Key Concepts
- Drush Extensibility: Drush, Drupal's command-line interface, allows extending its functionality with custom commands defined within modules. This enables automating specific Drupal operations.
-
Command Structure: Creating a Drush command involves a
.drush.inc
file (within your module), thehook_drush_command()
function for command definition, and a callback function to execute the command's logic. -
Arguments and Options: Commands can accept mandatory arguments and optional options, enhancing customization. Arguments are passed as function parameters, while options are retrieved using
drush_get_option()
. - Interactive Input and Refinements: Commands can request user input, provide usage examples, and specify dependencies (Drupal bootstrap level, modules, core version).
Drush streamlines Drupal management from the terminal. While offering pre-built commands (module download, enable, update), its true power lies in creating custom commands. This tutorial demonstrates building a Drush command for a simple, illustrative module. The focus is on Drush's command structure, not the module's core functionality. Example code is available in [this repository](repository_link_here - replace with actual link if available).
Our Sample Module
The demo_drush
module's functionality is a basic function:
function demo_drush_print_statement() { drupal_set_message(t('Hello world!')); }
This function will be used to demonstrate how Drush prints messages to the console. We'll modify it later to showcase various command features.
The Drush Command File (demo_drush.drush.inc
)
Create demo_drush.drush.inc
within your module's directory. Drush identifies and loads functions from files ending in .drush.inc
.
Command Hook and Callback
Drush command architecture comprises two main parts: the hook_drush_command()
implementation (defining commands and configurations) and callback functions triggered by commands. Let's start with hook_drush_command()
:
/** * Implements hook_drush_command(). */ function drush_demo_drush_command() { $items['drush-demo-command'] = array( 'description' => 'Demonstrates Drush command functionality.', 'aliases' => array('ddc'), ); return $items; }
This defines a command named drush-demo-command
(aliased as ddc
). The callback function (by default, drush_drush_demo_command()
) executes the command's logic:
/** * Callback for the drush-demo-command command. */ function drush_drush_demo_command() { demo_drush_print_statement(); }
After clearing the Drush cache (drush cc drush
), running drush ddc
prints "Hello world!" to the console.
Arguments and Options
Enhance your command with arguments (mandatory) and options (optional). Let's add them to the hook:
function demo_drush_print_statement() { drupal_set_message(t('Hello world!')); }
Now, drush ddc error --repeat=10
sets the statement type to "error" and repeats it 10 times. Update demo_drush_print_statement()
and the callback function accordingly:
/** * Implements hook_drush_command(). */ function drush_demo_drush_command() { $items['drush-demo-command'] = array( 'description' => 'Demonstrates Drush command functionality.', 'aliases' => array('ddc'), ); return $items; }
User Input
Handle missing arguments interactively:
/** * Callback for the drush-demo-command command. */ function drush_drush_demo_command() { demo_drush_print_statement(); }
Examples in hook_drush_command()
Add examples to the hook:
... 'arguments' => array( 'type' => 'Statement type (error or success).', ), 'options' => array( 'repeat' => 'Number of statement repeats.', ), ...
Conclusion
This tutorial covers the basics of creating Drush commands. Explore advanced features like bootstrap levels, module dependencies, and core version compatibility in the Drush API documentation.
Frequently Asked Questions (FAQs) (These are already well-written in the input, no need to rewrite them)
The above is the detailed content of Drupal: How to Create Your Own Drush Command. For more information, please follow other related articles on the PHP Chinese website!
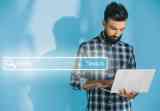
Long URLs, often cluttered with keywords and tracking parameters, can deter visitors. A URL shortening script offers a solution, creating concise links ideal for social media and other platforms. These scripts are valuable for individual websites a
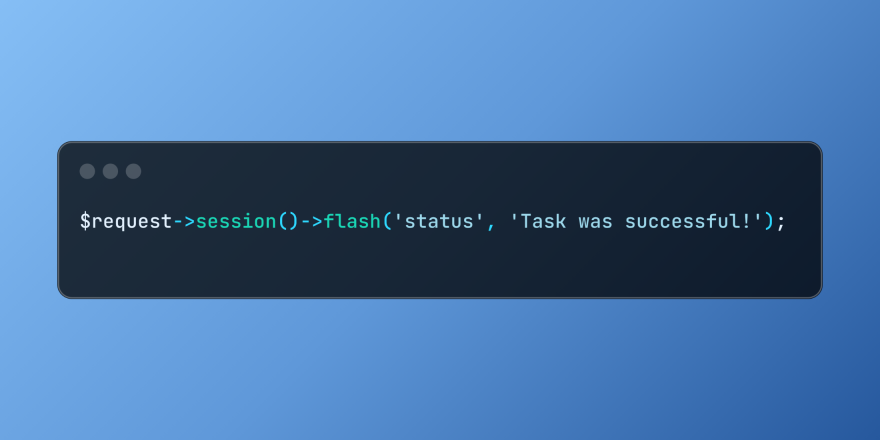
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
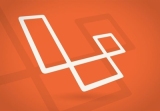
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
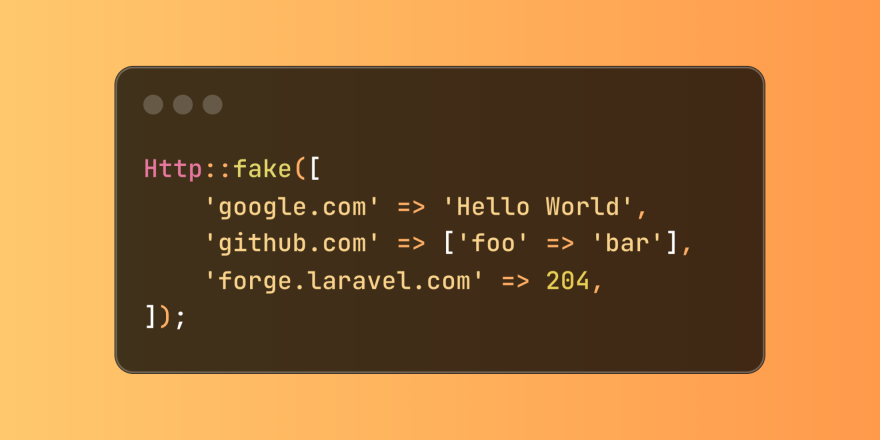
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
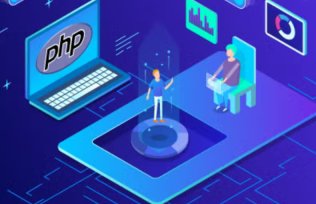
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
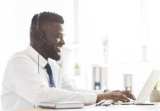
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
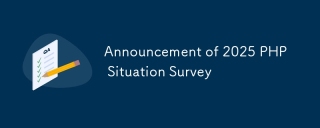
The 2025 PHP Landscape Survey investigates current PHP development trends. It explores framework usage, deployment methods, and challenges, aiming to provide insights for developers and businesses. The survey anticipates growth in modern PHP versio
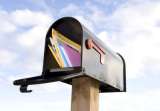
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
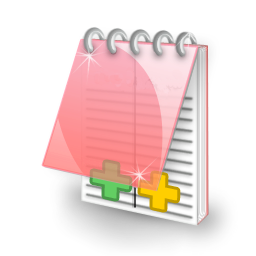
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SublimeText3 Chinese version
Chinese version, very easy to use
