This article benefited from peer review by Matt Smith and Tim Severien. Thanks to SitePoint's peer reviewers for enhancing content quality!
Selecting elements is fundamental to manipulating web pages with jQuery. Whether you're modifying content, attaching events, or performing other actions, you'll need to target the right elements first. This tutorial explores jQuery selectors, a key component of the library.
Key Concepts
- jQuery selectors are essential for targeting webpage elements for content manipulation, event attachment, and more. These selectors utilize criteria such as IDs, classes, attributes, or combinations thereof.
- jQuery provides a wide array of selectors, including basic, index-based, child, attribute, content, hierarchy, form, and visibility selectors. Each type offers unique selection capabilities based on specific conditions and parameters.
- Enhancements in jQuery 3 significantly improved the speed of custom selectors like
:visible
and:hidden
(up to 17x faster in some cases). Visibility is now determined by the presence of a layout box fromgetClientRects
. - Caching selected elements boosts performance, especially with numerous selectors. Storing selections in variables reduces redundant DOM scans.
- While many jQuery selectors mirror CSS selectors, jQuery also includes its own custom selectors for more concise and efficient element selection.
jQuery Selectors in Detail
jQuery selectors primarily identify elements based on criteria like ID, class, attributes, or combinations. Many are based on CSS selectors, but jQuery extends functionality with custom selectors.
Basic Selectors
Select elements by ID ($("#id")
), class ($(".class")
), or tag name ($("li")
). Combine these (e.g., $(".class tag")
) or use multiple selectors separated by commas (e.g., $("selectorA, selectorB, selectorC")
).
Additional basic selectors:
-
:header
: Selects all headings (<h1></h1>
to<h6></h6>
). More concise than individually listing each heading tag. -
:target
: Selects the element whose ID matches the URL fragment identifier (e.g.,https://example.com/#myElement
). -
:animated
: Selects elements currently undergoing animation (requires the jQuery effects module).
Index-Based Selectors
jQuery offers zero-based index selectors:
-
:eq(n)
: Selects the element at indexn
(supports positive and negative indices). -
:lt(n)
: Selects elements with an index less thann
. -
:gt(n)
: Selects elements with an index greater than or equal ton
. -
:first
: Selects the first matched element. -
:last
: Selects the last matched element. -
:even
: Selects elements with even indices (0, 2, 4...). -
:odd
: Selects elements with odd indices (1, 3, 5...).
Interactive demo showcasing index-based selectors
Child Selectors
These selectors target children based on index or type:
-
:first-child
: Selects the first child of each parent. -
:first-of-type
: Selects the first sibling of its type. -
:last-child
: Selects the last child of each parent. -
:last-of-type
: Selects the last sibling of its type. -
:nth-child(n)
: Selects the nth child (supports various expressions like numbers,even
,odd
, formulas). -
:nth-last-child(n)
: Similar to:nth-child
, but counts from the last child. -
:nth-of-type(n)
: Selects the nth sibling of its type. -
:nth-last-of-type(n)
: Similar to:nth-of-type
, but counts from the last sibling. -
:only-child
: Selects elements that are the only child of their parent. -
:only-of-type
: Selects elements with no siblings of the same type.
Interactive demo showcasing child selectors
Attribute Selectors
Select elements based on attribute values:
-
[attribute="value"]
: Selects elements with the exact attribute value. -
[attribute^="value"]
: Selects elements whose attribute value begins with "value". -
[attribute$="value"]
: Selects elements whose attribute value ends with "value". -
[attribute*="value"]
: Selects elements whose attribute value contains "value". -
[attribute|="value"]
: Selects elements whose attribute value is equal to or starts with "value" followed by a hyphen. -
[attribute~="value"]
: Selects elements whose attribute value contains "value" as a space-separated word. -
[attribute!="value"]
: Selects elements without the attribute or with a different value. -
[attribute]
: Selects elements with the specified attribute, regardless of value.
Content Selectors
These selectors target elements based on their content:
-
:contains(text)
: Selects elements containing the specified text (case-sensitive). -
:has(selector)
: Selects elements containing at least one element matching the provided selector. -
:empty
: Selects elements with no children. -
:parent
: Selects elements with at least one child.
Hierarchy Selectors
These selectors use the DOM hierarchy:
-
ancestor descendant
: Selects all descendants of the ancestor element. -
parent > child
: Selects direct children of the parent element. -
prev next
: Selects the next sibling of theprev
element. -
prev ~ siblings
: Selects all subsequent siblings of theprev
element.
Form Selectors
Simplified selectors for form elements:
-
:button
: Selects button elements. -
:checkbox
: Selects checkbox elements. -
:radio
: Selects radio button elements. -
:text
: Selects text input elements. -
:password
: Selects password input elements. -
:submit
: Selects submit button elements. -
:reset
: Selects reset button elements. -
:image
: Selects image button elements. -
:file
: Selects file input elements. -
:hidden
: Selects hidden form elements. -
:enabled
: Selects enabled form elements. -
:disabled
: Selects disabled form elements. -
:checked
: Selects checked checkboxes and radio buttons, and selected options. -
:selected
: Selects selected options in<select></select>
elements.
