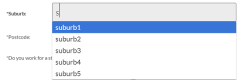
HTML5 Datalist Tag
Full jQuery
This code populates the datalist via JSON and auto fills other fields based on the field’s auto complete selection by the user.<span>window.DATALIST = { </span> <span>cache: {}, </span> <span>init: function() </span> <span>{ </span> <span>var _this = this, </span> <span>this.cache.$form = $('formid'); </span> <span>this.cache.$suburbs = this.cache.$form.find('datalist#suburbs'); </span> <span>this.cache.$suburbInput = this.cache.$form.find('input[name="suburb"]'); </span> <span>this.cache.$postcodeInput = this.cache.$form.find('input[name="postcode"]'); </span> <span>this.cache.$stateInput = this.cache.$form.find('input[name="state"]'); </span> <span>//grab the datalist options from JSON data </span> <span>var checkMembershipRequest = $.ajax({ </span> <span>type: "GET", </span> <span>dataType: "JSON", </span> <span>url: "/php/suburbs.php" </span> <span>}); </span> checkMembershipRequest<span>.done(function(data) </span> <span>{ </span> <span>console.log(data); </span> <span>//data could be cached in the browser if required for speed. </span> <span>// localStorage.postcodeData = JSON.stringify(data); </span> <span>//add options to datalist </span> $<span>.each(data.suburbs, function(i<span>,v</span>) </span> <span>{ </span> _this<span>.cache.$suburbs.append(''+i+''); </span> <span>}); </span> <span>//hook up data handler when suburb is changed to autocomplete postcode and state </span> _this<span>.cache.$suburbInput.on('change', function() </span> <span>{ </span> <span>// console.log('suburb changed'); </span> <span>var val = $(this).val(), </span> selected <span>= _this.cache.$suburbs.find('option[data-value="'+val+'"]'), </span> postcode <span>= selected.data('postcode'), </span> state <span>= selected.data('state'); </span> _this<span>.cache.$postcodeInput.val(postcode); </span> _this<span>.cache.$stateInput.val(state); </span> <span>}); </span> <span>}); </span> checkMembershipRequest<span>.fail(function(jqXHR<span>, textStatus</span>) </span> <span>{ </span> <span>console.log( "postcode request fail - an error occurred: (" + textStatus + ")." ); </span> <span>//try again... </span> <span>}); </span> <span>} </span> <span>}</span>
Full HTML
This is what your HTML might look like:*Suburb: *Postcode: State:
Full JSON
PHP file returns JSON – could be .json or .php and grab data from a database if required.<span>{ </span> <span>"suburbs": { </span> <span>"suburb1": { </span> <span>"postcode": "2016", </span> <span>"state": "NSW" </span> <span>}, </span> <span>"suburb2": { </span> <span>"postcode": "4016", </span> <span>"state": "QLD" </span> <span>}, </span> <span>"suburb3": { </span> <span>"postcode": "3016", </span> <span>"state": "CA" </span> <span>}, </span> <span>"suburb4": { </span> <span>"postcode": "8016", </span> <span>"state": "WA" </span> <span>}, </span> <span>"suburb5": { </span> <span>"postcode": "6016", </span> <span>"state": "SA" </span> <span>} </span> <span>} </span><span>}</span>
html5 trigger datalist
Use ALT down arrow to simulate user action. You’ll need to use jQuery to simulate a multiple trigger keypress. keycode ALT = 18 (also modifier key called altKey) keycode Down Arrow = 40<span>var e = jQuery.<span>Event</span>("keydown"); </span>e<span>.which = 40; </span>e<span>.altKey = true; </span><span>$("input").trigger(e);</span>
Frequently Asked Questions (FAQs) about jQuery AJAX and HTML5 Datalist Autocomplete
How can I dynamically load datalist options with AJAX in Firefox?
To dynamically load datalist options with AJAX in Firefox, you need to use the jQuery AJAX method. First, you need to create an AJAX request to your server-side script. This script should return the data you want to populate in the datalist. Once the AJAX request is successful, you can use the response data to populate the datalist. Here’s a simple example:
$.ajax({
url: 'your-server-side-script',
success: function(data) {
var dataList = $('#your-datalist-id');
dataList.empty();
$.each(data, function(index, value) {
dataList.append('
How can I use jQuery autocomplete with callback AJAX JSON?
jQuery UI provides an autocomplete widget that you can use with AJAX and JSON. You need to initialize the autocomplete widget on your input field and provide a source option. The source option should be a function that makes an AJAX request and uses the response data to populate the autocomplete suggestions. Here’s an example:
$('#your-input-id').autocomplete({
source: function(request, response) {
$.ajax({
url: 'your-server-side-script',
dataType: 'json',
data: {
term: request.term
},
success: function(data) {
response(data);
}
});
}
});
In this code, replace ‘your-input-id’ with the ID of your input field and ‘your-server-side-script’ with the URL of your server-side script.
How can I use jQuery AJAX and HTML5 datalist for autocomplete functionality?
You can use jQuery AJAX and HTML5 datalist to create an autocomplete functionality. First, you need to create an input field and a datalist in your HTML. Then, you need to use jQuery AJAX to fetch the data you want to use for the autocomplete suggestions. Once the AJAX request is successful, you can use the response data to populate the datalist. Here’s an example:
$.ajax({
url: 'your-server-side-script',
success: function(data) {
var dataList = $('#your-datalist-id');
dataList.empty();
$.each(data, function(index, value) {
dataList.append('
How can I handle errors in jQuery AJAX requests?
jQuery AJAX provides several methods to handle errors. The most common method is the ‘error’ callback option. This option is a function that is called when the AJAX request fails. The function receives three arguments: the jqXHR object, a string describing the type of error, and an optional exception object if one occurred. Here’s an example:
$.ajax({
url: 'your-server-side-script',
success: function(data) {
// handle successful response
},
error: function(jqXHR, textStatus, errorThrown) {
console.error('AJAX request failed: ' textStatus);
}
});
In this code, replace ‘your-server-side-script’ with the URL of your server-side script.
How can I use jQuery AJAX to send data to the server?
You can use the ‘data’ option in the jQuery AJAX method to send data to the server. The ‘data’ option should be an object where the property names are the data keys and the property values are the data values. The data is sent to the server in the body of the HTTP request. Here’s an example:
$.ajax({
url: 'your-server-side-script',
method: 'POST',
data: {
key1: 'value1',
key2: 'value2'
},
success: function(response) {
// handle successful response
}
});
In this code, replace ‘your-server-side-script’ with the URL of your server-side script, ‘key1’ and ‘key2’ with your data keys, and ‘value1’ and ‘value2’ with your data values.
The above is the detailed content of jQuery AJAX HTML5 Datalist Autocomplete Example. For more information, please follow other related articles on the PHP Chinese website!
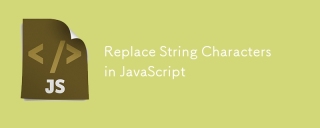
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
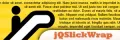
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
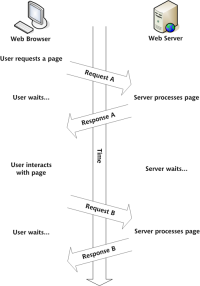
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
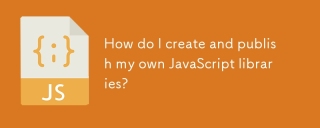
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
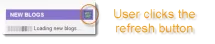
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery
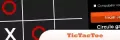
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
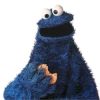
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session
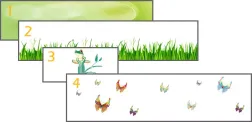
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
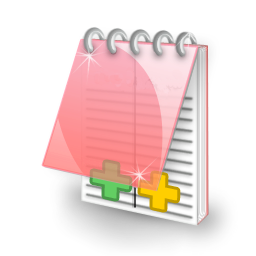
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
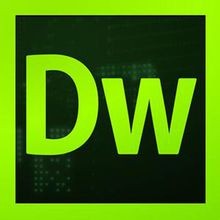
Dreamweaver CS6
Visual web development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
