What are the different ways to document Python code?
Documenting Python code is an essential practice for improving code readability, maintainability, and collaboration among developers. There are several effective ways to document Python code:
-
Inline Comments: These are brief notes placed directly within the code, intended to explain specific lines or blocks of code. Inline comments should be used sparingly and should clarify complex or non-obvious parts of the code. In Python, inline comments start with the
#
symbol. -
Docstrings: Docstrings are string literals that occur as the first statement in a function, class, or module. They provide a convenient way to associate documentation with Python objects. Docstrings are accessed by the
__doc__
attribute and can be used to generate documentation automatically. There are various formats for docstrings, including Google style, NumPy style, and reStructuredText. - External Documentation: For large projects or APIs, external documentation may be necessary. This can include README files, user manuals, and API reference guides. External documentation is typically written in markdown or reStructuredText and is often hosted on platforms like GitHub or Read the Docs.
- Type Hints: Although not traditional documentation, type hints can provide valuable information about expected data types and improve code clarity. Type hints are part of Python's type system and can be used in conjunction with tools like mypy for static type checking.
- README Files: A README file at the root of your project repository provides a high-level overview of the project, including installation instructions, usage examples, and sometimes even a quick start guide. It's typically the first point of contact for new users or contributors.
- Changelog: A changelog is a file that documents the changes, new features, bug fixes, and other updates made to the project over time. It is crucial for users and developers to understand the evolution of the project.
Each of these methods can be used individually or in combination to create comprehensive and effective documentation for Python projects.
How can I effectively use docstrings in Python?
Using docstrings effectively in Python involves following a consistent format and including all relevant information that would help users understand and use your code. Here's how to use docstrings effectively:
-
Choose a Docstring Format: Decide on a format for your docstrings. Common formats include:
- Google Style: Provides a clean, readable format with clear sections for parameters, returns, and raises.
- NumPy Style: Similar to Google style but often used in scientific computing, with additional sections for attributes and methods.
- reStructuredText: A more flexible format that can be used to generate rich documentation and is compatible with Sphinx.
-
Include Essential Information: A good docstring should include:
- A Brief Description: A one-line summary of what the function or class does.
- Parameters: A list of parameters, their types, and a brief description of each.
- Returns: Description of the return value and its type.
- Raises: Any exceptions that may be raised by the function.
- Examples: Usage examples, if applicable, can be very helpful.
-
Use Triple Quotes: Docstrings should be enclosed in triple quotes (
"""
) to allow for multi-line descriptions. - Place Docstrings Correctly: The docstring should be the first statement in a function, class, or module.
- Keep It Concise and Clear: While docstrings should be comprehensive, they should also be concise and avoid unnecessary verbosity.
Here's an example of a well-structured docstring using the Google style:
def calculate_area(length: float, width: float) -> float: """ Calculate the area of a rectangle. Args: length (float): The length of the rectangle. width (float): The width of the rectangle. Returns: float: The area of the rectangle. Raises: ValueError: If length or width is negative. Examples: >>> calculate_area(5, 3) 15.0 """ if length < 0 or width < 0: raise ValueError("Length and width must be non-negative.") return length * width
By following these guidelines, you can create docstrings that are informative, easy to read, and useful for both developers and automated documentation tools.
What tools are available for automatically generating Python code documentation?
Several tools are available for automatically generating Python code documentation, making it easier to maintain up-to-date and comprehensive documentation. Here are some of the most popular tools:
- Sphinx: Sphinx is one of the most widely used documentation generators for Python. It supports multiple output formats, including HTML, LaTeX, ePub, and more. Sphinx can parse reStructuredText docstrings and generate professional-looking documentation. It is often used in conjunction with Read the Docs for hosting.
- Pydoc: Pydoc is a standard tool included with Python that can generate documentation from docstrings. It can create HTML pages or run a local web server to display the documentation. Pydoc is simple to use but less feature-rich compared to Sphinx.
- Pycco: Inspired by Docco, Pycco is a lightweight documentation generator that produces HTML documentation with source code and inline comments. It's particularly useful for smaller projects or for developers who prefer a minimalistic approach.
- Doxygen: Although primarily used for C and other languages, Doxygen can also be used to document Python code. It supports multiple output formats and can generate diagrams and graphs.
- MkDocs: MkDocs is another popular tool for creating project documentation. It uses Markdown files and can be easily integrated with version control systems. MkDocs is particularly useful for creating user guides and project overviews.
- Read the Docs: While not a documentation generator itself, Read the Docs is a platform that can host documentation generated by tools like Sphinx or MkDocs. It integrates well with version control systems and can automatically build and publish documentation when changes are pushed to the repository.
Each of these tools has its strengths and is suited to different types of projects and documentation needs. Choosing the right tool depends on the size of your project, the desired output format, and the level of customization you need.
What are the best practices for maintaining up-to-date documentation in Python projects?
Maintaining up-to-date documentation is crucial for the success of any Python project. Here are some best practices to ensure your documentation remains current and useful:
- Integrate Documentation into the Development Process: Make documentation a part of your development workflow. Encourage developers to update documentation as they make changes to the code. This can be facilitated by including documentation tasks in pull requests and code reviews.
- Use Version Control: Store your documentation in the same version control system as your code. This ensures that documentation changes are tracked alongside code changes, making it easier to maintain consistency.
- Automate Documentation Generation: Use tools like Sphinx or Pydoc to automatically generate documentation from your code's docstrings. This reduces the manual effort required to keep documentation up-to-date and ensures that documentation reflects the current state of the code.
- Regularly Review and Update Documentation: Schedule regular reviews of your documentation to ensure it remains accurate and relevant. This can be part of your project's sprint planning or release cycle.
- Use Clear and Consistent Formatting: Adopt a consistent style for your documentation, whether it's Google style, NumPy style, or another format. Consistency makes documentation easier to read and maintain.
- Include Examples and Tutorials: Practical examples and tutorials can greatly enhance the usefulness of your documentation. They help users understand how to use your code in real-world scenarios.
- Document Breaking Changes: When making significant changes to your code, ensure that the documentation reflects these changes. Clearly document any breaking changes and provide migration guides if necessary.
- Leverage Continuous Integration (CI): Use CI tools to automatically build and test your documentation. This can help catch issues early and ensure that documentation is always up-to-date with the latest code changes.
- Encourage Community Contributions: If your project is open-source, encourage contributions to the documentation from the community. Provide clear guidelines on how to contribute and review documentation submissions carefully.
- Use Documentation as a Living Document: Treat your documentation as a living document that evolves with your project. Regularly solicit feedback from users and developers to identify areas for improvement.
By following these best practices, you can ensure that your Python project's documentation remains accurate, comprehensive, and helpful to users and developers alike.
The above is the detailed content of What are the different ways to document Python code?. For more information, please follow other related articles on the PHP Chinese website!
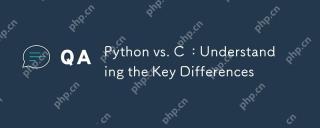
Python and C each have their own advantages, and the choice should be based on project requirements. 1) Python is suitable for rapid development and data processing due to its concise syntax and dynamic typing. 2)C is suitable for high performance and system programming due to its static typing and manual memory management.
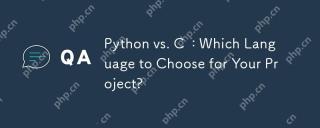
Choosing Python or C depends on project requirements: 1) If you need rapid development, data processing and prototype design, choose Python; 2) If you need high performance, low latency and close hardware control, choose C.
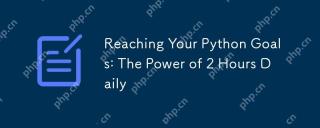
By investing 2 hours of Python learning every day, you can effectively improve your programming skills. 1. Learn new knowledge: read documents or watch tutorials. 2. Practice: Write code and complete exercises. 3. Review: Consolidate the content you have learned. 4. Project practice: Apply what you have learned in actual projects. Such a structured learning plan can help you systematically master Python and achieve career goals.
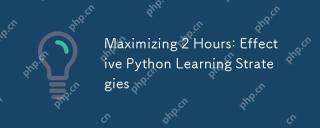
Methods to learn Python efficiently within two hours include: 1. Review the basic knowledge and ensure that you are familiar with Python installation and basic syntax; 2. Understand the core concepts of Python, such as variables, lists, functions, etc.; 3. Master basic and advanced usage by using examples; 4. Learn common errors and debugging techniques; 5. Apply performance optimization and best practices, such as using list comprehensions and following the PEP8 style guide.

Python is suitable for beginners and data science, and C is suitable for system programming and game development. 1. Python is simple and easy to use, suitable for data science and web development. 2.C provides high performance and control, suitable for game development and system programming. The choice should be based on project needs and personal interests.
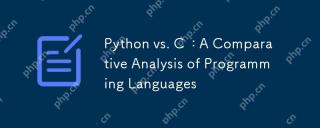
Python is more suitable for data science and rapid development, while C is more suitable for high performance and system programming. 1. Python syntax is concise and easy to learn, suitable for data processing and scientific computing. 2.C has complex syntax but excellent performance and is often used in game development and system programming.
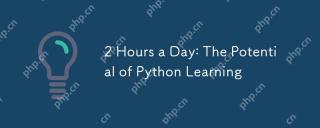
It is feasible to invest two hours a day to learn Python. 1. Learn new knowledge: Learn new concepts in one hour, such as lists and dictionaries. 2. Practice and exercises: Use one hour to perform programming exercises, such as writing small programs. Through reasonable planning and perseverance, you can master the core concepts of Python in a short time.
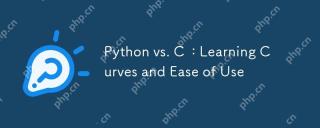
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
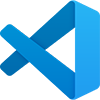
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
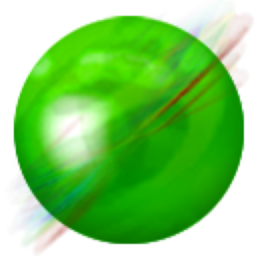
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment