


How can you use goroutine pools to limit the number of concurrent goroutines?
Goroutine pools are used to manage and limit the number of concurrent goroutines in a Go program. This is particularly useful when you want to control the level of concurrency to prevent overwhelming system resources or to ensure that the program behaves predictably under high load. Here's how you can use goroutine pools to achieve this:
- Creation of a Pool: First, you create a pool of goroutines. This pool typically consists of a fixed number of worker goroutines that are ready to execute tasks.
- Task Submission: When a task needs to be executed, it is submitted to the pool. The pool manages a queue of tasks waiting to be processed.
- Task Execution: The worker goroutines in the pool pick up tasks from the queue and execute them. Since the number of worker goroutines is fixed, the number of concurrently running goroutines is limited to the size of the pool.
- Completion and Reuse: Once a worker goroutine completes a task, it returns to the pool and is ready to pick up another task. This allows for efficient reuse of goroutines.
By using a goroutine pool, you ensure that no more than the specified number of goroutines are running at any given time, thus controlling the level of concurrency.
What are the benefits of using goroutine pools for managing concurrency in Go?
Using goroutine pools to manage concurrency in Go offers several benefits:
- Resource Management: Goroutine pools help in managing system resources more efficiently. By limiting the number of concurrent goroutines, you prevent the system from being overwhelmed, which can lead to better performance and stability.
- Predictable Behavior: With a fixed number of goroutines, the behavior of the program becomes more predictable. This is particularly important in production environments where consistent performance is crucial.
- Efficient Reuse: Goroutine pools allow for the efficient reuse of goroutines. Instead of creating and destroying goroutines for each task, the same goroutines are reused, reducing the overhead associated with goroutine creation and termination.
- Scalability: Goroutine pools can be easily scaled by adjusting the size of the pool. This allows you to fine-tune the level of concurrency based on the specific needs of your application.
- Simplified Error Handling: With a fixed number of goroutines, it becomes easier to manage and handle errors. You can implement centralized error handling mechanisms within the pool, making it easier to debug and maintain the application.
How do you implement a goroutine pool to control the number of simultaneous goroutines?
Implementing a goroutine pool in Go involves creating a structure to manage the pool and its workers. Here's a basic example of how you can implement a goroutine pool:
package main import ( "fmt" "sync" ) type Task func() type Pool struct { workers int taskQueue chan Task wg sync.WaitGroup } func NewPool(workers int) *Pool { p := Pool{ workers: workers, taskQueue: make(chan Task), } return p } func (p *Pool) Run() { for i := 0; i < p.workers; i { go func() { for task := range p.taskQueue { task() p.wg.Done() } }() } } func (p *Pool) Submit(task Task) { p.wg.Add(1) p.taskQueue <- task } func (p *Pool) Shutdown() { close(p.taskQueue) p.wg.Wait() } func main() { pool := NewPool(5) // Create a pool with 5 workers pool.Run() for i := 0; i < 10; i { task := func() { fmt.Printf("Task %d executed\n", i) } pool.Submit(task) } pool.Shutdown() }
In this example:
-
Pool Structure: The
Pool
struct contains the number of workers, a channel for the task queue, and async.WaitGroup
to manage the completion of tasks. - NewPool Function: This function initializes a new pool with the specified number of workers.
- Run Method: This method starts the worker goroutines. Each worker continuously pulls tasks from the task queue and executes them.
-
Submit Method: This method adds a new task to the task queue and increments the
WaitGroup
counter. -
Shutdown Method: This method closes the task queue and waits for all tasks to complete using the
WaitGroup
.
By using this implementation, you can control the number of simultaneous goroutines to the specified number of workers.
What are the potential drawbacks of using goroutine pools to manage concurrency?
While goroutine pools offer several benefits, there are also potential drawbacks to consider:
- Complexity: Implementing and managing a goroutine pool can add complexity to your code. You need to handle the creation of the pool, task submission, and proper shutdown, which can be error-prone.
- Overhead: There is some overhead associated with managing a goroutine pool, such as maintaining the task queue and coordinating the workers. This overhead might not be justified for simple applications with low concurrency needs.
- Fixed Concurrency: By using a fixed number of workers, you might not be able to take full advantage of the available system resources. If the system has more capacity, a fixed pool size might limit the overall performance.
- Potential Bottlenecks: If the task queue grows too large, it can become a bottleneck. Tasks might wait in the queue for a long time before being executed, leading to increased latency.
- Difficulty in Scaling: While you can adjust the size of the pool, doing so dynamically based on the current load can be challenging. This might require additional logic to monitor and adjust the pool size, adding further complexity.
In summary, while goroutine pools are a powerful tool for managing concurrency in Go, they come with trade-offs that need to be carefully considered based on the specific requirements of your application.
The above is the detailed content of How can you use goroutine pools to limit the number of concurrent goroutines?. For more information, please follow other related articles on the PHP Chinese website!
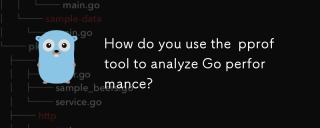
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
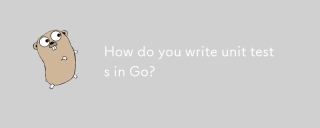
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
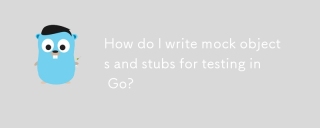
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
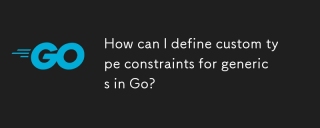
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
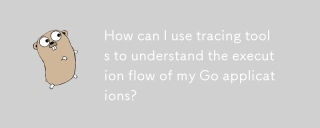
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
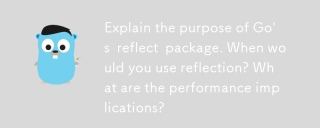
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
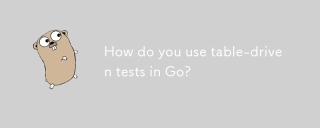
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
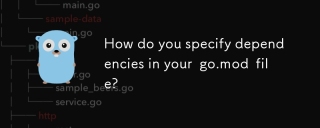
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
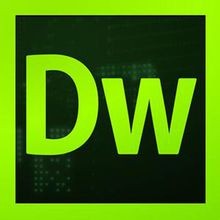
Dreamweaver CS6
Visual web development tools
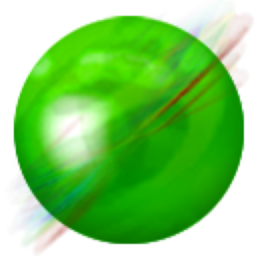
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
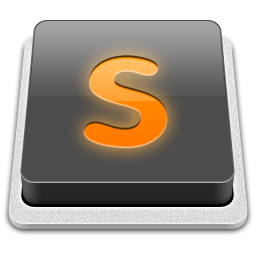
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
