This article examines the challenges of using random number generation in cryptography, highlighting the differences between PHP 5 and PHP 7. PHP 5 lacks readily available mechanisms for generating cryptographically secure random numbers, while PHP 7 introduces random_bytes
and random_int
functions for this purpose.
Understanding CSPRNGs
A Cryptographically Secure Pseudorandom Number Generator (CSPRNG) is a PRNG designed for cryptographic applications. Its key characteristic is high-quality randomness, crucial for:
- Key generation
- Random password creation
- Encryption algorithms
CSPRNGs in PHP 7
PHP 7 provides random_bytes
(returning a string of specified byte length) and random_int
(returning a random integer within a given range) for CSPRNG functionality.
random_bytes
example:
$bytes = random_bytes(10); var_dump(bin2hex($bytes)); // Possible output: string(20) "7dfab0af960d359388e6"
random_int
example:
var_dump(random_int(1, 100)); // Possible output: 27
These functions utilize various sources of randomness depending on the operating system, prioritizing secure options like CryptGenRandom
(Windows), arc4random_buf
(BSD), getrandom(2)
(Linux), and finally /dev/urandom
as a fallback. An error is thrown if no suitable source is found.
Testing Randomness
Evaluating the quality of a random number generator involves statistical tests. A simple example is simulating dice rolls. The expected distribution of outcomes for rolling three dice 1,000,000 times can be compared to the actual results generated by random_int
and the standard rand
function.
A code example (simplified for brevity) and a comparative graph (shown below) demonstrate that random_int
exhibits a distribution closer to the expected values, indicating superior randomness compared to rand
.
PHP 5 Alternatives
PHP 5 lacks built-in CSPRNGs. Workarounds include openssl_random_pseudo_bytes()
, mcrypt_create_iv()
, or direct access to /dev/random
or /dev/urandom
. Libraries like RandomLib or libsodium offer additional solutions.
The random_compat
Library
For PHP 5 compatibility, the Paragon Initiative Enterprises random_compat
library provides random_bytes
and random_int
functionality. It can be installed via Composer (composer require paragonie/random_compat
) and used as follows:
$bytes = random_bytes(10); var_dump(bin2hex($bytes)); // Possible output: string(20) "7dfab0af960d359388e6"
This library prioritizes different randomness sources compared to PHP 7, starting with /dev/urandom
.
A simple password generation example using random_compat
:
var_dump(random_int(1, 100)); // Possible output: 27
Conclusion
Using a CSPRNG is crucial for secure applications. The random_compat
library offers a backward-compatible solution for PHP 5, while PHP 7 developers should directly utilize random_bytes
and random_int
. Prioritizing reliable random number generation significantly enhances application security.
Further Reading
Description | Link |
---|---|
Die Hard Test | https://www.php.cn/link/1852a2083dbe1c2ec33ab9366feb2862 |
Chi-square test with dice example | https://www.php.cn/link/fb6a253729096c1e92e43c26a6fdadc3 |
Kolmogorov-Smirnov Test | https://www.php.cn/link/030d13e49bb7d1add5ac5ea2e4a43231 |
Spectral Test | https://www.php.cn/link/6bbd80b04535d39be5e02dbfd8730469 |
RaBiGeTe test suite | https://www.php.cn/link/e626afbcdb83368b3491c0c473da19f1 |
Random Number Generation In PHP (2011) | https://www.php.cn/link/f7ff233e4ed3e6b13c5d5c7a9201e4ec |
Testing RNG part 1 and 2 | https://www.php.cn/link/a1c71b134d46d7f7ff00f488874a8d43, https://www.php.cn/link/2e3517ba49c2a7c999b9c8381185ae4e |
Acknowledgements
Thanks to Scott Arciszewski for their peer review assistance.
The above is the detailed content of Randomness in PHP - Do You Feel Lucky?. For more information, please follow other related articles on the PHP Chinese website!
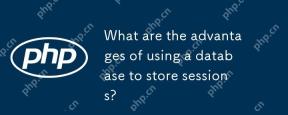
The main advantages of using database storage sessions include persistence, scalability, and security. 1. Persistence: Even if the server restarts, the session data can remain unchanged. 2. Scalability: Applicable to distributed systems, ensuring that session data is synchronized between multiple servers. 3. Security: The database provides encrypted storage to protect sensitive information.
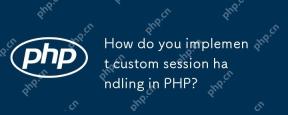
Implementing custom session processing in PHP can be done by implementing the SessionHandlerInterface interface. The specific steps include: 1) Creating a class that implements SessionHandlerInterface, such as CustomSessionHandler; 2) Rewriting methods in the interface (such as open, close, read, write, destroy, gc) to define the life cycle and storage method of session data; 3) Register a custom session processor in a PHP script and start the session. This allows data to be stored in media such as MySQL and Redis to improve performance, security and scalability.
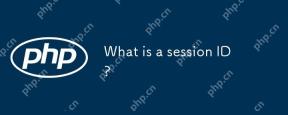
SessionID is a mechanism used in web applications to track user session status. 1. It is a randomly generated string used to maintain user's identity information during multiple interactions between the user and the server. 2. The server generates and sends it to the client through cookies or URL parameters to help identify and associate these requests in multiple requests of the user. 3. Generation usually uses random algorithms to ensure uniqueness and unpredictability. 4. In actual development, in-memory databases such as Redis can be used to store session data to improve performance and security.
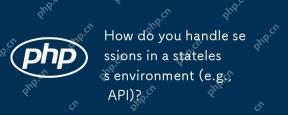
Managing sessions in stateless environments such as APIs can be achieved by using JWT or cookies. 1. JWT is suitable for statelessness and scalability, but it is large in size when it comes to big data. 2.Cookies are more traditional and easy to implement, but they need to be configured with caution to ensure security.
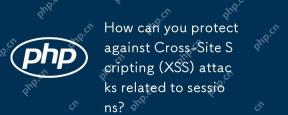
To protect the application from session-related XSS attacks, the following measures are required: 1. Set the HttpOnly and Secure flags to protect the session cookies. 2. Export codes for all user inputs. 3. Implement content security policy (CSP) to limit script sources. Through these policies, session-related XSS attacks can be effectively protected and user data can be ensured.
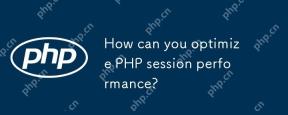
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
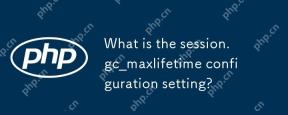
Thesession.gc_maxlifetimesettinginPHPdeterminesthelifespanofsessiondata,setinseconds.1)It'sconfiguredinphp.iniorviaini_set().2)Abalanceisneededtoavoidperformanceissuesandunexpectedlogouts.3)PHP'sgarbagecollectionisprobabilistic,influencedbygc_probabi

In PHP, you can use the session_name() function to configure the session name. The specific steps are as follows: 1. Use the session_name() function to set the session name, such as session_name("my_session"). 2. After setting the session name, call session_start() to start the session. Configuring session names can avoid session data conflicts between multiple applications and enhance security, but pay attention to the uniqueness, security, length and setting timing of session names.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
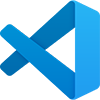
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
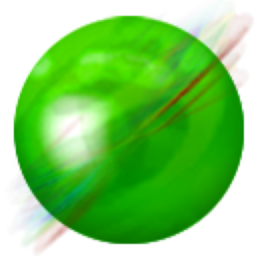
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment