Laravel's session blocking mechanism safeguards against race conditions and data inconsistencies by regulating simultaneous access to sessions. This ensures data integrity during concurrent operations.
Understanding Session Blocking
Effective session blocking hinges on these prerequisites:
- A cache driver capable of atomic locking (Redis, Memcached, DynamoDB, or a relational database).
- A non-cookie-based session driver.
The following code snippet demonstrates its basic usage:
Route::post('/endpoint', function() { // Application logic here })->block($lockSeconds = 5, $waitSeconds = 10);
Real-World Application: Payment Processing
Let's illustrate session blocking within a payment processing system designed for concurrency control:
<?php namespace App\Http\Controllers; use App\Models\Payment; use Illuminate\Http\Request; use Illuminate\Support\Facades\DB; use App\Exceptions\PaymentException; class PaymentController extends Controller { public function process(Request $request) { return DB::transaction(function() use ($request) { // Verify payment existence and unprocessed status $payment = Payment::findOrFail($request->payment_id); if ($payment->isProcessed()) { throw new PaymentException('Payment already processed.'); } // Initiate payment processing $result = $this->paymentGateway->charge([ 'amount' => $payment->amount, 'currency' => $payment->currency, 'token' => $request->payment_token ]); $payment->markAsProcessed($result->transaction_id); return response()->json([ 'status' => 'success', 'transaction_id' => $result->transaction_id ]); }); } } // routes/api.php Route::post('/payments/process', [PaymentController::class, 'process'])->block(5, 10);
This refined implementation:
- Prevents duplicate payment processing.
- Imposes a 10-second timeout for lock acquisition.
- Leverages database transactions for atomicity.
- Elegantly handles concurrent requests.
In conclusion, Laravel's session blocking offers a robust approach to managing concurrent requests, ensuring data integrity in high-traffic applications while maintaining a streamlined, Laravel-native implementation.
The above is the detailed content of Managing Concurrent Requests with Laravel Session Blocking. For more information, please follow other related articles on the PHP Chinese website!
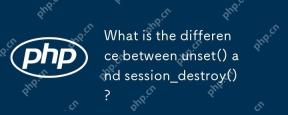
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
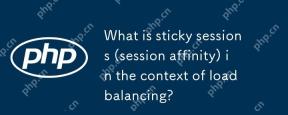
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
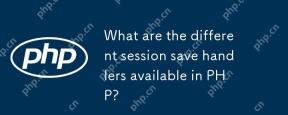
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
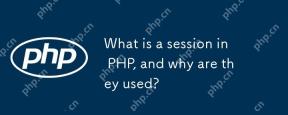
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
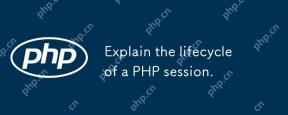
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
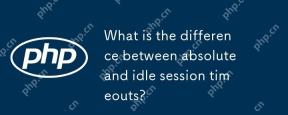
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
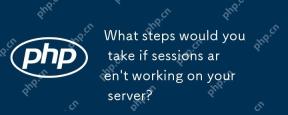
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
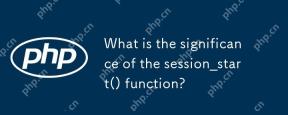
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor

Zend Studio 13.0.1
Powerful PHP integrated development environment

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
