Key Points
- PHP provides a large number of built-in string processing functions that can manipulate strings in various ways. These functions include changing the case of a string, finding the length of a string, replacing part of the string, and so on. Key functions include
strlen()
,str_replace()
,strpos()
,strtolower()
,strtoupper()
,substr()
, and . -
trim()
Theltrim()
function in PHP can remove spaces at the beginning and end of a string or other specified characters, which helps to clean up user input before processing. Thertrim()
and functions perform similar operations, but only remove characters on the left or right side of the string, respectively. -
substr()
Thestrpos()
function in PHP is used to intercept strings and can specify where to start intercepting and how long it is intercepted. Use this in conjunction with thestr_replace()
function to extract text from a string accurately. The function allows replacing specific words or characters in a string.
PHP has a large number of built-in string handling functions that allow you to easily manipulate strings in almost any way possible. However, it can be a bit daunting to learn all of these functions, remembering their functions and the time when they may come in handy, especially for novice developers. It is impossible to cover every string function in one article, and the PHP manual exists for this! But I'll show how to use some common string handling functions you should know. After learning, you will be able to manipulate strings as skillfully as any performer!
Case conversion
strtoupper()
PHP provides functions that enable you to manipulate the case of characters in strings without editing strings character by character. Why do you care about this? Maybe you want to make sure that some text is capitalized in all, such as acronyms, titles, to emphasize or just to make sure that the name is capitalized correctly. Or, you might just want to compare two strings, and you want to make sure the letters you are comparing are the same character set. The case conversion function is easy to master; you just need to pass the string as a parameter to the function, and the return value is the processed string. If you want to make sure all letters in a specific string are capitalized, you can use the
<?php $str = "Like a puppet on a string."; $cased = strtoupper($str); // 显示:LIKE A PUPPET ON A STRING. echo $cased; ?>
strtolower()
It may be obvious, but it is still worth noting that numbers and other non-alphabetical characters are not converted. As you might guess, the strtoupper()
function does exactly the opposite of
<?php $str = "LIKE A PUPPET ON A STRING."; $cased = strtolower($str); // 显示:like a puppet on a string. echo $cased; ?>
ucwords()
At other times, you may want to make sure that certain words (such as names or titles) only capitalize the first letter of each word. For this purpose, you can use the
<?php $str = "Like a puppet on a string."; $cased = strtoupper($str); // 显示:LIKE A PUPPET ON A STRING. echo $cased; ?>
You can also use the lcfirst()
and ucfirst()
functions to manipulate the case of the first letter of a string. If you want to lowercase the first letter, use lcfirst()
. If you want to capitalize the first letter, use ucfirst()
. The ucfirst()
function is probably the most useful because you can use it to make sure that sentences always start with capital letters.
<?php $str = "LIKE A PUPPET ON A STRING."; $cased = strtolower($str); // 显示:like a puppet on a string. echo $cased; ?>
Quick trim
Sometimes it is necessary to trim the edges of the string. It may have spaces or other characters at the beginning or end that need to be removed. Spaces can be actual space characters, or tab characters, carriage return characters, etc. An example of a situation where you might need to do this is when you process user input and want to clean it up before starting processing. The trim()
function in PHP allows you to do this; you can pass a string as a parameter and will delete all spaces at the beginning and end of the string:
<?php $str = "a knot"; $cased = ucwords($str); // 显示:A Knot echo $cased; ?>
trim()
is also versatile, in addition to strings, you can pass a set of characters that will remove any characters that match it at the beginning or end:
<?php $str = "how long is a piece of string?"; $cased = ucfirst($str); // 显示:How long is a piece of string? echo $cased; ?>
Be careful when using these additional characters, because trim()
The space will be removed only if you explicitly provide one of the characters you want to delete as a space:
<?php $str = " A piece of string? "; // 显示:string(22) " A piece of string? " var_dump($str); $trimmed = trim($str); // 显示:string(18) "A piece of string?" var_dump($trimmed); ?>
Even if trim()
only deletes characters at the beginning and end of the string, it also deletes "A" and spaces because when "A" is deleted, the spaces become the new beginning of the string and are therefore deleted. There are also ltrim()
and rtrim()
functions in PHP, which are similar to trim()
functions, but only delete spaces (or other specified characters) on the left or right side of the string, respectively.
