Handling dates and time is one of the problems that make programmers headaches. At the same time, they are the foundation of software development, from metadata and thing sorting to time-based triggers, and a large number of applications in between.
Date and time are also prone to errors. Improper handling, they can confuse end users and programmer colleagues.
Here is a quick guide on how to handle dates and times in PHP programming language. It is designed as a reference for your most common needs such as date formatting and adjustment. It's simple, but it's likely to cover 80% of your needs.
Table of contents
- Get the current date and time
- Constructing a DateTime object for a specific time
- Time zone
- Localization
- Time Travel
- Date and time that occurs regularly
- How many days ago?
- Next steps?
This study was supported by Frontend Masters, the official learning partner of CSS-Tricks.
Need front-end development training?
Frontend Masters is the best place to learn. They offer courses on all the most important front-end technologies. Interested in becoming a full stack developer? This is your best choice:
Take the course#### Get the current date and time
It should be noted that dates and time can be represented in three forms: timestamps (i.e. epoch time), DateTime object and string.
First, get the code for the current date and time:
<?php $now = new DateTime(); var_dump($now); // object(DateTime)#1 (3) { // ["date"]=?> // string(26) "2021-10-13 22:25:11.790490" // ["timezone_type"]=> // int(3) // ["timezone"]=> // string(12) "Asia/Jakarta" // }
This provides a DateTime object that can be used to create date and time strings:
<?php $now = new DateTime(); echo $now-?>format("Ymd"); // 2021-10-13 echo $now->format("Ymd h:i:s A"); // 2021-10-13 10:10:31 PM
You can intuitively understand that Y represents the year, m represents the month, d represents the date in the month, and so on. The complete parameter list can be found in the PHP manual, but I will list some of the most commonly used parameters here for reference.
The DateTime object can be converted to a timestamp:
<?php $now = new DateTime(); echo $now-?>getTimestamp(); // 1634139081
But we can also get the current timestamp without constructing the DateTime object:
<?php echo time(); // 1634139081</pre?><h3 id="Constructing-a-DateTime-object-for-a-specific-time"> Constructing a DateTime object for a specific time</h3> <p>What if we want to construct a DateTime object for a specific time (for example, July 14, 2011)? We can pass the formatted date string to the constructor:</p> <?php $date = new DateTime("2011-07-14"); var_dump($date); // object(DateTime)#1 (3) { // ["date"]=?> // string(26) "2011-07-14 00:00:00.000000" // ["timezone_type"]=> // int(3) // ["timezone"]=> // string(12) "Asia/Jakarta" // } <p>The constructor also accepts other formats:</p> <?php $date = new DateTime("14-07-2011"); var_dump($date); // object(DateTime)#1 (3) { // ["date"]=?> // string(26) "2011-07-14 00:00:00.000000" // ["timezone_type"]=> // int(3) // ["timezone"]=> // string(12) "Asia/Jakarta" // } <p>But be aware of ambiguous formats, such as:</p> <?php $date = new DateTime("07/14/2011"); var_dump($date); // object(DateTime)#1 (3) { // ["date"]=?> // string(26) "2011-07-14 00:00:00.000000" // ["timezone_type"]=> // int(3) // ["timezone"]=> // string(12) "Asia/Jakarta" // } <p>You might think everyone should be familiar with the date format in the United States. But not everyone is familiar with it, and it may be interpreted differently. PostgreSQL is no exception.