How do you create a TCP server and client in Go?
Creating a TCP server and client in Go involves a few straightforward steps. Below, I'll explain how to set up both components.
TCP Server in Go
-
Import the necessary package:
You need to import thenet
package, which provides the necessary functions for network programming.import "net"
-
Create a listener:
Usenet.Listen
to create a listener on a specific network address and port.listener, err := net.Listen("tcp", ":8080") if err != nil { // Handle error } defer listener.Close()
-
Accept incoming connections:
Use a loop to accept incoming connections using theAccept
method of the listener.for { conn, err := listener.Accept() if err != nil { // Handle error } go handleConnection(conn) }
-
Handle connections:
Create a function to handle each connection. This function can read from and write to the connection.func handleConnection(conn net.Conn) { defer conn.Close() // Read and write to the connection buffer := make([]byte, 1024) for { n, err := conn.Read(buffer) if err != nil { // Handle error return } // Process the data // Write back to the client _, err = conn.Write(buffer[:n]) if err != nil { // Handle error return } } }
TCP Client in Go
-
Import the necessary package:
Again, you need to import thenet
package.import "net"
-
Establish a connection:
Usenet.Dial
to connect to the server.conn, err := net.Dial("tcp", "localhost:8080") if err != nil { // Handle error } defer conn.Close()
-
Send and receive data:
Use the connection to send and receive data.// Send data _, err = conn.Write([]byte("Hello, server!")) if err != nil { // Handle error } // Receive data buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { // Handle error } fmt.Println(string(buffer[:n]))
What are the essential steps to establish a TCP connection in Go?
Establishing a TCP connection in Go involves several key steps, which are essential for both the server and the client:
-
Import the
net
package:
This package provides the necessary functions for network programming.import "net"
-
Server: Create a listener:
Usenet.Listen
to create a listener on a specific network address and port.listener, err := net.Listen("tcp", ":8080") if err != nil { // Handle error } defer listener.Close()
-
Server: Accept incoming connections:
Use a loop to accept incoming connections using theAccept
method of the listener.for { conn, err := listener.Accept() if err != nil { // Handle error } // Handle the connection }
-
Client: Establish a connection:
Usenet.Dial
to connect to the server.conn, err := net.Dial("tcp", "localhost:8080") if err != nil { // Handle error } defer conn.Close()
-
Send and receive data:
Both the server and client can use the connection to send and receive data.// Send data _, err = conn.Write([]byte("Hello, server!")) if err != nil { // Handle error } // Receive data buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { // Handle error } fmt.Println(string(buffer[:n]))
How can you handle multiple client connections in a Go TCP server?
Handling multiple client connections in a Go TCP server can be achieved using goroutines. Here's how you can do it:
-
Create a listener:
Usenet.Listen
to create a listener on a specific network address and port.listener, err := net.Listen("tcp", ":8080") if err != nil { // Handle error } defer listener.Close()
-
Accept incoming connections in a loop:
Use a loop to accept incoming connections using theAccept
method of the listener.for { conn, err := listener.Accept() if err != nil { // Handle error } // Handle the connection in a new goroutine go handleConnection(conn) }
-
Handle connections in goroutines:
Create a function to handle each connection. This function will run in its own goroutine, allowing the server to handle multiple clients concurrently.func handleConnection(conn net.Conn) { defer conn.Close() // Read and write to the connection buffer := make([]byte, 1024) for { n, err := conn.Read(buffer) if err != nil { // Handle error return } // Process the data // Write back to the client _, err = conn.Write(buffer[:n]) if err != nil { // Handle error return } } }
By using goroutines, the server can handle multiple client connections simultaneously, improving its scalability and performance.
What common errors should you watch out for when implementing TCP communications in Go?
When implementing TCP communications in Go, there are several common errors you should watch out for:
-
Connection Errors:
-
Dialing Errors: When using
net.Dial
, you might encounter errors if the server is not running or if the network address is incorrect.conn, err := net.Dial("tcp", "localhost:8080") if err != nil { // Handle error }
-
Accepting Errors: When using
listener.Accept
, you might encounter errors if the server is unable to accept new connections.conn, err := listener.Accept() if err != nil { // Handle error }
-
-
Read/Write Errors:
-
Read Errors: When reading from a connection using
conn.Read
, you might encounter errors if the connection is closed or if there is a network issue.n, err := conn.Read(buffer) if err != nil { // Handle error }
-
Write Errors: When writing to a connection using
conn.Write
, you might encounter errors if the connection is closed or if there is a network issue._, err = conn.Write(buffer[:n]) if err != nil { // Handle error }
-
-
Resource Management:
-
Closing Connections: It's important to close connections properly to avoid resource leaks. Use
defer
to ensure connections are closed even if an error occurs.defer conn.Close()
-
Closing Listeners: Similarly, ensure that listeners are closed properly.
defer listener.Close()
-
-
Concurrency Issues:
- Race Conditions: When handling multiple connections using goroutines, be aware of potential race conditions. Use synchronization primitives like mutexes if necessary.
- Deadlocks: Be cautious of deadlocks when using synchronization primitives. Ensure that locks are released properly.
-
Buffer Management:
-
Buffer Sizes: Choose appropriate buffer sizes for reading and writing data. Too small a buffer can lead to inefficient communication, while too large a buffer can waste memory.
buffer := make([]byte, 1024)
-
By being aware of these common errors and handling them appropriately, you can create more robust and reliable TCP communications in Go.
The above is the detailed content of How do you create a TCP server and client in Go?. For more information, please follow other related articles on the PHP Chinese website!
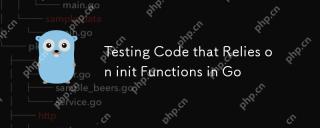
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
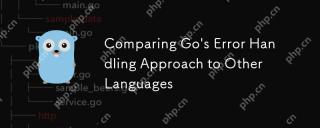
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
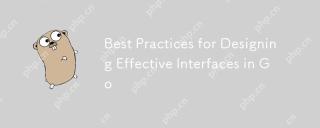
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
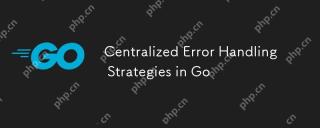
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.
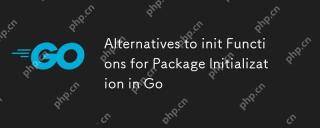
InGo,alternativestoinitfunctionsincludecustominitializationfunctionsandsingletons.1)Custominitializationfunctionsallowexplicitcontroloverwheninitializationoccurs,usefulfordelayedorconditionalsetups.2)Singletonsensureone-timeinitializationinconcurrent
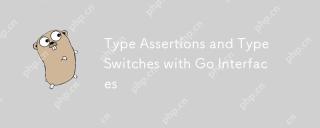
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
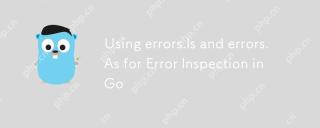
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
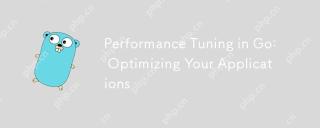
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
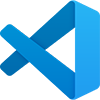
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
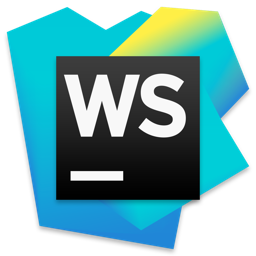
WebStorm Mac version
Useful JavaScript development tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Linux new version
SublimeText3 Linux latest version
