


Application scenarios:
Supports multiple sorting methods:
- Ascending or descending order:
https://your-url?sort[first_name]=desc&sort[last_name]=asc
- Use ascending or descending:
https://your-url?sort[first_name]=ascending&sort[last_name]=descending
- Use 1 or -1:
https://your-url?sort[first_name]=1&sort[last_name]=-1
This function also supports sorting using the sortBy
and sortOrder
fields: https://your-url?sortOrder=desc&sortBy=last_name
TypeScript code:
type ISortOrder = "asc" | "desc" | "ascending" | "descending" | 1 | -1; export interface IPaginationFields { page?: number; limit?: number; sortBy?: string | string[]; sortOrder?: ISortOrder | ISortOrder[]; sort?: Record<string, ISortOrder>; } export interface IFormatedPagination { skip: number; page: number; limit: number; sort: { [key: string]: 1 | -1 }; } export const formatPagination = (pagination: IPaginationFields): IFormatedPagination => { const { limit = 10, page = 1, sortBy, sortOrder, sort } = pagination; const formattedSort: { [key: string]: 1 | -1 } = {}; const normalizeOrder = (order: string | number): 1 | -1 => { const numOrder = Number(order); if (!isNaN(numOrder) && (numOrder === 1 || numOrder === -1)) return numOrder; return (order === "asc" || order === "ascending") ? 1 : -1; }; if (sortBy) { const sortByArray = Array.isArray(sortBy) ? sortBy : [sortBy]; const sortOrderArray = Array.isArray(sortOrder) ? sortOrder : [sortOrder]; sortByArray.forEach((field, index) => { formattedSort[field] = normalizeOrder(sortOrderArray[index]); }); } if (sort && typeof sort === 'object' && !Array.isArray(sort)) { Object.entries(sort).forEach(([field, order]) => { formattedSort[field] = normalizeOrder(order); }); } if (!formattedSort.createdAt) { formattedSort.createdAt = -1; } return { skip: (page - 1) * limit, limit: limit, page: page, sort: formattedSort, }; };
JavaScript code:
const formatPagination = (pagination) => { const { limit = 10, page = 1, sortBy, sortOrder, sort } = pagination; const formattedSort = {}; const normalizeOrder = (order) => { const numOrder = Number(order); if (!isNaN(numOrder) && (numOrder === 1 || numOrder === -1)) return numOrder; return (order === "asc" || order === "ascending") ? 1 : -1; }; if (sortBy) { const sortByArray = Array.isArray(sortBy) ? sortBy : [sortBy]; const sortOrderArray = Array.isArray(sortOrder) ? sortOrder : [sortOrder]; sortByArray.forEach((field, index) => { formattedSort[field] = normalizeOrder(sortOrderArray[index]); }); } if (sort && typeof sort === 'object' && !Array.isArray(sort)) { Object.entries(sort).forEach(([field, order]) => { formattedSort[field] = normalizeOrder(order); }); } if (!formattedSort.createdAt) { formattedSort.createdAt = -1; } return { skip: (page - 1) * limit, limit: limit, page: page, sort: formattedSort, }; };
Overview
This code defines an interface to the paging and sorting fields, as well as a utility function for formatting these fields into a structure suitable for database queries or other paging use cases. This utility helps standardize the paging and sorting process.
Code explanation
Interface
ISortOrder
: Possible values indicating the sort order: "asc", "desc", "ascending", "descending", 1, -1.
IPaginationFields
: Input structure describing paging and sorting: page
(optional), limit
(optional), sortBy
(optional), sortOrder
(optional), sort
(optional).
IFormatedPagination
: Describes the output structure of formatted pagination: skip
, page
, limit
, sort
.
formatPagination
Function
This function processes the input pagination object and converts it into a standardized format.
Parameters
-
pagination
: Object that implements theIPaginationFields
interface.
