A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be capitalized. or lowercase.
What is a vowel?
Vowels are alphabetic characters representing specific pronunciations. There are five vowels in English, including caps and lowercase:
<code>a, e, i, o, u</code>
Example 1
- Input: String = "Tutorialspoint"
- Output: 6
Explanation
The vowels in the string "Tutorialspoint" areu, o, i, i, a, o, i. There are a total of 6
vowels.Example 2
- Input: String = "PHP"
- Output: 0
Explanation
The string "PHP" does not contain any vowels, so the count is 0.
Example 3
- Input: String = "Ayush Mishra"
- Output: 4
Explanation
The vowels in the string are a, u, i, i, a
. There are a total of4
vowels.- The following are different ways to use PHP to count vowels in strings:
- Use direct logic method
Using function
Use direct logic methods to count vowels in strings
We use a simple loop to iterate over the string and check if each character is a vowel. If so, increment the counter. After the loop ends, we return the total number of vowels.
- Implementation steps
- First, define a string as input parameter.
- Now, initialize the count variable to 0.
- Loop through each character in the string.
- For each character, check if it is a vowel (case insensitive).
- Add count variables for each vowel.
Returns the count of vowels.
<?php $string = "Anshu Ayush"; $vowel_count = 0; // 将字符串转换为小写 $string = strtolower($string); // 循环遍历字符串中的每个字符 for ($i = 0; $i < strlen($string); $i++) { if (in_array($string[$i], ['a', 'e', 'i', 'o', 'u'])) { $vowel_count++; } } // 输出 echo "字符串 '$string' 中元音的数量是:$vowel_count"; ?>
Implementation code
<code>字符串 'anshu ayush' 中元音的数量是:3</code>
Output
Time complexity: O(n)
Space complexity:
O(1)Use function to count vowels in strings In this method, we use a function to calculate the number of vowels in a string. We use a function so that we can use it later as needed.
Implementation steps
- First, create a function that accepts strings as input.
- Now, initialize the count variable to 0 and convert the string to lowercase.
- Transfer through the string and check vowels with a predefined list.
- Add count variables for each vowel.
- Returns the count of vowels.
Implementation code
<code>a, e, i, o, u</code>
Output
<?php $string = "Anshu Ayush"; $vowel_count = 0; // 将字符串转换为小写 $string = strtolower($string); // 循环遍历字符串中的每个字符 for ($i = 0; $i < strlen($string); $i++) { if (in_array($string[$i], ['a', 'e', 'i', 'o', 'u'])) { $vowel_count++; } } // 输出 echo "字符串 '$string' 中元音的数量是:$vowel_count"; ?>
Time complexity: O(n)
Space complexity: O(1)
The above is the detailed content of PHP Program to Count Vowels in a String. For more information, please follow other related articles on the PHP Chinese website!
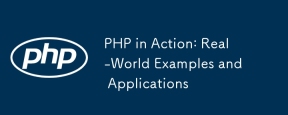
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
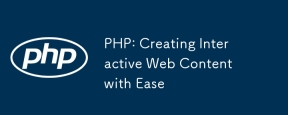
PHP makes it easy to create interactive web content. 1) Dynamically generate content by embedding HTML and display it in real time based on user input or database data. 2) Process form submission and generate dynamic output to ensure that htmlspecialchars is used to prevent XSS. 3) Use MySQL to create a user registration system, and use password_hash and preprocessing statements to enhance security. Mastering these techniques will improve the efficiency of web development.
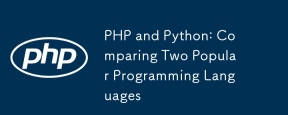
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
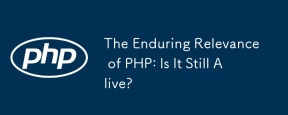
PHP is still dynamic and still occupies an important position in the field of modern programming. 1) PHP's simplicity and powerful community support make it widely used in web development; 2) Its flexibility and stability make it outstanding in handling web forms, database operations and file processing; 3) PHP is constantly evolving and optimizing, suitable for beginners and experienced developers.
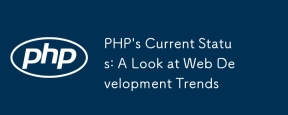
PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
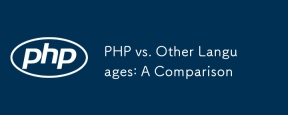
PHP is suitable for web development, especially in rapid development and processing dynamic content, but is not good at data science and enterprise-level applications. Compared with Python, PHP has more advantages in web development, but is not as good as Python in the field of data science; compared with Java, PHP performs worse in enterprise-level applications, but is more flexible in web development; compared with JavaScript, PHP is more concise in back-end development, but is not as good as JavaScript in front-end development.
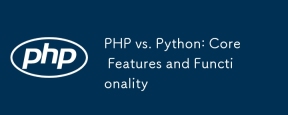
PHP and Python each have their own advantages and are suitable for different scenarios. 1.PHP is suitable for web development and provides built-in web servers and rich function libraries. 2. Python is suitable for data science and machine learning, with concise syntax and a powerful standard library. When choosing, it should be decided based on project requirements.
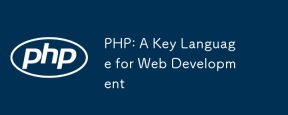
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

Zend Studio 13.0.1
Powerful PHP integrated development environment

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
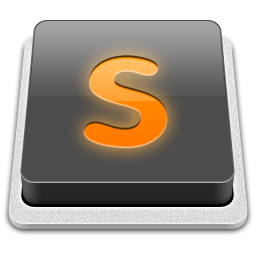
SublimeText3 Mac version
God-level code editing software (SublimeText3)
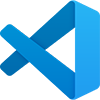
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft