


How can you use Go's sync.Pool to reuse objects and reduce garbage collection overhead?
Go's sync.Pool
is a built-in mechanism designed to help reduce the pressure on the garbage collector by allowing the reuse of temporary objects. Here's how you can use sync.Pool
to achieve this:
-
Initialization: First, you need to initialize a
sync.Pool
with aNew
function that returns a new instance of the object you want to reuse. This function is called when the pool is empty and a new object is needed.var bytePool = sync.Pool{ New: func() interface{} { b := make([]byte, 1024) return &b }, }
-
Getting an Object: To get an object from the pool, you use the
Get
method. This method returns an object if one is available in the pool, or it creates a new one using theNew
function if the pool is empty.obj := bytePool.Get().(*[]byte)
- Using the Object: After retrieving an object from the pool, you can use it as needed in your application.
-
Returning the Object: Once you're done using the object, you should return it to the pool using the
Put
method. This allows the object to be reused later, reducing the need for new allocations.bytePool.Put(obj)
By reusing objects in this way, you can significantly reduce the number of allocations and deallocations, which in turn reduces the frequency and duration of garbage collection pauses, improving the overall performance of your Go application.
What are the best practices for managing object lifecycles with sync.Pool in Go?
Managing object lifecycles with sync.Pool
effectively requires adherence to several best practices:
-
Use for Short-Lived Objects:
sync.Pool
is best suited for short-lived objects that are frequently allocated and deallocated. Long-lived objects should not be managed withsync.Pool
. - Avoid Storing State: Objects in the pool should not retain state between uses. Ensure that any state is reset or cleared before returning the object to the pool.
-
Thread Safety:
sync.Pool
is safe for concurrent use, but the objects themselves may not be. Ensure that any operations on the objects are thread-safe if they will be used concurrently. -
Proper Initialization: Always provide a
New
function that initializes the object correctly. This ensures that even if the pool is empty, a valid object can be created. -
Avoid Complex Objects:
sync.Pool
is most effective with simple, lightweight objects. Complex objects with many dependencies or initialization steps may not benefit as much from pooling. - Monitor and Tune: Use profiling tools to monitor the effectiveness of your pools. Adjust the size and type of objects in the pool based on your application's needs.
How does sync.Pool help in improving the performance of Go applications?
sync.Pool
can significantly improve the performance of Go applications in several ways:
-
Reduced Garbage Collection Overhead: By reusing objects,
sync.Pool
reduces the number of allocations and deallocations, which in turn reduces the frequency and duration of garbage collection pauses. This leads to more predictable and stable performance. - Lower Memory Usage: Reusing objects means fewer new allocations, which can lead to lower overall memory usage. This is particularly beneficial in memory-constrained environments.
- Improved Throughput: With fewer garbage collection pauses and less time spent on allocations, applications can process more requests or perform more operations per unit of time, leading to higher throughput.
- Better Cache Utilization: Reusing objects can improve cache utilization, as the same memory locations are accessed more frequently, leading to better performance on modern CPU architectures.
-
Reduced Latency: By minimizing the time spent on garbage collection and allocations,
sync.Pool
can help reduce the latency of individual operations, making applications more responsive.
What are the potential pitfalls to avoid when using sync.Pool for object reuse in Go?
While sync.Pool
can be a powerful tool, there are several potential pitfalls to be aware of:
-
Overuse: Using
sync.Pool
for objects that are not frequently allocated and deallocated can lead to unnecessary complexity and potential performance issues. - State Retention: If objects retain state between uses, it can lead to bugs and unexpected behavior. Always ensure that objects are properly reset before being returned to the pool.
-
Thread Safety Issues: While
sync.Pool
itself is thread-safe, the objects it manages may not be. Ensure that any operations on the objects are safe for concurrent use. - Memory Leaks: If objects are not properly returned to the pool, it can lead to memory leaks. Always ensure that objects are returned to the pool when they are no longer needed.
-
Over-Optimization: Relying too heavily on
sync.Pool
can lead to over-optimization, where the complexity of managing the pool outweighs the benefits. Use profiling and benchmarking to ensure thatsync.Pool
is actually improving performance. -
Inappropriate Use Cases:
sync.Pool
is not suitable for all use cases. For example, it should not be used for long-lived objects or objects that require complex initialization.
By being aware of these pitfalls and following best practices, you can effectively use sync.Pool
to improve the performance and efficiency of your Go applications.
The above is the detailed content of How can you use Go's sync.Pool to reuse objects and reduce garbage collection overhead?. For more information, please follow other related articles on the PHP Chinese website!
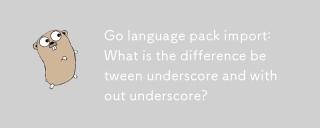
This article explains Go's package import mechanisms: named imports (e.g., import "fmt") and blank imports (e.g., import _ "fmt"). Named imports make package contents accessible, while blank imports only execute t

This article explains Beego's NewFlash() function for inter-page data transfer in web applications. It focuses on using NewFlash() to display temporary messages (success, error, warning) between controllers, leveraging the session mechanism. Limita
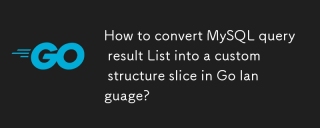
This article details efficient conversion of MySQL query results into Go struct slices. It emphasizes using database/sql's Scan method for optimal performance, avoiding manual parsing. Best practices for struct field mapping using db tags and robus
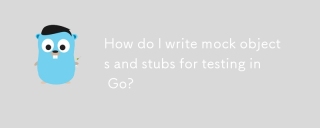
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
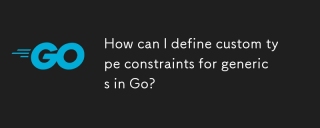
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices

This article details efficient file writing in Go, comparing os.WriteFile (suitable for small files) with os.OpenFile and buffered writes (optimal for large files). It emphasizes robust error handling, using defer, and checking for specific errors.
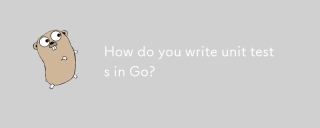
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
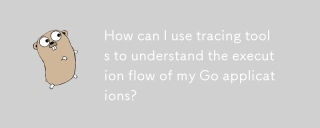
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
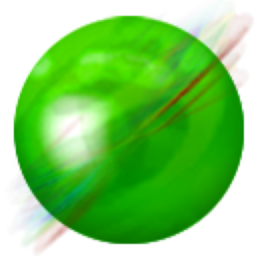
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
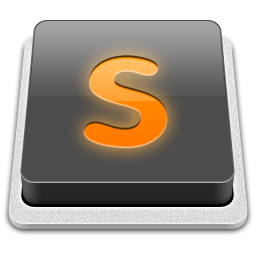
SublimeText3 Mac version
God-level code editing software (SublimeText3)
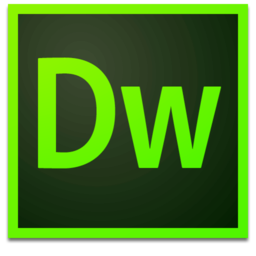
Dreamweaver Mac version
Visual web development tools