Visibility Selectors
-
:visible
: Selects visible elements. -
:hidden
: Selects hidden elements.
jQuery 3 Changes
jQuery 3 introduced performance improvements for :visible
and :hidden
, and refined the definition of visibility. Error handling for invalid selectors was also enhanced.
Caching for Performance
Caching selected elements improves performance by avoiding repeated DOM scans. Store selections in variables for reuse.
Conclusion
This tutorial comprehensively covers jQuery selectors. Remember to utilize caching for optimal performance. Understanding these selectors is crucial for effective jQuery development.
Frequently Asked Questions (FAQs)
The FAQs section from the original input is already well-structured and comprehensive. I would suggest keeping it as is, perhaps with minor wording adjustments for improved flow and clarity.
The above is the detailed content of A Comprehensive Look at jQuery Selectors. For more information, please follow other related articles on the PHP Chinese website!
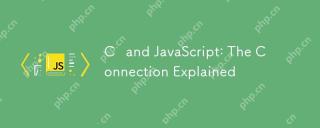
C and JavaScript achieve interoperability through WebAssembly. 1) C code is compiled into WebAssembly module and introduced into JavaScript environment to enhance computing power. 2) In game development, C handles physics engines and graphics rendering, and JavaScript is responsible for game logic and user interface.
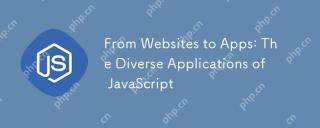
JavaScript is widely used in websites, mobile applications, desktop applications and server-side programming. 1) In website development, JavaScript operates DOM together with HTML and CSS to achieve dynamic effects and supports frameworks such as jQuery and React. 2) Through ReactNative and Ionic, JavaScript is used to develop cross-platform mobile applications. 3) The Electron framework enables JavaScript to build desktop applications. 4) Node.js allows JavaScript to run on the server side and supports high concurrent requests.
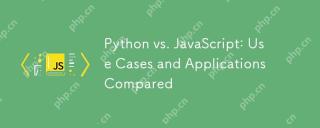
Python is more suitable for data science and automation, while JavaScript is more suitable for front-end and full-stack development. 1. Python performs well in data science and machine learning, using libraries such as NumPy and Pandas for data processing and modeling. 2. Python is concise and efficient in automation and scripting. 3. JavaScript is indispensable in front-end development and is used to build dynamic web pages and single-page applications. 4. JavaScript plays a role in back-end development through Node.js and supports full-stack development.
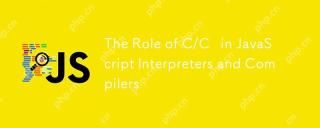
C and C play a vital role in the JavaScript engine, mainly used to implement interpreters and JIT compilers. 1) C is used to parse JavaScript source code and generate an abstract syntax tree. 2) C is responsible for generating and executing bytecode. 3) C implements the JIT compiler, optimizes and compiles hot-spot code at runtime, and significantly improves the execution efficiency of JavaScript.
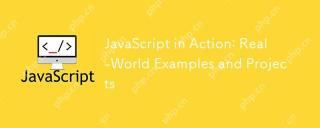
JavaScript's application in the real world includes front-end and back-end development. 1) Display front-end applications by building a TODO list application, involving DOM operations and event processing. 2) Build RESTfulAPI through Node.js and Express to demonstrate back-end applications.
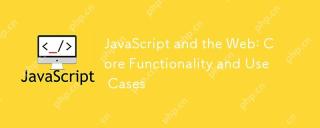
The main uses of JavaScript in web development include client interaction, form verification and asynchronous communication. 1) Dynamic content update and user interaction through DOM operations; 2) Client verification is carried out before the user submits data to improve the user experience; 3) Refreshless communication with the server is achieved through AJAX technology.
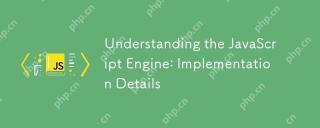
Understanding how JavaScript engine works internally is important to developers because it helps write more efficient code and understand performance bottlenecks and optimization strategies. 1) The engine's workflow includes three stages: parsing, compiling and execution; 2) During the execution process, the engine will perform dynamic optimization, such as inline cache and hidden classes; 3) Best practices include avoiding global variables, optimizing loops, using const and lets, and avoiding excessive use of closures.

Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Atom editor mac version download
The most popular open source editor
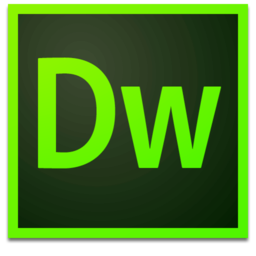
Dreamweaver Mac version
Visual web development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
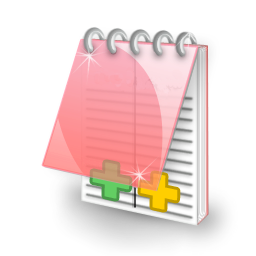
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function