How do you benchmark Go code using the testing package?
Benchmarking in Go is facilitated through the testing
package, which provides a simple yet powerful way to measure the performance of your code. To write a benchmark, you use the Benchmark
function prefix followed by a name that describes what the benchmark tests. Here's a basic example of how to write a benchmark:
package main import "testing" func BenchmarkMyFunction(b *testing.B) { for i := 0; i < b.N; i { MyFunction() } }
In this example, b.N
is a number set by the testing package that indicates how many times the function should be run. The testing package will adjust b.N
to get accurate measurements.
To run the benchmark, you use the go test
command with the -bench
flag. For example:
go test -bench=BenchmarkMyFunction
This command will run the benchmark and output the results, showing the time taken per operation.
What are the best practices for writing effective benchmarks in Go?
Writing effective benchmarks in Go involves several best practices to ensure accurate and meaningful results:
- Isolate the Code Being Benchmarked: Ensure that the benchmark only measures the code you're interested in. Avoid including setup or teardown code within the benchmark loop.
-
Use
b.ResetTimer()
: If you need to perform setup operations before the actual benchmark, useb.ResetTimer()
to reset the timer after setup and before the actual benchmark code.func BenchmarkMyFunction(b *testing.B) { // Setup code b.ResetTimer() for i := 0; i < b.N; i { MyFunction() } }
- Avoid Memory Allocations Inside the Loop: Memory allocations can skew benchmark results. Try to allocate memory outside the benchmark loop if possible.
-
Use
b.StopTimer()
andb.StartTimer()
: If you need to perform operations that should not be included in the benchmark, you can stop and start the timer around those operations.func BenchmarkMyFunction(b *testing.B) { for i := 0; i < b.N; i { b.StopTimer() // Some operation not to be measured b.StartTimer() MyFunction() } }
-
Run Benchmarks Multiple Times: Use the
-count
flag to run benchmarks multiple times to account for variability.go test -bench=BenchmarkMyFunction -count=5
-
Use
b.ReportAllocs()
: To measure memory allocations, useb.ReportAllocs()
at the beginning of your benchmark function.func BenchmarkMyFunction(b *testing.B) { b.ReportAllocs() for i := 0; i < b.N; i { MyFunction() } }
How can you analyze and interpret benchmark results in Go?
Analyzing and interpreting benchmark results in Go involves understanding the output provided by the go test
command. Here's how to interpret the typical output:
BenchmarkMyFunction-8 1000000 123 ns/op
- BenchmarkMyFunction-8: The name of the benchmark and the GOMAXPROCS value (8 in this case).
-
1000000: The number of iterations (
b.N
) the benchmark ran. - 123 ns/op: The average time per operation in nanoseconds.
To analyze the results more deeply:
- Compare Different Implementations: Run benchmarks for different implementations of the same function to compare their performance.
-
Use
-benchmem
Flag: This flag provides memory allocation statistics.go test -bench=BenchmarkMyFunction -benchmem
The output might look like this:
BenchmarkMyFunction-8 1000000 123 ns/op 16 B/op 1 allocs/op
- 16 B/op: The average number of bytes allocated per operation.
- 1 allocs/op: The average number of allocations per operation.
- Analyze Trends Over Time: Use version control to track changes in benchmark results over time, helping to identify performance regressions.
-
Use Benchmark Comparison Tools: Tools like
benchstat
can help compare benchmark results across different runs or versions of your code.
What tools can be used alongside Go's testing package to enhance benchmarking?
Several tools can enhance the benchmarking capabilities provided by Go's testing package:
-
benchstat: A tool from the Go team that helps compare benchmark results across different runs. It can be installed using:
go get golang.org/x/perf/cmd/benchstat
You can use it to compare two sets of benchmark results:
benchstat old.txt new.txt
-
pprof: Go's built-in profiling tool that can be used to analyze CPU and memory usage. You can enable CPU profiling in your benchmark with:
func BenchmarkMyFunction(b *testing.B) { b.Run("CPU", func(b *testing.B) { b.SetParallelism(1) b.ReportAllocs() b.ResetTimer() for i := 0; i < b.N; i { MyFunction() } }) }
Then run the benchmark with profiling enabled:
go test -bench=BenchmarkMyFunction -cpuprofile cpu.out
You can then analyze the profile with:
go tool pprof cpu.out
-
Benchmark Plot: A tool for visualizing benchmark results over time. It can be installed with:
go get github.com/ajstarks/svgo/benchplot
You can use it to generate plots from benchmark results:
benchplot -t "My Benchmark" -o mybenchmark.png old.txt new.txt
-
Go-Torch: A tool for visualizing Go execution traces. It can be installed with:
go get github.com/uber/go-torch
You can generate a trace with:
go test -bench=BenchmarkMyFunction -trace trace.out
And then visualize it with:
go-torch trace.out
These tools, when used alongside Go's testing package, can provide a comprehensive view of your code's performance and help you optimize it effectively.