</p> CREATE TABLE IF NOT EXISTS public.datetime_demo ( created_at date ); insert into datetime_demo (created_at) values ('07/12/2011'); select created_at from datetime_demo; /* 2011-12-07 */ <p>You might think this will return July 12, 2011, but it returns December 7, 2011. A better approach is to use an explicit format:</p> <?php $date = DateTime::createFromFormat('m/d/y', "10/08/21"); var_dump($date); //object(DateTime)#2 (3) { // ["date"]=?> // string(26) "2021-10-08 16:00:47.000000" // ["timezone_type"]=> // int(3) // ["timezone"]=> // string(12) "Asia/Jakarta" //} <p>What if we want to construct a DateTime object from a timestamp?</p> <?php $date = new DateTime(); $date-?>setTimestamp(1634142890); var_dump($date); //object(DateTime)#1 (3) { // ["date"]=> // string(26) "2021-10-13 23:34:50.000000" // ["timezone_type"]=> // int(3) // ["timezone"]=> // string(12) "Asia/Jakarta" // } <p>If we want to convert the timestamp object to a formatted date string, we don't have to create a DateTime object:</p> <?php echo date("Ymd h:i A", time()); // 2021-10-14 04:10 PM</pre?><h3 id="Time-zone"> Time zone</h3> <p>We can create a DateTime object with time zone information, for example, if we are dealing with Pacific Standard Time, Eastern Daylight Time, etc.</p> <?php $timezone = new DateTimeZone("America/New_York"); $date = new DateTime("2021-10-13 05:00", $timezone); var_dump($date); // object(DateTime)#1 (3) { // ["date"]=?> // string(26) "2021-10-13 05:00:00.000000" // ["timezone_type"]=> // int(3) // ["timezone"]=> // string(16) "America/New_York" // } // For example, Eastern Daylight Time: New York $date = new DateTime("2021-10-13 05:00 EDT"); var_dump($date); // object(DateTime)#2 (3) { // ["date"]=> // string(26) "2021-10-13 05:00:00.000000" // ["timezone_type"]=> // int(2) // ["timezone"]=> // string(3) "EDT" // } $date = new DateTime("2021-10-13 05:00 -04:00"); var_dump($date); // object(DateTime)#1 (3) { // ["date"]=> // string(26) "2021-10-13 05:00:00.000000" // ["timezone_type"]=> // int(1) // ["timezone"]=> // string(6) "-04:00" // } <p>There are three ways to create a DateTime object containing time zone information. timezone_type accepts different values for each method.</p> <p>But what if we want to convert dates and times displayed in the New York time zone to display in the Jakarta time zone?</p> <?php $newYorkTimeZone = new DateTimeZone("America/New_York"); $date = new DateTime("2021-11-11 05:00", $newYorkTimeZone); echo $date-?>format("Ymd h:i A"); // 2021-11-11 05:00 AM $jakartaTimeZone = new DateTimeZone("Asia/Jakarta"); $date->setTimeZone($jakartaTimeZone); echo $date->format("Ymd h:i A"); // 2021-11-11 05:00 PM <p>When New York is 5:00 a.m., Jakarta is 5:00 p.m. on the same day. On November 11, 2021, Jakarta is 12 hours faster than New York. But a month ago, Jakarta was only 11 hours faster than New York, as shown below:</p> <?php $newYorkTimeZone = new DateTimeZone("America/New_York"); $date = new DateTime("2021-10-11 05:00", $newYorkTimeZone); echo $date-?>format("Ymd h:i A"); // 2021-10-11 05:00 AM $jakartaTimeZone = new DateTimeZone("Asia/Jakarta"); $date->setTimeZone($jakartaTimeZone); echo $date->format("Ymd h:i A"); // 2021-10-11 04:00 PM <p>PHP automatically processes daylight saving time.</p> <h3 id="Localization">Localization</h3> <p>Here are the common ways to display dates and times in the United States:</p> <?php $now = new DateTime(); echo $now-?>format("m/d/Y h:i A"); // 10/14/2021 03:00 PM <p>But someone in France may prefer a more common format than their region. They may complain, “This is horrible.” First, no one except the United States puts the month before the date. Second, France does not use AM or PM – they use a 24-hour system like the military (e.g. 14:00 instead of 2:00 pm). This is what satisfies the French locals.</p> <?php $now = new DateTime(); echo $now-?