String length
You often need to know its length when processing a string. For example, when working on a form, you might have a field that you want to make sure that the user cannot exceed a certain number of characters. To calculate the number of characters in a string, use the strlen()
function:
<?php $str = "A piece of string?"; $trimmed = trim($str, "A?"); // 显示:string(16) " piece of string" var_dump($trimmed); ?>
Intercept the string
Another common situation is to look up a specific text in a given string and "cut" it out so that you can do other operations on it. To cut a string to a specified size, you need a good pair of scissors, in PHP, your scissors are the substr()
function. To use the substr()
function, pass the string to be processed as a parameter, as well as a positive or negative integer. This number determines where you will start cutting the string; 0 starts with the first character of the string (remember, when you iterate through the string, the first character on the left starts at position 0, not 1).
<?php $str = "A piece of string?"; $trimmed = trim($str, "A ?"); // 显示:string(15) "piece of string" var_dump($trimmed); ?>
When using a negative number, substr()
will start backward from the end of the string.
<?php $str = "How long is a piece of string?"; $size = strlen($str); // 显示:30 echo $size; ?>The optional third parameter for
substr()
is length, another integer value, which allows you to specify the number of characters to be extracted from the string.
<?php $str = "Like a puppet on a string."; $cased = strtoupper($str); // 显示:LIKE A PUPPET ON A STRING. echo $cased; ?>
If you only need to find the location of the specific text in the string without any other operation, you can use the strpos()
function, which returns the position of your choice relative to the beginning of the string. A useful trick, especially if you don't know the starting position of the text you want to cut from a string, is to use these two functions together. Instead of specifying the start position as an integer, you can search for a specific text and then extract that text.
<?php $str = "LIKE A PUPPET ON A STRING."; $cased = strtolower($str); // 显示:like a puppet on a string. echo $cased; ?>
Replace
Finally, let's see how to replace part of a string with something else, for which the str_replace()
function can be used. This is great for cases where you just want to replace instances of a specific word or a set of characters in a string and replace it with something else:
<?php $str = "a knot"; $cased = ucwords($str); // 显示:A Knot echo $cased; ?>
If you want to replace multiple values, you can also provide an array for str_replace()
:
<?php $str = "how long is a piece of string?"; $cased = ucfirst($str); // 显示:How long is a piece of string? echo $cased; ?>
str_replace()
is case sensitive, so if you don't want to worry about this, you can use its case-insensitive sibling function str_ireplace()
.
Summary
I hope this article has given you some of the operations of using strings in PHP and makes you eager to learn more. I actually just touched on the fur! The best way to understand all the different string functions is to spend some time reading the String Functions page in the PHP manual. I'd love to know which string functions you use most often, so feel free to mention them in the comments below. Pictures from Vasilius / Shutterstock
Frequently Asked Questions for PHP String Processing Functions
What are some common PHP string processing functions and their uses?
PHP provides a wide range of string processing functions. Some of the most commonly used functions include:
-
strlen()
: This function returns the length of the string. -
str_replace()
: This function replaces certain characters with other characters in the string. -
strpos()
: This function finds where the string first appears in another string. -
strtolower()
: This function converts a string to lowercase. -
strtoupper()
: This function converts a string to uppercase. -
substr()
: This function returns part of the string.
These functions are used to manipulate and process strings in various ways, such as changing the case of a string, finding the length of a string, replacing part of the string, and so on.
How to convert a string to an array in PHP?
In PHP, the str_split()
function is used to convert strings into arrays. This function splits the string into an array by length. Here is an example:
<?php $str = " A piece of string? "; // 显示:string(22) " A piece of string? " var_dump($str); $trimmed = trim($str); // 显示:string(18) "A piece of string?" var_dump($trimmed); ?>
In this example, the str_split()
function splits the string "Hello, World!" into an array where each element is a single character in the string.
How to compare two strings in PHP?
PHP provides several functions for comparing strings, including strcmp()
, strcasecmp()
, strnatcmp()
, and strnatcasecmp()
. The strcmp()
function performs binary-safe and case-sensitive comparisons of two strings. Here is an example:
<?php $str = "Like a puppet on a string."; $cased = strtoupper($str); // 显示:LIKE A PUPPET ON A STRING. echo $cased; ?>
In this example, the strcmp()
function compares the strings "Hello" and "World". If string1 is greater than string2, the function returns 0, and if they are equal, 0.
How to reverse a string in PHP?
The strrev()
function in PHP is used to invert strings. Here is an example:
<?php $str = "LIKE A PUPPET ON A STRING."; $cased = strtolower($str); // 显示:like a puppet on a string. echo $cased; ?>
In this example, the strrev()
function inverts the string "Hello, World!".
How to remove spaces from PHP strings?
The trim()
function in PHP is used to remove spaces and other predefined characters from both sides of a string. Here is an example:
<?php $str = "a knot"; $cased = ucwords($str); // 显示:A Knot echo $cased; ?>
In this example, the trim()
function deletes the spaces at the beginning and end of the string "Hello, World!".
The above is the detailed content of PHP String Handling Functions. For more information, please follow other related articles on the PHP Chinese website!
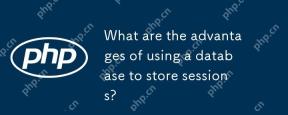
The main advantages of using database storage sessions include persistence, scalability, and security. 1. Persistence: Even if the server restarts, the session data can remain unchanged. 2. Scalability: Applicable to distributed systems, ensuring that session data is synchronized between multiple servers. 3. Security: The database provides encrypted storage to protect sensitive information.
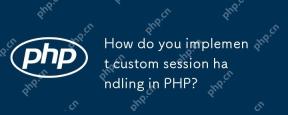
Implementing custom session processing in PHP can be done by implementing the SessionHandlerInterface interface. The specific steps include: 1) Creating a class that implements SessionHandlerInterface, such as CustomSessionHandler; 2) Rewriting methods in the interface (such as open, close, read, write, destroy, gc) to define the life cycle and storage method of session data; 3) Register a custom session processor in a PHP script and start the session. This allows data to be stored in media such as MySQL and Redis to improve performance, security and scalability.
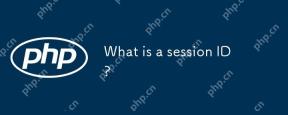
SessionID is a mechanism used in web applications to track user session status. 1. It is a randomly generated string used to maintain user's identity information during multiple interactions between the user and the server. 2. The server generates and sends it to the client through cookies or URL parameters to help identify and associate these requests in multiple requests of the user. 3. Generation usually uses random algorithms to ensure uniqueness and unpredictability. 4. In actual development, in-memory databases such as Redis can be used to store session data to improve performance and security.
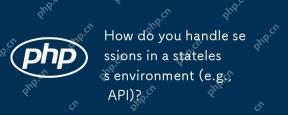
Managing sessions in stateless environments such as APIs can be achieved by using JWT or cookies. 1. JWT is suitable for statelessness and scalability, but it is large in size when it comes to big data. 2.Cookies are more traditional and easy to implement, but they need to be configured with caution to ensure security.
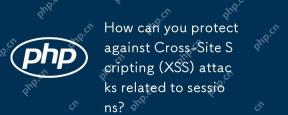
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
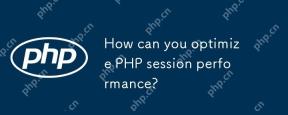
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
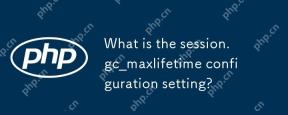
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 Chinese version
Chinese version, very easy to use

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.