What are lambda functions in Python? When are they useful?
Lambda functions in Python, also known as anonymous functions, are small, inline functions that you can define without giving them a name. They are defined using the lambda
keyword, followed by a set of parameters, a colon, and an expression. The syntax for a lambda function is as follows:
lambda arguments: expression
Here's an example of a simple lambda function:
add = lambda x, y: x y print(add(5, 3)) # Output: 8
Lambda functions are useful in several scenarios:
- Short, Simple Functions: When you need a small function for a short period, lambda functions can be very handy. They can be defined right where they are needed, reducing the need to clutter your code with many small function definitions.
-
Functional Programming: Lambda functions are particularly useful in functional programming paradigms. They can be passed as arguments to higher-order functions like
map()
,filter()
, andreduce()
. - Reducing Code Complexity: When used judiciously, lambda functions can make your code more concise and readable by avoiding unnecessary function definitions.
- Sorting and Key Functions: Lambda functions are often used as key functions in sorting operations, where you need to define a custom sorting logic on the fly.
How can lambda functions improve the readability of your Python code?
Lambda functions can improve the readability of your Python code in several ways:
-
Conciseness: By allowing you to define small functions inline, lambda functions can reduce the overall length of your code. This can make it easier to understand the flow of the program without having to jump to a separate function definition.
For example, instead of defining a separate function to square a number:
def square(x): return x * x numbers = [1, 2, 3, 4, 5] squared_numbers = list(map(square, numbers))
You can use a lambda function:
numbers = [1, 2, 3, 4, 5] squared_numbers = list(map(lambda x: x * x, numbers))
The lambda version is more concise and keeps the logic together.
-
Clarity in Functional Operations: When using built-in functions like
map()
,filter()
, andreduce()
, lambda functions can make it clear what operation is being applied to the data without needing to look elsewhere in the code. - Avoiding Unnecessary Names: Lambda functions help avoid cluttering the namespace with single-use function names, which can improve the overall clarity of your code.
In what specific scenarios would you prefer using a lambda function over a regular function in Python?
You would prefer using a lambda function over a regular function in the following specific scenarios:
-
Inline Operations: When you need to perform a simple operation within a larger expression, lambda functions are ideal. For example, sorting a list of tuples based on the second element:
students = [('Alice', 88), ('Bob', 92), ('Charlie', 75)] sorted_students = sorted(students, key=lambda student: student[1])
-
Callbacks and Event Handlers: In graphical user interface (GUI) programming or web development, lambda functions can be used as short-lived callbacks or event handlers.
import tkinter as tk root = tk.Tk() button = tk.Button(root, text="Click Me", command=lambda: print("Button clicked!")) button.pack() root.mainloop()
-
Data Processing with Built-in Functions: When working with functions like
map()
,filter()
, orreduce()
, lambda functions allow you to specify the transformation or filtering logic inline.numbers = [1, 2, 3, 4, 5] even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
- When Function Definition Would Be Overkill: If you need a function for just one or two lines of code, defining a full function might be unnecessary. Lambda functions provide a more lightweight solution.
Can lambda functions be used effectively with Python's built-in functions like map(), filter(), and reduce()?
Yes, lambda functions can be used very effectively with Python's built-in functions like map()
, filter()
, and reduce()
. Here are some examples of how they work together:
-
map(): The
map()
function applies a given function to each item of an iterable and returns a map object. Lambda functions are often used to define the function inline.numbers = [1, 2, 3, 4, 5] squared_numbers = list(map(lambda x: x * x, numbers)) print(squared_numbers) # Output: [1, 4, 9, 16, 25]
-
filter(): The
filter()
function constructs an iterator from elements of an iterable for which a function returns true. Lambda functions are commonly used to define the filtering criteria.numbers = [1, 2, 3, 4, 5] even_numbers = list(filter(lambda x: x % 2 == 0, numbers)) print(even_numbers) # Output: [2, 4]
-
reduce(): The
reduce()
function, which is part of thefunctools
module, applies a rolling computation to sequential pairs of values in a list. Lambda functions can be used to specify the computation.from functools import reduce numbers = [1, 2, 3, 4, 5] sum_of_numbers = reduce(lambda x, y: x y, numbers) print(sum_of_numbers) # Output: 15
These examples illustrate how lambda functions can be used to provide concise and clear implementations of operations that involve applying a function to a sequence of data.
