How can I use Swoole to build a message queue?
To build a message queue using Swoole, you can utilize its built-in components and features that support high-performance asynchronous programming. Here’s a step-by-step guide on how to set up a basic message queue using Swoole:
- Install Swoole: First, ensure that Swoole is installed in your development environment. You can install it via PECL or by downloading the source code and compiling it manually.
-
Create the Message Queue: Swoole does not come with a built-in message queue system, but you can create one using Swoole's asynchronous I/O capabilities and coroutines. A common approach is to use a Swoole server with a memory-based queue such as
SplQueue
or a more robust solution like Redis as the storage mechanism. -
Set Up a Swoole Server: Start by creating a Swoole server to handle the connections and queue operations. Below is a basic example of a Swoole server for a message queue:
$server = new Swoole\Server("0.0.0.0", 9501); $server->on('Start', function ($server) { echo "Swoole message queue server is started at http://127.0.0.1:9501\n"; }); $server->on('Connect', function ($server, $fd) { echo "Client: Connect.\n"; }); $queue = new SplQueue(); $server->on('Receive', function ($server, $fd, $reactor_id, $data) use ($queue) { $data = trim($data); $command = explode(' ', $data); switch ($command[0]) { case 'push': $queue->push($command[1]); $server->send($fd, "Message pushed to queue\n"); break; case 'pop': if (!$queue->isEmpty()) { $message = $queue->pop(); $server->send($fd, "Message popped: " . $message . "\n"); } else { $server->send($fd, "Queue is empty\n"); } break; default: $server->send($fd, "Invalid command\n"); } }); $server->on('Close', function ($server, $fd) { echo "Client: Close.\n"; }); $server->start();
- Client Implementation: Develop a client application that can send commands to the server to push messages onto the queue or pop them off the queue. The client can be written in PHP or any other language that can communicate over TCP/IP.
- Testing: Test the server by running it and using a client to push and pop messages from the queue, ensuring it works as expected.
This setup provides a simple, in-memory message queue using Swoole's asynchronous capabilities. For production environments, you might want to integrate with more robust storage solutions like Redis or RabbitMQ, still leveraging Swoole for its performance advantages.
What are the performance benefits of using Swoole for message queue implementations?
Swoole offers several performance benefits for message queue implementations, which include:
- Asynchronous I/O: Swoole supports non-blocking I/O operations, which means that while waiting for I/O operations like network requests or database queries, other tasks can be processed, improving overall system throughput.
- Coroutines: Swoole's coroutines provide a lightweight method of handling multiple concurrent operations within a single thread. This significantly reduces the overhead associated with traditional threading models, allowing for efficient handling of numerous queue operations.
- Low Latency: With its event-driven architecture, Swoole can handle high-frequency messages with low latency, which is crucial for real-time messaging applications.
- Memory Efficiency: Swoole's use of coroutines and its event-loop model minimizes memory usage, allowing more operations to be handled with less system resource consumption.
- Scalability: Swoole servers are designed to scale horizontally, making it easier to manage increased loads by adding more server instances.
- Integrated Features: Swoole provides built-in features like timers and task workers that can be used to manage message timeouts or offload heavy processing tasks, further optimizing the performance of your message queue.
These benefits make Swoole an attractive option for building high-performance message queue systems that need to handle a large volume of messages with minimal delay.
How can I integrate Swoole's message queue with existing applications?
Integrating Swoole's message queue with existing applications can be achieved through several approaches:
- API Integration: Develop an API layer that acts as an interface between the existing application and the Swoole message queue. The application can send HTTP requests to this API to push or pop messages from the queue.
- Direct TCP/UDP Communication: If the existing application supports TCP or UDP protocols, it can communicate directly with the Swoole server by sending commands to push or pop messages.
- Database Integration: If the existing application interacts with a database, you can use a shared database as an intermediary. The Swoole server can monitor the database for new messages, and the existing application can insert messages into the database for processing by the Swoole server.
- Message Bus Pattern: Use a message bus or event-driven architecture where the existing application can publish messages to the bus, and the Swoole server can subscribe to these messages for processing.
Here’s a simple example of how an existing application might use HTTP requests to interact with a Swoole message queue:
// Existing application code $client = new \GuzzleHttp\Client(); $response = $client->post('http://127.0.0.1:9501', [ 'body' => 'push Hello, World!' ]); if ($response->getStatusCode() == 200) { echo $response->getBody(); }
In this example, the existing application uses an HTTP client to send a push command to the Swoole server.
What are the best practices for maintaining and scaling a message queue built with Swoole?
To maintain and scale a message queue built with Swoole, consider the following best practices:
- Monitoring and Logging: Implement comprehensive monitoring and logging to track queue performance, message throughput, and errors. Use tools like Prometheus or Grafana to monitor the system health.
- Load Balancing: Use load balancers to distribute incoming traffic across multiple Swoole server instances, ensuring no single point of failure and efficient handling of high volumes of messages.
- Scaling Horizontally: As demand grows, scale horizontally by adding more Swoole server instances. This can be managed through containerization tools like Docker and orchestration platforms like Kubernetes.
- Persistence: Ensure messages are persisted in a reliable storage system like Redis or a dedicated message broker like RabbitMQ to prevent data loss in case of server failures.
- Message Acknowledgment: Implement a message acknowledgment system to ensure messages are processed correctly. If a message is not acknowledged within a certain time, it should be re-queued for processing.
- Error Handling and Retry Logic: Design robust error handling and retry logic to manage failures gracefully. Implement backoff strategies to prevent overwhelming the system with retries.
- Queue Partitioning: For very high-volume systems, partition the queue into multiple smaller queues based on message type or other criteria to enhance performance and scalability.
- Regular Maintenance: Schedule regular maintenance tasks like clearing out old messages, updating software, and optimizing configurations to keep the system running smoothly.
- Security Measures: Implement security measures to protect the message queue from unauthorized access and potential attacks. Use SSL/TLS for encrypted communication and consider implementing authentication mechanisms.
By following these best practices, you can ensure that your Swoole-based message queue remains efficient, reliable, and scalable as your application grows.
The above is the detailed content of How can I use Swoole to build a message queue?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
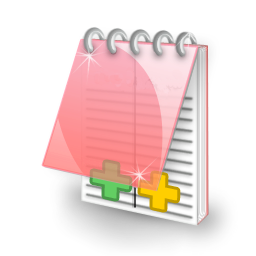
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
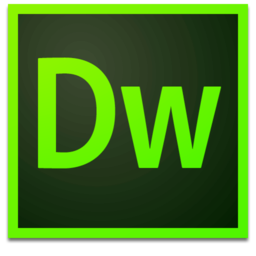
Dreamweaver Mac version
Visual web development tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
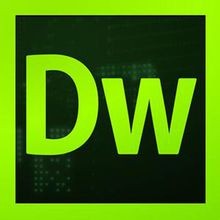
Dreamweaver CS6
Visual web development tools
