


How do smart pointers (unique_ptr, shared_ptr, weak_ptr) work in C and when should I use them?
How do smart pointers (unique_ptr, shared_ptr, weak_ptr) work in C and when should I use them?
Smart pointers in C are essentially classes that act like pointers but automatically manage the memory they point to. They help prevent memory leaks and dangling pointers, common issues with raw pointers. They achieve this through RAII (Resource Acquisition Is Initialization): the resource (memory) is acquired when the smart pointer is created and automatically released when the smart pointer goes out of scope or is explicitly deleted.
There are three main types of smart pointers in C :
-
unique_ptr
: This represents exclusive ownership of a dynamically allocated object. Only oneunique_ptr
can point to a given object at any time. When theunique_ptr
goes out of scope, the object it points to is automatically deleted. You should useunique_ptr
when you need sole ownership of a resource and want to ensure it's automatically cleaned up. It's generally the preferred choice for most situations where you need automatic memory management.unique_ptr
doesn't allow copying, only moving. -
shared_ptr
: This allows shared ownership of a dynamically allocated object. Multipleshared_ptr
objects can point to the same object. An internal reference counter tracks the number ofshared_ptr
s pointing to the object. When the reference count drops to zero, the object is automatically deleted. Useshared_ptr
when multiple parts of your code need to access and manage the same object. -
weak_ptr
: This provides a non-owning reference to an object managed by ashared_ptr
. It doesn't increment the reference count. Aweak_ptr
can be used to check if the object still exists before attempting to access it, preventing potential dangling pointer issues. Useweak_ptr
when you need to observe the lifecycle of an object managed by ashared_ptr
without affecting its lifetime. You must use.lock()
to obtain ashared_ptr
from aweak_ptr
; this will return ashared_ptr
if the object still exists, or an emptyshared_ptr
otherwise.
When to use which:
- Use
unique_ptr
for single ownership scenarios, offering the best performance and simplicity. - Use
shared_ptr
when multiple owners are necessary, but be mindful of the overhead of the reference counting. - Use
weak_ptr
to safely observe objects managed byshared_ptr
without influencing their lifetime.
What are the key differences between unique_ptr
, shared_ptr
, and weak_ptr
in terms of ownership and memory management?
The core difference lies in their ownership semantics:
-
unique_ptr
: Exclusive ownership. Only oneunique_ptr
can point to a given object at a time. The object is deleted when theunique_ptr
is destroyed. No reference counting is involved. Ownership can be transferred usingstd::move
. -
shared_ptr
: Shared ownership. Multipleshared_ptr
s can point to the same object. An internal reference count tracks the number ofshared_ptr
s. The object is deleted when the reference count reaches zero. This introduces overhead compared tounique_ptr
. -
weak_ptr
: Non-owning reference. Aweak_ptr
doesn't affect the reference count of the object it refers to. It's used to check if the object still exists before attempting access. It provides a way to break circular dependencies betweenshared_ptr
s.
In terms of memory management, unique_ptr
offers the most straightforward approach, while shared_ptr
involves the overhead of maintaining a reference count. weak_ptr
doesn't directly manage memory but helps prevent dangling pointers in scenarios involving shared ownership.
How can I avoid memory leaks and dangling pointers when using smart pointers in C ?
Smart pointers significantly reduce the risk of memory leaks and dangling pointers, but careful usage is still crucial:
- Avoid raw pointers whenever possible: Prefer smart pointers for managing dynamically allocated memory.
-
Correct usage of
std::move
: When transferring ownership of aunique_ptr
, usestd::move
to avoid copying and ensure the originalunique_ptr
is properly reset. -
Careful cycle detection: Circular dependencies between
shared_ptr
s can lead to memory leaks. Useweak_ptr
to break these cycles. If object A has ashared_ptr
to object B, and object B has ashared_ptr
to object A, neither will ever be deleted. Using aweak_ptr
in one of these relationships breaks the cycle. - Exception safety: Ensure proper exception handling to prevent leaks in case of exceptions during object creation or manipulation. If an exception occurs before a smart pointer is created, no leak will happen; if an exception occurs after a smart pointer is created, the smart pointer's destructor will automatically clean up.
- Proper initialization: Always initialize smart pointers, avoiding null pointers unless explicitly intended.
What are the performance implications of using different types of smart pointers in a C application?
The performance of smart pointers varies depending on the type and usage:
-
unique_ptr
: Generally has the lowest overhead because it doesn't involve reference counting. It's the most performant option. -
shared_ptr
: Has higher overhead due to the atomic increment and decrement operations on the reference count. This overhead can become significant in performance-critical sections of code or with frequent modifications to shared ownership. -
weak_ptr
: Has relatively low overhead compared toshared_ptr
since it doesn't maintain a reference count. However, using.lock()
to access the managed object introduces a small performance cost.
In summary: unique_ptr
is the most efficient, followed by weak_ptr
, and shared_ptr
having the highest overhead. The choice of smart pointer should be driven by ownership requirements and performance considerations. If performance is paramount and single ownership is sufficient, unique_ptr
is the clear winner. If shared ownership is needed, the performance cost of shared_ptr
must be carefully evaluated against the benefits of shared ownership.
The above is the detailed content of How do smart pointers (unique_ptr, shared_ptr, weak_ptr) work in C and when should I use them?. For more information, please follow other related articles on the PHP Chinese website!
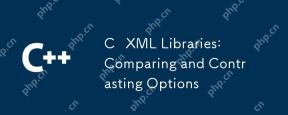
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
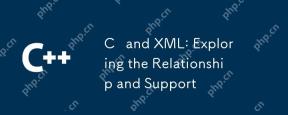
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
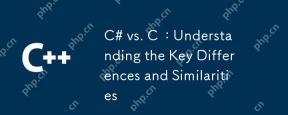
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
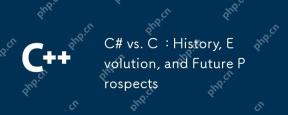
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
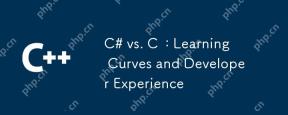
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
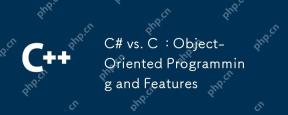
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
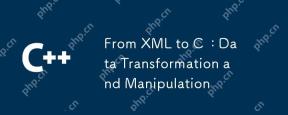
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
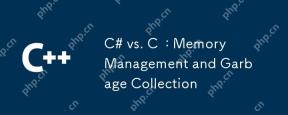
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
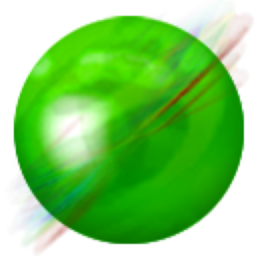
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment