This tutorial explores two approaches to form handling in Symfony 2. The first demonstrates embedding form elements directly within the view and manually processing them in the controller. The second utilizes Symfony's form system for object-oriented form declaration and automated processing and persistence.
We'll use Symfony's default AcmeDemoBundle for illustration. The complete code is available in [this repository](repository_link_here - replace with actual link if available).
Key Concepts:
- Symfony 2 offers two primary methods for form creation and handling: manual HTML embedding in the view with controller-based processing, and Symfony's object-oriented form system.
- Non-entity forms are created by adding standard HTML form elements to a Symfony view file. This involves a view file containing the form HTML, a controller method for logic handling (rendering, processing), and a route mapping a URL to the controller method.
- Symfony Entities and Forms offer a more efficient approach. This involves creating a form for a Symfony entity, using the Symfony form builder, and defining methods for form construction, naming, and default options. These forms are built and displayed via controller methods.
- Symfony's form system is flexible, adapting to various needs. For database-related CRUD operations, the Symfony form system is preferred. For simple, non-persistent forms, direct HTML embedding in the view is sufficient.
Non-Entity Forms:
This example demonstrates processing forms defined as standard HTML within a Symfony view file. The process involves three steps:
-
View File Creation: A
form1.html.twig
file (located insrc/Acme/DemoBundle/Resources/views/Welcome
) contains the form's HTML:
{% extends "AcmeDemoBundle::layout.html.twig" %} {% block content %} <h1 id="Form-values">Form values</h1> {% if name is defined %} <p>Name: {{ name }} </p> {% endif %} <form method="post" action="{{ path('_form1') }}"> <label for="name">Name:</label> <input type="text" id="name" name="name" /><br> <input type="submit" name="submit" value="Submit" /> </form> {% endblock %}
-
Controller Method: The
WelcomeController
includes aform1Action
method:
use Symfony\Component\HttpFoundation\Request; // ... other code ... public function form1Action(Request $request) { $name = 'Not submitted yet'; if ($request->isMethod('POST') && $request->request->has('submit')) { $name = $request->request->get('name'); } return $this->render('AcmeDemoBundle:Welcome:form1.html.twig', ['name' => $name]); }
-
Route Definition: The
routing.yml
file (insrc/Acme/DemoBundle/Resources/config
) includes:
_form1: path: /form1 defaults: { _controller: AcmeDemoBundle:Welcome:form1 }
This maps /form1
to the form1Action
method.
This approach, while functional, is less suitable for complex forms or data persistence.
Symfony Entities and Forms:
This section illustrates creating and processing a form for a Symfony entity (e.g., Article
). We'll use five files: the controller, routing.yml
, two view files (form2.html.twig
, form2saved.html.twig
), and an ArticleType.php
form type class.
-
ArticleType.php: (Located in
src/Acme/DemoBundle/Form
)
{% extends "AcmeDemoBundle::layout.html.twig" %} {% block content %} <h1 id="Form-values">Form values</h1> {% if name is defined %} <p>Name: {{ name }} </p> {% endif %} <form method="post" action="{{ path('_form1') }}"> <label for="name">Name:</label> <input type="text" id="name" name="name" /><br> <input type="submit" name="submit" value="Submit" /> </form> {% endblock %}
- WelcomeController:
use Symfony\Component\HttpFoundation\Request; // ... other code ... public function form1Action(Request $request) { $name = 'Not submitted yet'; if ($request->isMethod('POST') && $request->request->has('submit')) { $name = $request->request->get('name'); } return $this->render('AcmeDemoBundle:Welcome:form1.html.twig', ['name' => $name]); }
- form2.html.twig:
_form1: path: /form1 defaults: { _controller: AcmeDemoBundle:Welcome:form1 }
- form2saved.html.twig:
<?php namespace Acme\DemoBundle\Form; use Symfony\Component\Form\AbstractType; use Symfony\Component\Form\FormBuilderInterface; use Symfony\Component\OptionsResolver\OptionsResolver; class ArticleType extends AbstractType { public function buildForm(FormBuilderInterface $builder, array $options) { $builder ->add('title', 'text', ['label' => 'Title']) ->add('body', 'textarea') ->add('save', 'submit') ; } public function configureOptions(OptionsResolver $resolver) { $resolver->setDefaults(['data_class' => 'Acme\DemoBundle\Entity\Article']); } public function getName() { return 'article'; } }
