Laravel's transform()
helper function offers a streamlined approach to managing conditional data modifications, particularly useful when dealing with potentially null values. This tutorial explores its functionality and demonstrates its application in enhancing data processing within Laravel applications.
Understanding transform()
The transform()
helper simplifies data manipulation by accepting three arguments:
- The data value: The input data to be transformed.
- A callback function: Executed only if the data value is not null. This function performs the desired transformation.
- A default value (optional): Returned if the data value is null.
// Basic usage: Convert to uppercase $result = transform('hello world', fn ($text) => strtoupper($text)); // Output: HELLO WORLD // Handling null values: $result = transform(null, fn ($value) => $value * 2, 'default'); // Output: 'default'
Practical Applications of transform()
Let's illustrate transform()
's utility in a user profile scenario:
<?php namespace App\Http\Controllers; use App\Models\User; use Illuminate\Http\Request; class ProfileController extends Controller { public function formatUserData(User $user) { return [ 'profile' => transform($user->profile, function ($profile) { return [ 'display_name' => transform( $profile->name, fn ($name) => ucwords(strtolower($name)), 'Anonymous User' ), 'avatar' => transform( $profile->avatar_url, fn ($url) => asset($url), '/images/default-avatar.png' ), 'bio' => transform( $profile->biography, fn ($bio) => str_limit($bio, 160), 'No biography provided' ), 'joined_date' => transform( $profile->created_at, fn ($date) => $date->format('F j, Y'), 'Recently' ) ]; }, [ 'display_name' => 'Guest User', 'avatar' => '/images/guest.png', 'bio' => 'Welcome, guest!', 'joined_date' => 'N/A' ]) ]; } }
Another example involving configuration values:
<?php namespace App\Services; class CacheService { public function getCacheTimeout() { return transform( config('cache.timeout'), fn ($timeout) => $timeout * 60, 3600 ); } }
transform()
vs. Traditional Conditionals
Compare the conciseness of transform()
with a traditional conditional approach:
// Traditional method $displayName = $user->name ? ucwords($user->name) : 'Guest'; // Using transform() $displayName = transform($user->name, fn ($name) => ucwords($name), 'Guest');
transform()
significantly improves code readability and maintainability while elegantly handling null values and data transformations. Its use leads to cleaner, more efficient Laravel code.
The above is the detailed content of Enhancing Data Processing with Laravel's transform() Method. For more information, please follow other related articles on the PHP Chinese website!
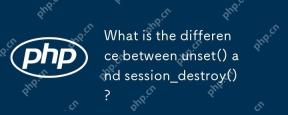
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
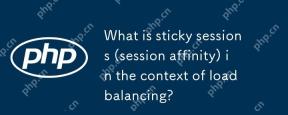
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
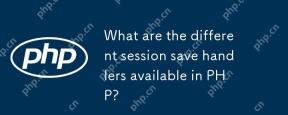
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
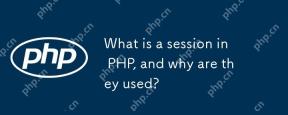
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
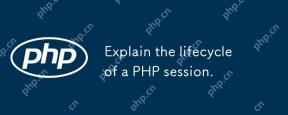
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
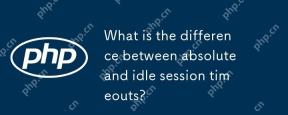
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
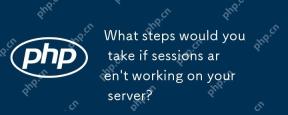
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
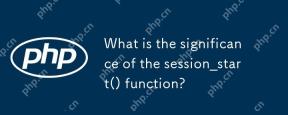
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