>format("d/m/YH:i"); // 14/10/2021 15:00 <p>But this requires a deep understanding of a particular country or region. Instead, we can localize the date. To localize the date, we need to install internationalization support for PHP. In Ubuntu, we can perform this step:</p> $ sudo apt-get install php-intl <p>To display date and time in French, we can use IntlDateFormatter:</p> $locale = "fr_FR.UTF-8"; $formatter = new IntlDateFormatter($locale, IntlDateFormatter::FULL, IntlDateFormatter::SHORT, "Asia/Singapore"); $date = new DateTime("2020-10-10 00:00 UTC"); echo $formatter->format($date); // samedi 10 octobre 2020 à 08:00 <p>You pass the French locale as the first parameter of the IntlDateFormatter.<br> The second parameter is the format of the date. The third parameter is the format of time. The time zone that displays the date and time is in the fourth parameter.</p> <p>In addition to IntlDateFormatter::FULL and IntlDateFormatter::SHORT, other commonly used formats include IntlDateFormatter::NONE and IntlDateFormatter::LONG<br> and IntlDateFormatter::MEDIUM.</p> <p>If you use IntlDateFormatter::NONE for time or third parameter, you do not include the time in the format:</p> $locale = "fr_FR.UTF-8"; $formatter = new IntlDateFormatter($locale, IntlDateFormatter::LONG, IntlDateFormatter::NONE, "Asia/Singapore"); $date = new DateTime("2020-10-10 00:00 UTC"); echo $formatter->format($date); // 10 octobre 2020 <h3 id="Time-Travel">Time Travel</h3> <p>Let's travel time and go back to the past and the future. First, let's understand DateInterval:</p> <?php $interval = new DateInterval("P4M1W2DT2H5M"); // P 4M 1W 2D T 2H 5M // // P = Weekly interval (year, month, week, day) // 4M = 4 months // 1W = 1 week // 2D = 2 days // // T = Time interval (hours, minutes, seconds) // 2H = 2 hours // 5M = 5 minutes</pre?><p> P and T are used to separate the period interval and time interval. Here are how we go to the future:</p> <?php $date = new DateTime("2021-10-14"); $interval = new DateInterval("P2D"); // 2 days $futureDate = $date-?>add($interval); echo $futureDate->format("Ymd"); // 2021-10-16 <p>Here is how we can go back to the past:</p> <?php $date = new DateTime("2021-10-14 10:00"); $interval = new DateInterval("PT6H"); // 6 hours $pastDate = $date-?>sub($interval); echo $pastDate->format("Ymd H:i"); // 2021-10-14 04:00 <p>If we want to use the name of the day of the week for time travel, we can use the setTimestamp() method of the strtotime() function and the DateTime object:</p> <?php $nextTuesday = strtotime("next tutorial"); $date = new DateTime("2021-10-14"); $date-?>setTimestamp($nextTuesday); echo $date->format("Ymd"); // 2021-10-19 <p>See the complete list of strtotime() parameters in the PHP documentation.</p> <h3 id="Date-and-time-that-occurs-regularly">Date and time that occurs regularly</h3> <p>A common feature in a calendar application is setting up repeated reminders, such as two days or once a week. We can use DatePeriod to represent a period of time:</p> <?php $start = new DateTime("2021-10-01"); $end = new DateTime("2021-11-01"); $interval = new DateInterval("P1W"); // 1 week$range = new DatePeriod($start, $interval, $end); // Starting from October 1, 2021 (inclusive), skipped every 1 week // until November 1, 2021 (excluding) foreach ($range as $date) { echo $date-?>format("Ymd") . "n"; } // 2022-10-01 // 2022-10-08 // 2022-10-15 // 2022-10-22 // 2022-10-29 <h3 id="How-many-days-ago">How many days ago?</h3> <p>Did you know that a service like Twitter will show someone’s X minutes/hour/day/etc.? We can do this by calculating the elapsed time between the current time and the time when the operation occurs.