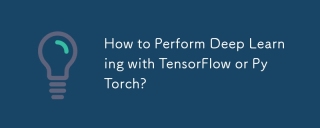
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
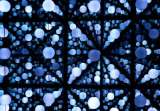
This tutorial demonstrates creating a custom pipeline data structure in Python 3, leveraging classes and operator overloading for enhanced functionality. The pipeline's flexibility lies in its ability to apply a series of functions to a data set, ge
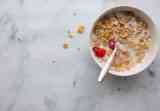
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
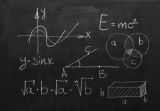
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor

SublimeText3 Linux new version
SublimeText3 Linux latest version
