This article guides Python programmers on designing object-oriented programs. It covers identifying objects, defining classes, establishing relationships (inheritance, composition), and applying OOP principles like encapsulation, abstraction, and po
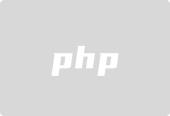
How Do I Design a Python Program Using OOP Principles?
Designing a Python program using Object-Oriented Programming (OOP) principles involves structuring your code around objects, which combine data (attributes) and methods (functions) that operate on that data. Here's a step-by-step approach:
-
Identify Objects: Begin by identifying the key entities in your program. What are the nouns? These often represent potential objects. For example, in a banking application, you might have
Account
, Customer
, and Transaction
objects.
-
Define Classes: Each object type becomes a class. A class is a blueprint for creating objects. Define attributes (data) within the class using variables, and define methods (behavior) using functions. For instance, the
Account
class might have attributes like account_number
, balance
, and methods like deposit()
, withdraw()
, and get_balance()
.
-
Establish Relationships: Consider how objects interact. Do they inherit properties from each other (inheritance)? Do they have a "has-a" relationship (composition)? For example, a
Customer
object might have multiple Account
objects.
-
Encapsulation: Hide internal data (attributes) and implementation details within the class. Expose only necessary information through methods (getters and setters). This protects data integrity and improves code maintainability.
-
Abstraction: Provide a simplified interface to complex processes. Users shouldn't need to understand the internal workings of a method to use it. The
deposit()
method handles the details of updating the balance; the user simply calls the method.
-
Polymorphism: Allow objects of different classes to respond to the same method call in their own specific way. For example, both
SavingsAccount
and CheckingAccount
classes might have a calculate_interest()
method, but the calculation would differ for each account type.
-
Inheritance: Create new classes (child classes) that inherit attributes and methods from existing classes (parent classes). This promotes code reusability and reduces redundancy. A
SavingsAccount
class could inherit from a base Account
class.
What are the key benefits of using OOP in Python programming?
OOP offers several significant advantages in Python development:
-
Modularity: Code is organized into self-contained modules (classes), making it easier to understand, maintain, and reuse. Changes in one part of the program are less likely to affect other parts.
-
Reusability: Inheritance allows you to create new classes based on existing ones, avoiding redundant code.
-
Maintainability: Well-structured OOP code is easier to debug, modify, and extend. Changes are localized, minimizing the risk of introducing errors.
-
Scalability: OOP facilitates the development of large, complex applications. The modular design makes it easier to manage the growth of the project.
-
Readability: OOP code tends to be more readable and understandable than procedural code, as it mirrors real-world objects and their relationships.
-
Data Protection: Encapsulation protects data integrity by restricting direct access to attributes. Data is modified only through controlled methods.
How can I effectively manage classes and objects in a large Python OOP project?
Managing classes and objects in a large project requires careful planning and organization:
-
Modular Design: Break down the project into smaller, manageable modules, each responsible for a specific aspect of the application. Each module can contain related classes.
-
Package Structure: Organize your modules into packages to improve code organization and avoid naming conflicts. Use descriptive package and module names.
-
Design Patterns: Employ established design patterns (discussed in the next section) to solve common design problems and improve code structure.
-
Version Control: Use a version control system like Git to track changes, collaborate effectively, and manage different versions of your code.
-
Testing: Write unit tests to verify the correctness of individual classes and methods. Use testing frameworks like pytest or unittest.
-
Documentation: Document your classes, methods, and modules thoroughly using docstrings and external documentation tools like Sphinx.
-
Refactoring: Regularly refactor your code to improve its structure, readability, and maintainability. Address code smells and eliminate redundancies.
-
Code Reviews: Have other developers review your code to identify potential problems and improve code quality.
What are some common design patterns suitable for Python OOP projects?
Several design patterns can significantly enhance the structure and maintainability of your Python OOP projects:
-
Singleton Pattern: Ensures that only one instance of a class is created. Useful for managing resources or configurations.
-
Factory Pattern: Provides an interface for creating objects without specifying their concrete classes. Useful for creating objects based on different configurations or conditions.
-
Observer Pattern: Defines a one-to-many dependency between objects, where a change in one object automatically notifies its dependents. Useful for event handling and notifications.
-
Decorator Pattern: Dynamically adds responsibilities to an object without altering its structure. Useful for adding functionality to existing classes without modifying their code.
-
Adapter Pattern: Converts the interface of a class into another interface clients expect. Useful for integrating classes with incompatible interfaces.
-
Strategy Pattern: Defines a family of algorithms, encapsulates each one, and makes them interchangeable. Useful for selecting different algorithms at runtime.
-
Template Method Pattern: Defines the skeleton of an algorithm in a base class, allowing subclasses to override specific steps without changing the algorithm's overall structure. Useful for defining common steps in a process with variations in individual steps.
These are just a few examples; the choice of pattern depends on the specific needs of your project. Understanding these patterns will greatly improve your ability to design robust and maintainable OOP applications in Python.
The above is the detailed content of How Do I Design a Python Program Using OOP Principles?. For more information, please follow other related articles on the PHP Chinese website!
Statement:The content of this article is voluntarily contributed by netizens, and the copyright belongs to the original author. This site does not assume corresponding legal responsibility. If you find any content suspected of plagiarism or infringement, please contact admin@php.cn