Key Points
- Cross-site scripting attack (XSS) is a common code injection attack that occurs due to improper user data verification (usually inserting or manipulating hyperlinks via web forms). This may allow harmful client code to be saved on the server or executed in the user's browser.
- XSS attacks can be divided into two types: non-persistent XSS (malicious code is passed through the server and presented to the victim) and persistent XSS (harmful code bypasses verification and is stored in the website's data store and is executed when information is displayed on the website).
- Preventing XSS attacks requires never trusting data from users or third-party sources, verifying all data at input, and escaping it when output. This includes implementing data verification, data cleaning and output escaping measures.
- While many PHP frameworks provide built-in security measures, it is critical to continuously test validation code with the latest XSS test vectors to ensure that the code remains unaffected by XSS attacks.
Cross-site scripting attacks are one of the five major security attacks that occur every day on the Internet, and your PHP scripts may not be spared. This attack, also known as XSS, is basically a code injection attack that is possible due to incorrect verification of user data, which is usually inserted into a page via a web form or using a changed hyperlink. The injected code can be any malicious client code, such as JavaScript, VBScript, HTML, CSS, Flash, etc. This code is used to save harmful data on the server or perform malicious actions in the user's browser. Unfortunately, cross-site scripting attacks occur mainly because developers fail to provide secure code. It is a responsibility for every PHP programmer to understand how to attack their PHP scripts to exploit possible security vulnerabilities. Read this article and you will learn more about cross-site scripting attacks and how to prevent them in your code.
Learn through examples
Let's look at the following code snippet.
<form action="post.php" method="post"> <input type="text" name="comment" value=""> <input type="submit" name="submit" value="Submit"> </form>
Here we have a simple form with a text box for data entry and a submit button. After submitting the form, it submits the data to post.php for processing. Assume post.php only outputs data, as shown below:
<?php echo $_POST["comment"]; ?>
Without any filtering, the hacker can submit the following through the form, which will generate a popup in the browser with the message "hacked".
alert("hacked")
This example, although malicious in nature, does not seem to cause much harm. But think about what happens if JavaScript code is written to steal the user's cookies and extract sensitive information from it? There are worse XSS attacks than simple alert() calls.
Cross-site scripting attacks can be divided into two categories based on how they deliver malicious payloads: non-persistent XSS and persistent XSS. Please allow me to discuss each type in detail.
Non-persistent XSS
Also known as a reflective XSS attack, it means that malicious code is not actually stored on the server, but is passed through and presented to the victim, which is the more popular XSS strategy among the two delivery methods. Attacks are initiated from external sources, such as emails or third-party websites. Here is a part of a simple search result script:
<form action="post.php" method="post"> <input type="text" name="comment" value=""> <input type="submit" name="submit" value="Submit"> </form>
This example may be a very unsafe outcome page where the search query will be displayed back to the user. The problem here is that the $_GET["query"]
variable is not verified or escaped, so the attacker can send the following link to the victim:
<?php echo $_POST["comment"]; ?>
No verification is required, the page will contain:
alert("hacked")
Permanence XSS
This type of attack occurs when the malicious code has passed the verification process and is stored in the data store. This could be a comment, log file, notification message, or any other part of the website that requires user input. Later, when this specific information is displayed on the website, the malicious code executes. Let's use the following example to create a basic file-based comment system. Assuming the same form I introduced earlier, assuming the receiving script just appends the comments to the data file.
<?php // 根据查询获取搜索结果 echo "You searched for: " . $_GET["query"]; // 列出搜索结果 ...
Other places, the content of comments.txt is displayed to the visitor:
http://example.com/search.php?query=alert("hacked")
When a user submits a comment, it is saved to the data file. The entire file (and therefore the entire comment series) will then be displayed to the reader. If malicious code is submitted, it will be saved and displayed as is without any validation or escape.
Prevent cross-site scripting attacks
Luckily, it's as easy as XSS attacks on unprotected sites, and it's just as easy to prevent them. However, prevention must always be kept in mind even before writing a line of code. The first rule that any web environment (whether development, staging or production) needs to be "enforced" is to never trust data from users or any other third-party sources. This cannot be emphasized too much. Each bit of data input must be verified and escaped at output. This is the golden rule to prevent XSS. To implement reliable security measures to prevent XSS attacks, we should pay attention to data verification, data cleaning, and output escaping.
Data Verification
Data verification is the process of ensuring that the application runs with the correct data. If your PHP script expects the user to enter an integer, any other type of data will be discarded. Each piece of user data received must be verified to ensure its type is correct and discarded if it fails the verification process. For example, if you want to verify a phone number, you will discard any string containing the letters, because the phone number should contain only numbers. You should also consider the length of the string. If you want to be a little looser, you can allow a limited set of special characters, such as plus signs, brackets, and dashes, which are often used to format phone numbers specific to your target locale.
<form action="post.php" method="post"> <input type="text" name="comment" value=""> <input type="submit" name="submit" value="Submit"> </form>
Data Cleaning
Data cleaning focuses on manipulating data to ensure it is safe by removing any unwanted bits from the data and normalizing it into the correct form. For example, if you expect plain text strings as user input, you may want to remove any HTML tags from it.
<?php echo $_POST["comment"]; ?>
Sometimes, data verification and cleaning/normalization can be performed simultaneously.
alert("hacked")
Output escape
To protect the integrity of the display/output data, you should escape the data when it is presented to the user. This prevents the browser from applying any unexpected meaning to any special character sequence that may be found.
<?php // 根据查询获取搜索结果 echo "You searched for: " . $_GET["query"]; // 列出搜索结果 ...
Together now!
To better understand the three aspects of data processing, let's look at the previous file-based comment system again and modify it to ensure its security. The potential vulnerability in the code stems from the fact that $_POST["comment"]
is blindly attached to the comments.txt file and then displayed directly to the user. To ensure it is safe, the $_POST["comment"]
value should be verified and cleaned before adding it to the file, and it should be escaped when the file content is displayed to the user.
http://example.com/search.php?query=alert("hacked")
The script first verifies incoming comments to ensure that the user has provided a non-zero-length string. After all, blank comments are not very interesting. Data validation needs to be done in a well-defined context, which means that if I expect to get an integer from the user, then I validate it accordingly by converting the data into an integer and processing it as an integer. If this results in invalid data, just discard it and let the user know. The script then cleans up the comments by removing any HTML tags that may be included. Finally, retrieve, filter, and display comments. Typically, the htmlspecialchars()
function is sufficient to filter the output intended to be viewed in the browser. However, if the character encoding you are using in a web page is not ISO-8859-1 or UTF-8, you may need to use htmlentities()
. For more information about these two functions, read the respective descriptions in the official PHP documentation. Remember that there is no single solution that is 100% secure on an evolving medium like the Web. Thoroughly test your verification code with the latest XSS test vectors. Using the test data from the following sources should reveal whether your code is still vulnerable to XSS attacks.
- RSnake XSS cheatsheet (a fairly comprehensive list of XSS vectors that you can use to test your code)
- Zend Framework's XSS test data
- XSS cheatsheet (using HTML5 features)
Summary
Hope this article explains you very well what cross-site scripting attacks are and how to prevent them from happening in your code. Never trust data from users or any other third-party sources. You can protect yourself by validating incoming values in a well-defined context, cleaning up data to protect your code, and escaping output to protect your users. After writing the code, make sure you work properly by testing the code as thoroughly as possible.
(Picture from Inge Schepers / Shutterstock)
If you like this post, you will like Learnable; a place to learn new skills and skills from masters. Members can instantly access all SitePoint's e-books and interactive online courses, such as Jump Start PHP.
Comments in this article have been closed. Have questions about PHP? Why not ask questions on our forum?