</p> <?php $date = new DateTime("2022-10-30"); $date2 = new DateTime("2022-10-25"); $date3 = new DateTime("2022-10-10"); $date4 = new DateTime("2022-03-30"); $date5 = new DateTime("2020-03-30"); function get_period_ago($endDate, $startDate) { $dateInterval = $endDate-?>diff($startDate); if ($dateInterval->invert==1) { if ($dateInterval->y > 0) { return $dateInterval->y . " years ago"; } if ($dateInterval->m > 0) { return $dateInterval->m . " months ago"; } if ($dateInterval->d > 7) { return (int)($dateInterval->d / 7) . " weeks ago"; } if ($dateInterval->d > 0) { return $dateInterval->d . " days ago"; } } } echo get_period_ago($date, $date2); // 5 days ago echo get_period_ago($date, $date3); // 2 weeks ago echo get_period_ago($date, $date4); // 7 months ago echo get_period_ago($date, $date5); // 2 years ago <p>After getting the DateInterval object from the diff() method, make sure that the $startDate variable is in the past by checking the invert property. Then check the y, m, and d properties.</p> <p>A complete list of DateInterval object properties can be found in the PHP document here.</p> <h3 id="Next-steps">Next steps?</h3> <p>Now you have mastered some PHP code snippets that are commonly used when dealing with dates and times. Need to get the current date and time? Maybe you need to format the date somehow, or include the local time zone, or compare the dates. All of this is here!</p> <p>Of course, we haven't discussed more methods and functions about dates and times - such as calendar-related functions, etc. Be sure to check the date and time section of the PHP manual at any time for more use cases and examples.</p>
The above is the detailed content of PHP Date and Time Recipes. For more information, please follow other related articles on the PHP Chinese website!
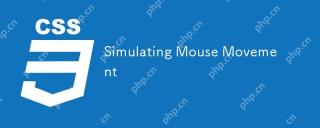
If you've ever had to display an interactive animation during a live talk or a class, then you may know that it's not always easy to interact with your slides
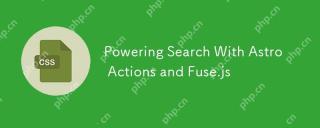
With Astro, we can generate most of our site during our build, but have a small bit of server-side code that can handle search functionality using something like Fuse.js. In this demo, we’ll use Fuse to search through a set of personal “bookmarks” th
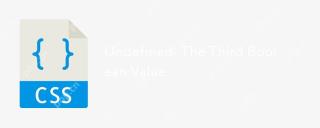
I wanted to implement a notification message in one of my projects, similar to what you’d see in Google Docs while a document is saving. In other words, a
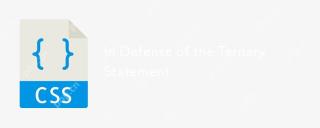
Some months ago I was on Hacker News (as one does) and I ran across a (now deleted) article about not using if statements. If you’re new to this idea (like I
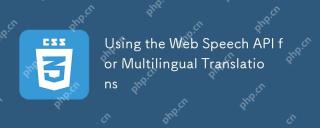
Since the early days of science fiction, we have fantasized about machines that talk to us. Today it is commonplace. Even so, the technology for making
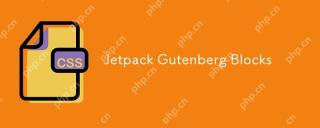
I remember when Gutenberg was released into core, because I was at WordCamp US that day. A number of months have gone by now, so I imagine more and more of us
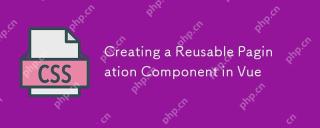
The idea behind most of web applications is to fetch data from the database and present it to the user in the best possible way. When we deal with data there
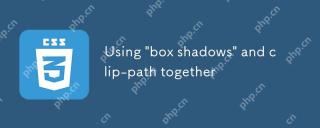
Let's do a little step-by-step of a situation where you can't quite do what seems to make sense, but you can still get it done with CSS trickery. In this


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
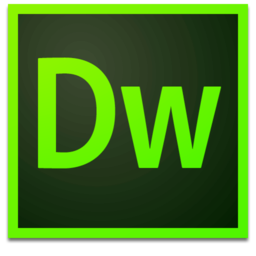
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
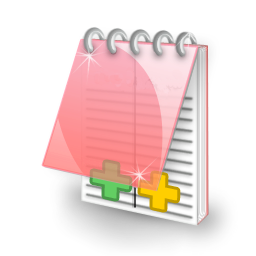
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function