- routing.yml:
// ... other uses ... use Acme\DemoBundle\Entity\Article; use Acme\DemoBundle\Form\ArticleType; // ... other methods ... public function form2Action(Request $request) { $article = new Article(); $form = $this->createForm(ArticleType::class, $article); $form->handleRequest($request); if ($form->isSubmitted() && $form->isValid()) { $em = $this->getDoctrine()->getManager(); $em->persist($article); $em->flush(); $this->addFlash('message', 'Article saved!'); return $this->redirectToRoute('_form2saved'); } return $this->render('AcmeDemoBundle:Welcome:form2.html.twig', ['form' => $form->createView()]); } public function form2savedAction() { return $this->render('AcmeDemoBundle:Welcome:form2saved.html.twig'); }
This approach leverages Symfony's features for a more robust and maintainable solution. Choose the method best suited to your form's complexity and data handling needs. For complex forms or database interactions, the entity/form approach is strongly recommended.
The above is the detailed content of Building and Processing Forms in Symfony 2. For more information, please follow other related articles on the PHP Chinese website!
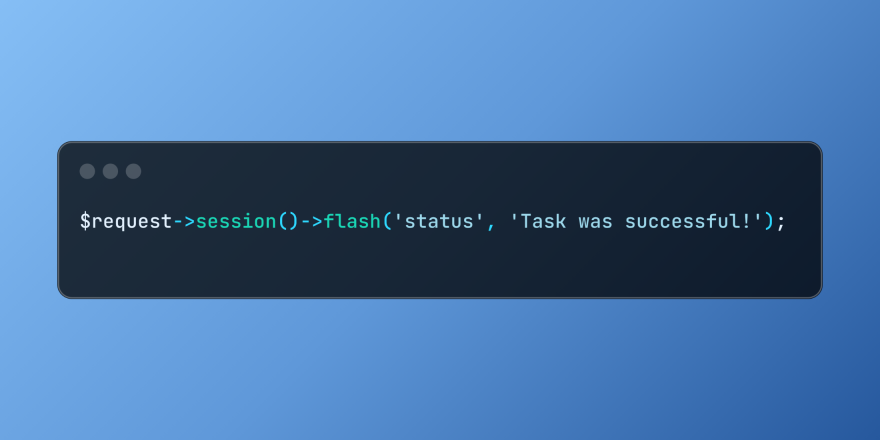
Laravel simplifies handling temporary session data using its intuitive flash methods. This is perfect for displaying brief messages, alerts, or notifications within your application. Data persists only for the subsequent request by default: $request-
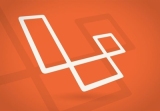
This is the second and final part of the series on building a React application with a Laravel back-end. In the first part of the series, we created a RESTful API using Laravel for a basic product-listing application. In this tutorial, we will be dev
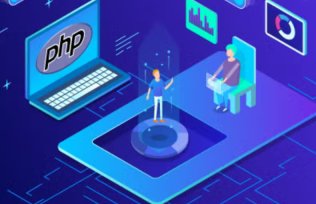
The PHP Client URL (cURL) extension is a powerful tool for developers, enabling seamless interaction with remote servers and REST APIs. By leveraging libcurl, a well-respected multi-protocol file transfer library, PHP cURL facilitates efficient execution of various network protocols, including HTTP, HTTPS, and FTP. This extension offers granular control over HTTP requests, supports multiple concurrent operations, and provides built-in security features.
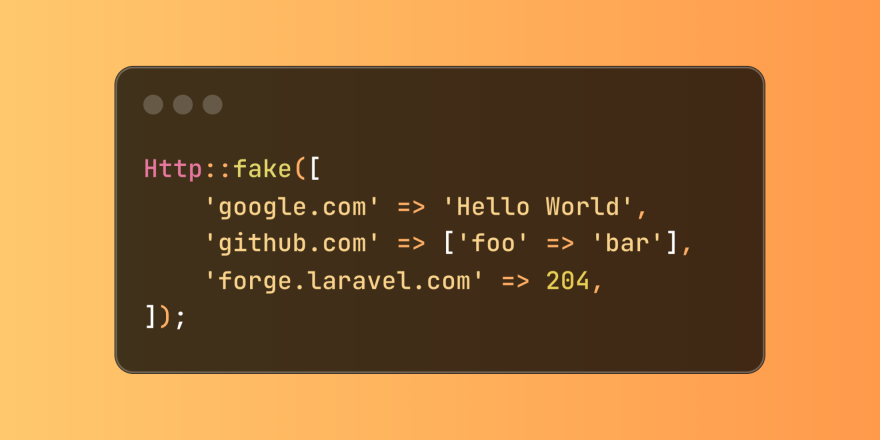
Laravel provides concise HTTP response simulation syntax, simplifying HTTP interaction testing. This approach significantly reduces code redundancy while making your test simulation more intuitive. The basic implementation provides a variety of response type shortcuts: use Illuminate\Support\Facades\Http; Http::fake([ 'google.com' => 'Hello World', 'github.com' => ['foo' => 'bar'], 'forge.laravel.com' =>
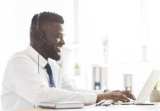
Do you want to provide real-time, instant solutions to your customers' most pressing problems? Live chat lets you have real-time conversations with customers and resolve their problems instantly. It allows you to provide faster service to your custom
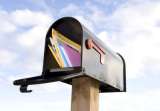
In this article, we're going to explore the notification system in the Laravel web framework. The notification system in Laravel allows you to send notifications to users over different channels. Today, we'll discuss how you can send notifications ov
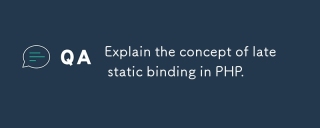
Article discusses late static binding (LSB) in PHP, introduced in PHP 5.3, allowing runtime resolution of static method calls for more flexible inheritance.Main issue: LSB vs. traditional polymorphism; LSB's practical applications and potential perfo
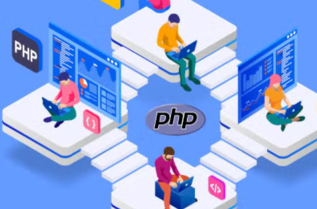
PHP logging is essential for monitoring and debugging web applications, as well as capturing critical events, errors, and runtime behavior. It provides valuable insights into system performance, helps identify issues, and supports faster troubleshoot


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
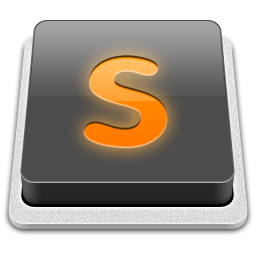
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

Atom editor mac version download
The most popular open source editor

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