FAQs (FAQ) about PHP security and cross-site scripting attacks (XSS)
What is the impact of cross-site scripting (XSS) attacks on PHP applications?
Cross-site scripting (XSS) attacks can have a significant impact on PHP applications. They can lead to data theft, session hijacking, website corruption, and even distribution of malicious code to users. XSS attacks exploit vulnerabilities in web applications to inject malicious scripts and then execute them by the user's browser. This can jeopardize user interaction with the application and may disclose sensitive information.
How to identify potential XSS vulnerabilities in my PHP application?
Identifying potential XSS vulnerabilities in PHP applications requires a combination of manual code review and automated testing. Find areas in the code where user input is directly included in the output without proper cleaning or verification. Automation tools like XSS Scanners can also help identify potential vulnerabilities by testing various XSS attack vectors.
What are some common methods used in XSS attacks?
XSS attacks usually involve injecting malicious scripts into web pages viewed by other users. This can be done in a variety of ways, such as embedding scripts into URL parameters, form inputs, and even cookies. The malicious script can then perform actions on behalf of the user, such as stealing their session cookies or manipulating web page content.
How to prevent XSS attacks in my PHP application?
Preventing XSS attacks in PHP applications involves validating and cleaning user input, encoded output, and using appropriate HTTP headers. Always treat user input as untrusted and validate it against the allowable list of acceptable values. Clean the input to remove any characters or code that may be harmful. The output is encoded to ensure that any characters that may be harmful become harmless. Use HTTP headers such as content security policies to limit the source of scripts and other resources.
What role does content security policies play in preventing XSS attacks?
Content Security Policy (CSP) HTTP headers play a crucial role in preventing XSS attacks. It allows you to specify a domain that the browser should consider to be a valid source of executable scripts. This means that even if an attacker can inject a script into your web page, the browser won't run it unless the source of the script is whitelisted in your CSP.
What is the difference between a storage XSS attack and a reflective XSS attack?
Storage XSS attacks involve injecting malicious scripts that are permanently stored on the target server. Then, when the user views certain pages, the script is provided to the user. Reflective XSS attacks, on the other hand, involve injecting a script through a URL or form input, which the server immediately returns in the response and executes by the user's browser.
How to use PHP's built-in functions to prevent XSS attacks?
PHP provides some built-in functions that can help prevent XSS attacks. For example, the htmlspecialchars()
function can be used to encode special characters in user input, making potential scripts harmless. filter_input()
Functions can be used to clean up user input, delete or encode harmful characters.
What role does HTTPOnly cookies play in preventing XSS attacks?
HTTPOnly Cookie is a cookie that cannot be accessed through client scripts. This means that even if an attacker can inject a script into your web page, they cannot use the script to read or modify HTTPOnly cookies. This can help protect sensitive information (such as session identifiers) from being stolen by XSS attacks.
Can XSS attacks be used to bypass CSRF protection?
Yes, XSS attacks may be used to bypass cross-site request forgery (CSRF) protection. If an attacker can inject a script into your web page, they can use it to perform actions on behalf of the user, potentially bypassing any CSRF protections you have implemented. This is why it is important to protect against both XSS and CSRF attacks.
What PHP frameworks provide built-in protection against XSS attacks?
Yes, many PHP frameworks provide built-in protection against XSS attacks. For example, Laravel automatically encodes the output to prevent XSS attacks. Other frameworks such as Symfony and CodeIgniter also provide functionality for cleaning user input and encoded output. However, it must be remembered that no framework can provide complete protection and you should still follow best practices for preventing XSS attacks.
The above is the detailed content of Cross-Site Scripting Attacks (XSS). For more information, please follow other related articles on the PHP Chinese website!