The above is the detailed content of What are lambda functions in Python? When are they useful?. For more information, please follow other related articles on the PHP Chinese website!
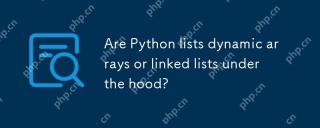
Pythonlistsareimplementedasdynamicarrays,notlinkedlists.1)Theyarestoredincontiguousmemoryblocks,whichmayrequirereallocationwhenappendingitems,impactingperformance.2)Linkedlistswouldofferefficientinsertions/deletionsbutslowerindexedaccess,leadingPytho
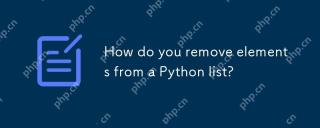
Pythonoffersfourmainmethodstoremoveelementsfromalist:1)remove(value)removesthefirstoccurrenceofavalue,2)pop(index)removesandreturnsanelementataspecifiedindex,3)delstatementremoveselementsbyindexorslice,and4)clear()removesallitemsfromthelist.Eachmetho
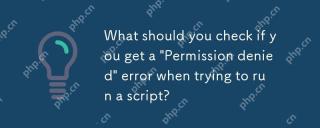
Toresolvea"Permissiondenied"errorwhenrunningascript,followthesesteps:1)Checkandadjustthescript'spermissionsusingchmod xmyscript.shtomakeitexecutable.2)Ensurethescriptislocatedinadirectorywhereyouhavewritepermissions,suchasyourhomedirectory.
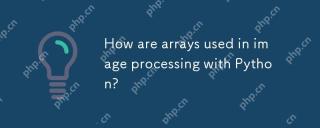
ArraysarecrucialinPythonimageprocessingastheyenableefficientmanipulationandanalysisofimagedata.1)ImagesareconvertedtoNumPyarrays,withgrayscaleimagesas2Darraysandcolorimagesas3Darrays.2)Arraysallowforvectorizedoperations,enablingfastadjustmentslikebri
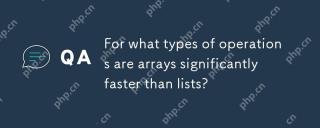
Arraysaresignificantlyfasterthanlistsforoperationsbenefitingfromdirectmemoryaccessandfixed-sizestructures.1)Accessingelements:Arraysprovideconstant-timeaccessduetocontiguousmemorystorage.2)Iteration:Arraysleveragecachelocalityforfasteriteration.3)Mem
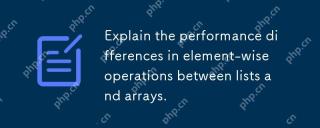
Arraysarebetterforelement-wiseoperationsduetofasteraccessandoptimizedimplementations.1)Arrayshavecontiguousmemoryfordirectaccess,enhancingperformance.2)Listsareflexiblebutslowerduetopotentialdynamicresizing.3)Forlargedatasets,arrays,especiallywithlib
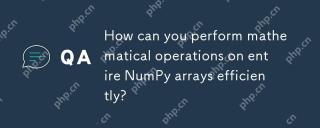
Mathematical operations of the entire array in NumPy can be efficiently implemented through vectorized operations. 1) Use simple operators such as addition (arr 2) to perform operations on arrays. 2) NumPy uses the underlying C language library, which improves the computing speed. 3) You can perform complex operations such as multiplication, division, and exponents. 4) Pay attention to broadcast operations to ensure that the array shape is compatible. 5) Using NumPy functions such as np.sum() can significantly improve performance.
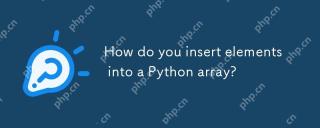
In Python, there are two main methods for inserting elements into a list: 1) Using the insert(index, value) method, you can insert elements at the specified index, but inserting at the beginning of a large list is inefficient; 2) Using the append(value) method, add elements at the end of the list, which is highly efficient. For large lists, it is recommended to use append() or consider using deque or NumPy arrays to optimize performance.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
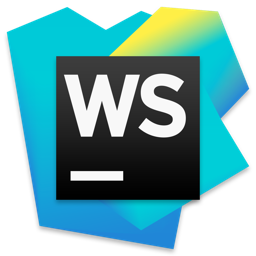
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor
