What is the iota keyword in Go? How is it used for defining constants?
The iota
keyword in Go is a special constant generator that is used within const
blocks to create a series of related numerical constants. Its primary function is to provide an incrementing counter that can be used to assign values to constants in a succinct and readable way.
Here’s how iota
works:
-
iota
resets to 0 at the start of eachconst
block. - Each time
iota
is used within aconst
block, its value increments by 1. - If
iota
is not used in a line, it remains at the same value it was at for the previous line.
Here is an example to illustrate its usage:
const ( a = iota // a = 0 b // b = 1 c // c = 2 d = iota // d = 3 e // e = 4 )
In this example, iota
starts at 0 and increments with each new constant declaration within the block. The explicit use of iota
at d
resets its consideration, but the value continues incrementing from the previous line.
What are some practical examples of using iota in Go for constant declarations?
Using iota
, you can define sets of related constants efficiently. Here are some practical examples:
-
Enumerated Types:
You can define an enumeration of states or statuses:
type Status int const ( Pending Status = iota Approved Rejected )
Here,
Pending
would be 0,Approved
would be 1, andRejected
would be 2. -
Bit Flags:
iota
can be used to define bit flags, which are powers of 2:type BitFlag int const ( Read BitFlag = 1 << iota Write Execute )
In this example,
Read
would be 1 (2^0),Write
would be 2 (2^1), andExecute
would be 4 (2^2). -
Week Days:
Define days of the week:
type Weekday int const ( Sunday Weekday = iota Monday Tuesday Wednesday Thursday Friday Saturday )
This makes
Sunday
0,Monday
1, and so on up toSaturday
which is 6.
How does iota facilitate the creation of enumerated constants in Go?
iota
facilitates the creation of enumerated constants by providing a simple and efficient way to generate sequential values. This is particularly useful for creating enumerations, which are sets of named constants that represent a distinct set of values.
Here’s how iota
aids in creating enumerated constants:
-
Simplicity: It automatically increments its value within a
const
block, allowing you to assign sequential numbers to constants with minimal code. -
Readability: Code that uses
iota
for enumerations is easy to read and maintain because the intention of the constants is clear and the logic is concise. -
Flexibility:
iota
can be combined with expressions to define more complex sequences, such as bit flags or scaled values.
For example, to create an enumeration of directions:
type Direction int const ( North Direction = iota East South West )
In this case, North
is assigned 0, East
is assigned 1, South
is assigned 2, and West
is assigned 3, all through the use of iota
.
What are the benefits of using iota for defining a series of related constants in Go?
Using iota
for defining a series of related constants in Go provides several benefits:
-
Conciseness:
iota
allows you to write less code while still defining multiple constants. Instead of manually setting each constant’s value,iota
does it automatically. - Readability: The code becomes cleaner and more readable because the intention and relationships between constants are clearly expressed. This makes it easier for other developers to understand and maintain the code.
-
Error Reduction: By automating the assignment of values,
iota
reduces the chance of making errors such as typos or miscalculations in sequential numbers. -
Flexibility:
iota
can be used in expressions, allowing for the creation of more complex sequences of constants, such as bit flags or scaled values. -
Maintainability: If you need to add or remove constants from the sequence,
iota
automatically adjusts the values, reducing the effort needed to update the code.
Overall, iota
is a powerful feature in Go that greatly enhances the process of defining and managing sequences of related constants.
The above is the detailed content of What is the iota keyword in Go? How is it used for defining constants?. For more information, please follow other related articles on the PHP Chinese website!
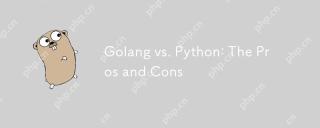
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
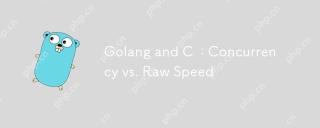
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
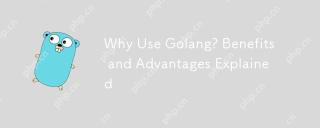
Reasons for choosing Golang include: 1) high concurrency performance, 2) static type system, 3) garbage collection mechanism, 4) rich standard libraries and ecosystems, which make it an ideal choice for developing efficient and reliable software.
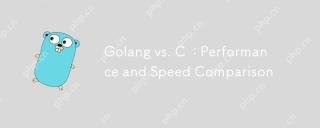
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
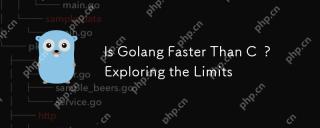
Golang performs better in compilation time and concurrent processing, while C has more advantages in running speed and memory management. 1.Golang has fast compilation speed and is suitable for rapid development. 2.C runs fast and is suitable for performance-critical applications. 3. Golang is simple and efficient in concurrent processing, suitable for concurrent programming. 4.C Manual memory management provides higher performance, but increases development complexity.
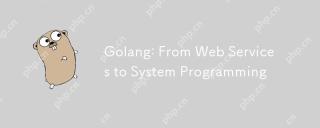
Golang's application in web services and system programming is mainly reflected in its simplicity, efficiency and concurrency. 1) In web services, Golang supports the creation of high-performance web applications and APIs through powerful HTTP libraries and concurrent processing capabilities. 2) In system programming, Golang uses features close to hardware and compatibility with C language to be suitable for operating system development and embedded systems.
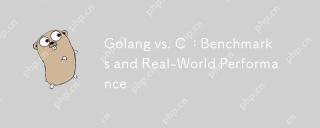
Golang and C have their own advantages and disadvantages in performance comparison: 1. Golang is suitable for high concurrency and rapid development, but garbage collection may affect performance; 2.C provides higher performance and hardware control, but has high development complexity. When making a choice, you need to consider project requirements and team skills in a comprehensive way.
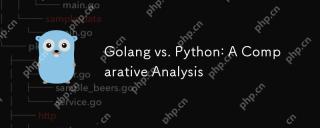
Golang is suitable for high-performance and concurrent programming scenarios, while Python is suitable for rapid development and data processing. 1.Golang emphasizes simplicity and efficiency, and is suitable for back-end services and microservices. 2. Python is known for its concise syntax and rich libraries, suitable for data science and machine learning.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
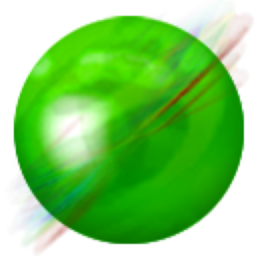
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
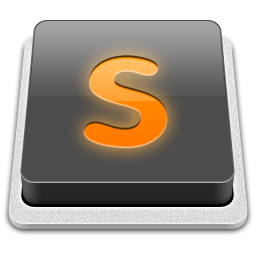
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
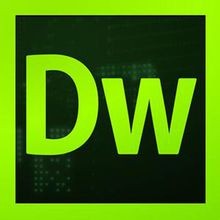
Dreamweaver CS6
Visual web development tools