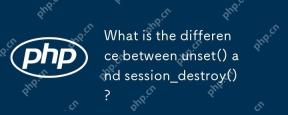
Thedifferencebetweenunset()andsession_destroy()isthatunset()clearsspecificsessionvariableswhilekeepingthesessionactive,whereassession_destroy()terminatestheentiresession.1)Useunset()toremovespecificsessionvariableswithoutaffectingthesession'soveralls
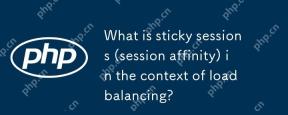
Stickysessionsensureuserrequestsareroutedtothesameserverforsessiondataconsistency.1)SessionIdentificationassignsuserstoserversusingcookiesorURLmodifications.2)ConsistentRoutingdirectssubsequentrequeststothesameserver.3)LoadBalancingdistributesnewuser
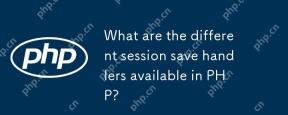
PHPoffersvarioussessionsavehandlers:1)Files:Default,simplebutmaybottleneckonhigh-trafficsites.2)Memcached:High-performance,idealforspeed-criticalapplications.3)Redis:SimilartoMemcached,withaddedpersistence.4)Databases:Offerscontrol,usefulforintegrati
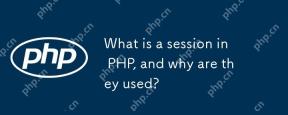
Session in PHP is a mechanism for saving user data on the server side to maintain state between multiple requests. Specifically, 1) the session is started by the session_start() function, and data is stored and read through the $_SESSION super global array; 2) the session data is stored in the server's temporary files by default, but can be optimized through database or memory storage; 3) the session can be used to realize user login status tracking and shopping cart management functions; 4) Pay attention to the secure transmission and performance optimization of the session to ensure the security and efficiency of the application.
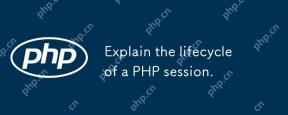
PHPsessionsstartwithsession_start(),whichgeneratesauniqueIDandcreatesaserverfile;theypersistacrossrequestsandcanbemanuallyendedwithsession_destroy().1)Sessionsbeginwhensession_start()iscalled,creatingauniqueIDandserverfile.2)Theycontinueasdataisloade
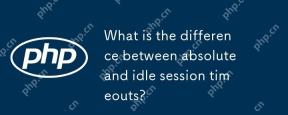
Absolute session timeout starts at the time of session creation, while an idle session timeout starts at the time of user's no operation. Absolute session timeout is suitable for scenarios where strict control of the session life cycle is required, such as financial applications; idle session timeout is suitable for applications that want users to keep their session active for a long time, such as social media.
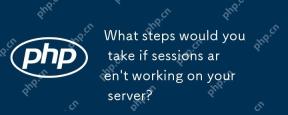
The server session failure can be solved through the following steps: 1. Check the server configuration to ensure that the session is set correctly. 2. Verify client cookies, confirm that the browser supports it and send it correctly. 3. Check session storage services, such as Redis, to ensure that they are running normally. 4. Review the application code to ensure the correct session logic. Through these steps, conversation problems can be effectively diagnosed and repaired and user experience can be improved.
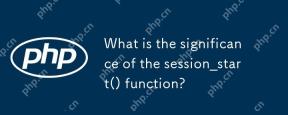
session_start()iscrucialinPHPformanagingusersessions.1)Itinitiatesanewsessionifnoneexists,2)resumesanexistingsession,and3)setsasessioncookieforcontinuityacrossrequests,enablingapplicationslikeuserauthenticationandpersonalizedcontent.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

SublimeText3 Chinese version
Chinese version, very easy to use
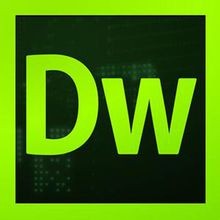
Dreamweaver CS6
Visual web development tools

Atom editor mac version download
The most popular open source editor