The above is the detailed content of How do you benchmark Go code using the testing package?. For more information, please follow other related articles on the PHP Chinese website!
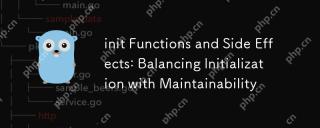
Toensureinitfunctionsareeffectiveandmaintainable:1)Minimizesideeffectsbyreturningvaluesinsteadofmodifyingglobalstate,2)Ensureidempotencytohandlemultiplecallssafely,and3)Breakdowncomplexinitializationintosmaller,focusedfunctionstoenhancemodularityandm
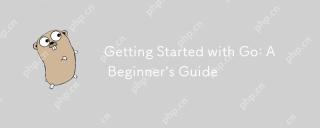
Goisidealforbeginnersandsuitableforcloudandnetworkservicesduetoitssimplicity,efficiency,andconcurrencyfeatures.1)InstallGofromtheofficialwebsiteandverifywith'goversion'.2)Createandrunyourfirstprogramwith'gorunhello.go'.3)Exploreconcurrencyusinggorout
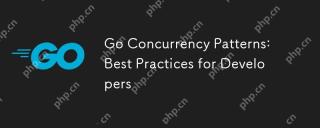
Developers should follow the following best practices: 1. Carefully manage goroutines to prevent resource leakage; 2. Use channels for synchronization, but avoid overuse; 3. Explicitly handle errors in concurrent programs; 4. Understand GOMAXPROCS to optimize performance. These practices are crucial for efficient and robust software development because they ensure effective management of resources, proper synchronization implementation, proper error handling, and performance optimization, thereby improving software efficiency and maintainability.
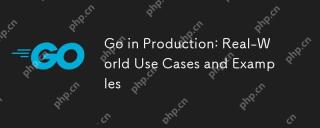
Goexcelsinproductionduetoitsperformanceandsimplicity,butrequirescarefulmanagementofscalability,errorhandling,andresources.1)DockerusesGoforefficientcontainermanagementthroughgoroutines.2)UberscalesmicroserviceswithGo,facingchallengesinservicemanageme
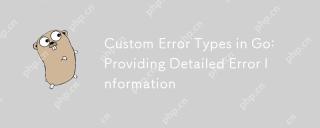
We need to customize the error type because the standard error interface provides limited information, and custom types can add more context and structured information. 1) Custom error types can contain error codes, locations, context data, etc., 2) Improve debugging efficiency and user experience, 3) But attention should be paid to its complexity and maintenance costs.
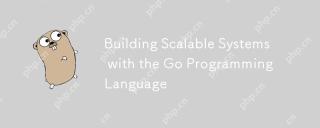
Goisidealforbuildingscalablesystemsduetoitssimplicity,efficiency,andbuilt-inconcurrencysupport.1)Go'scleansyntaxandminimalisticdesignenhanceproductivityandreduceerrors.2)Itsgoroutinesandchannelsenableefficientconcurrentprogramming,distributingworkloa
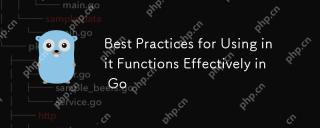
InitfunctionsinGorunautomaticallybeforemain()andareusefulforsettingupenvironmentsandinitializingvariables.Usethemforsimpletasks,avoidsideeffects,andbecautiouswithtestingandloggingtomaintaincodeclarityandtestability.
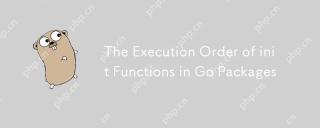
Goinitializespackagesintheordertheyareimported,thenexecutesinitfunctionswithinapackageintheirdefinitionorder,andfilenamesdeterminetheorderacrossmultiplefiles.Thisprocesscanbeinfluencedbydependenciesbetweenpackages,whichmayleadtocomplexinitializations


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
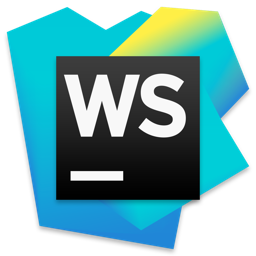
WebStorm Mac version
Useful JavaScript development tools

Atom editor mac version download
The most popular open source editor
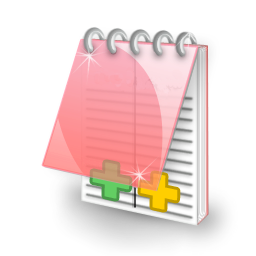
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
