Understanding NullReferenceExceptions in .NET
A NullReferenceException
arises when your code attempts to access a member (property, method, etc.) of a reference variable that currently holds a null
value. This typically happens when a variable hasn't been initialized, or when a method returns null
unexpectedly.
Common Scenarios Leading to NullReferenceExceptions:
- Uninitialized Variables: A variable declared but not assigned a valid object instance.
-
Unexpected Null Returns: A method or property returns
null
when a non-null value was anticipated. -
Chained References: Accessing nested properties (e.g.,
objectA.objectB.propertyC
) where any ofobjectA
orobjectB
might benull
.
Effective Debugging Strategies:
-
Strategic Breakpoints: Pause execution at key points in your code to inspect variable values and identify the source of the
null
reference. - Reference Tracking: Use your IDE's "Find All References" feature to trace how a variable is used throughout your code, helping pinpoint potential issues.
Proactive Prevention Techniques:
1. Explicit Null Checks:
Before accessing members of an object, always check if it's null
:
void PrintName(Person p) { if (p != null) Console.WriteLine(p.Name); }
2. Default Values:
Provide a default value in case a reference might be null
:
string GetCategory(Book b) { return b?.Category ?? "Unknown"; //Null-conditional operator and null-coalescing operator }
3. Custom Exceptions:
Handle potential null
values by throwing a more informative custom exception:
string GetCategory(string bookTitle) { var book = library.FindBook(bookTitle); if (book == null) throw new BookNotFoundException(bookTitle); return book.Category; }
4. Null-Conditional Operator (?.
) and Null-Conditional Member Access (?[]
):
These operators provide concise null checks:
var title = person?.Title?.ToUpper(); //Only executes if person and Title are not null. int[] myIntArray = null; var elem = myIntArray?[i]; //Only accesses index i if myIntArray is not null.
5. Null Context (C# 8 and later):
Leverage the null context feature to enforce stricter null checks at compile time.
Additional Considerations:
-
LINQ Queries: Be mindful of
FirstOrDefault()
andSingleOrDefault()
, which can returnnull
. -
Nullable Value Types: Use the
GetValueOrDefault()
method for nullable value types. - Events: Ensure event handlers are properly attached and detached.
- ASP.NET: Pay attention to the page lifecycle and session management.
By consistently applying these strategies, you can significantly reduce the occurrence of NullReferenceExceptions
and create more robust and reliable .NET applications.
The above is the detailed content of What Causes NullReferenceExceptions and How Can They Be Avoided in .NET?. For more information, please follow other related articles on the PHP Chinese website!
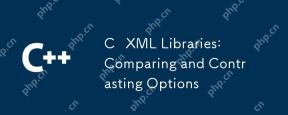
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
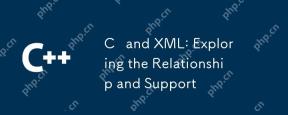
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
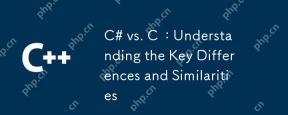
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
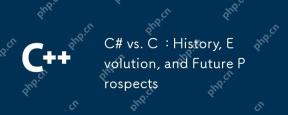
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
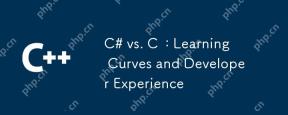
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
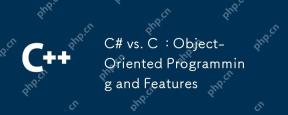
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
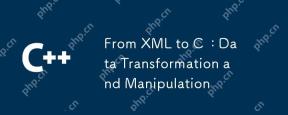
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
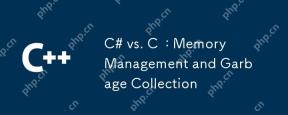
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
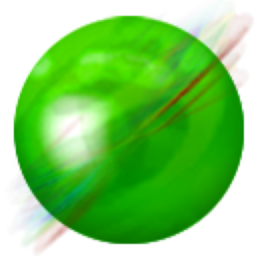
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
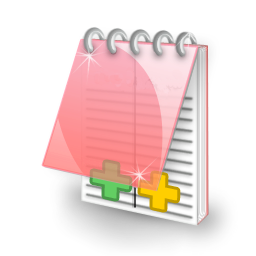
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

SublimeText3 Chinese version
Chinese version, very easy to use

Notepad++7.3.1
Easy-to-use and free code editor