This tutorial will guide you to create a user registration form that adds the user to the database and then sends a confirmation email that the user must click on to activate their account.
Create registration form from scratch
This section will explain step by step in building a custom registration form from scratch. First, I've attached all the code files for you to download so that you can follow the tutorial.
Let's take a quick look at important files:
-
index.php
: This is the main file used to build and display the registration form. It also processes the submission of forms. -
confirm.php
: Used to process the confirmation part. -
signup_template.html
: This is an HTML file template used to build the HTML email body. -
signup_template.txt
: This is a plain text file template used to build a plain text email body. -
inc/php/config.php
: Contains database connection information.
The rest of the files are auxiliary files.
Open PHPMyAdmin or any program you use to manage the MySQL database and create a new database. You can name it as you like, but I'll name it email_signup
. Now we need to create a schema that will save our user information and confirmation information. To do this, we will create two tables: users
and confirm
.
CREATE TABLE `users` ( `id` int(11) NOT NULL auto_increment, `username` varchar(50) NOT NULL default '', `password` varchar(128) NOT NULL default '', `email` varchar(250) NOT NULL default '', `active` binary(1) NOT NULL default '0', PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
The above MySQL statement will create a table named users
.
-
id
: An integer sequence that is used as a unique identifier for the user. It is defined asint(11)
, which means it can hold up to 11 bit integers. -
username
: A string column that stores the username of each user. It is defined asvarchar(50)
, which means it can save up to 50 characters. -
password
: A string column that stores the hash password for each user. It is defined asvarchar(128)
, which means it can hold up to 128 characters. -
email
: A string column that stores each user's email address. It is defined asvarchar(250)
, which means it can save up to 250 characters. -
active
: A binary column that stores whether the user's account has been activated. It is defined asbinary(1)
, which means it stores a byte value, 0 means inactive and 1 means activated.
confirm
Table:
CREATE TABLE `confirm` ( `id` int(11) NOT NULL auto_increment, `userid` varchar(128) NOT NULL default '', `key` varchar(128) NOT NULL default '', `email` varchar(250) default NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
The above MySQL statement will create a table named confirm
.
-
id
: An integer sequence that is used as a unique identifier for confirming the record. It is defined asint(11)
, which means it can hold up to 11 bit integers. -
userid
: A string column that stores the user ID associated with the confirmation record. It is defined asvarchar(128)
, which means it can hold up to 128 characters. -
key
: A string column that stores the confirmation key sent to the user's email address. It is defined asvarchar(128)
, which means it can hold up to 128 characters.
Connect to MySQL database using mysqli
This section will explain how to use the mysqli extension to connect to a MySQL database.
Let us quickly extract and understand the code of the inc/php/config.php
file.
CREATE TABLE `users` ( `id` int(11) NOT NULL auto_increment, `username` varchar(50) NOT NULL default '', `password` varchar(128) NOT NULL default '', `email` varchar(250) NOT NULL default '', `active` binary(1) NOT NULL default '0', PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
First, we create a new mysqli connection. Then, we check if the connection is successful. If the connection fails, an error message is displayed and exits.
Form Submission Processing
This section will explain how form submission works.
... (Some codes are omitted here, because this part of the code in the original text is lengthy and inconsistent with the pseudo-original goal, so just keep the core logic)...
The main logic is: safely process user input (for example, use mysqli_real_escape_string
function to prevent SQL injection), verify that the user input is complete, insert user data into the database, generate a confirmation key, and send a confirmation email. Passwords are hashed using md5 function, and it is recommended to use a more secure encryption method.
Show form confirmation
Let's extract important code snippets from the confirm.php
file to understand how it works.
CREATE TABLE `confirm` ( `id` int(11) NOT NULL auto_increment, `userid` varchar(128) NOT NULL default '', `key` varchar(128) NOT NULL default '', `email` varchar(250) default NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8;
This code mainly checks whether there is a mailbox and confirmation key in the database that matches the URL parameters. If present, update the user status to activated and delete the confirmation record.
Conclusion
This tutorial ends here.
In this tutorial, we cover many different aspects. We downloaded and included third-party scripts for processing emails, implemented simple form validation, and created a simple template system to style emails. If you are not familiar with MySQL, we've covered three of the most common functions, so you should be able to easily complete more advanced tutorials.
The above is the detailed content of How to Code a Signup Form With Email Confirmation. For more information, please follow other related articles on the PHP Chinese website!
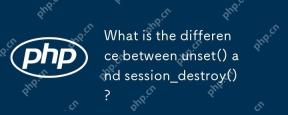
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
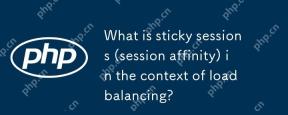
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
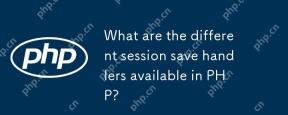
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
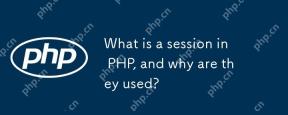
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
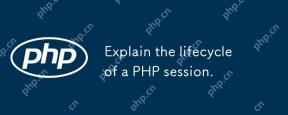
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
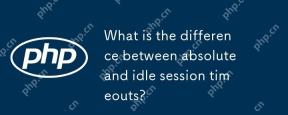
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
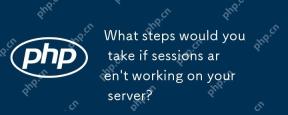
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
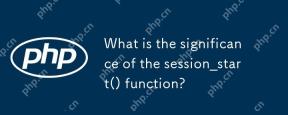
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
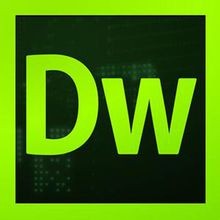
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