Steps
-
Default value: Assign default values to
limit
(10) andpage
(1). Initialize an empty objectformattedSort
to store the formatted sort field. -
Helper functions:
normalizeOrder
: Convert the given sort order (value) to numeric format (1 or -1). -
handles
sortBy
andsortOrder
: handles the case wheresortBy
andsortOrder
are arrays or single values, convert them to arrays (if they are not already), and usenormalizeOrder
Add them toformattedSort
. -
handles
sort
objects : ifsort
is an object (not an array), iterate over its keys, usenormalizeOrder
to convert the values of each key into numerical order, and Add it toformattedSort
. -
Default sort field: If
formattedSort
does not contain thecreatedAt
field, add it and set its sort order to descending (-1). -
Return result: Returns an object containing the following properties:
skip
,limit
,page
,sort
.
type ISortOrder = "asc" | "desc" | "ascending" | "descending" | 1 | -1; export interface IPaginationFields { page?: number; limit?: number; sortBy?: string | string[]; sortOrder?: ISortOrder | ISortOrder[]; sort?: Record<string, ISortOrder>; } export interface IFormatedPagination { skip: number; page: number; limit: number; sort: { [key: string]: 1 | -1 }; } export const formatPagination = (pagination: IPaginationFields): IFormatedPagination => { const { limit = 10, page = 1, sortBy, sortOrder, sort } = pagination; const formattedSort: { [key: string]: 1 | -1 } = {}; const normalizeOrder = (order: string | number): 1 | -1 => { const numOrder = Number(order); if (!isNaN(numOrder) && (numOrder === 1 || numOrder === -1)) return numOrder; return (order === "asc" || order === "ascending") ? 1 : -1; }; if (sortBy) { const sortByArray = Array.isArray(sortBy) ? sortBy : [sortBy]; const sortOrderArray = Array.isArray(sortOrder) ? sortOrder : [sortOrder]; sortByArray.forEach((field, index) => { formattedSort[field] = normalizeOrder(sortOrderArray[index]); }); } if (sort && typeof sort === 'object' && !Array.isArray(sort)) { Object.entries(sort).forEach(([field, order]) => { formattedSort[field] = normalizeOrder(order); }); } if (!formattedSort.createdAt) { formattedSort.createdAt = -1; } return { skip: (page - 1) * limit, limit: limit, page: page, sort: formattedSort, }; };
Main functions
- Flexible input: handle single and array values of
sortBy
andsortOrder
. - Default: Ensures pagination works properly even with incomplete input.
- Default sort: Add a fallback sort field (
createdAt
). - Custom sorting: Supports
sort
objects to handle complex sorting scenarios. This utility is ideal for applications that require standardized paging and sorting, such as REST APIs or database queries.
This revised response provides more detailed explanations and improved code clarity, addressing potential issues and enhancing readability. The code is also formatted for better visual presentation.
The above is the detailed content of Multiple fields sorting with a time saving function for server side developer (api pagination). For more information, please follow other related articles on the PHP Chinese website!
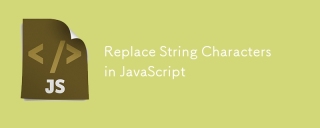
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
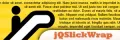
Leverage jQuery for Effortless Web Page Layouts: 8 Essential Plugins jQuery simplifies web page layout significantly. This article highlights eight powerful jQuery plugins that streamline the process, particularly useful for manual website creation
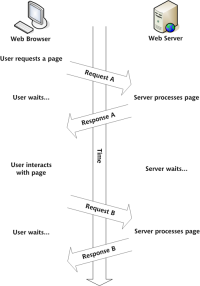
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
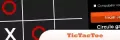
10 fun jQuery game plugins to make your website more attractive and enhance user stickiness! While Flash is still the best software for developing casual web games, jQuery can also create surprising effects, and while not comparable to pure action Flash games, in some cases you can also have unexpected fun in your browser. jQuery tic toe game The "Hello world" of game programming now has a jQuery version. Source code jQuery Crazy Word Composition Game This is a fill-in-the-blank game, and it can produce some weird results due to not knowing the context of the word. Source code jQuery mine sweeping game
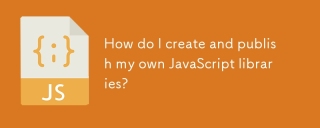
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
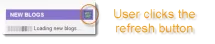
This tutorial demonstrates creating dynamic page boxes loaded via AJAX, enabling instant refresh without full page reloads. It leverages jQuery and JavaScript. Think of it as a custom Facebook-style content box loader. Key Concepts: AJAX and jQuery
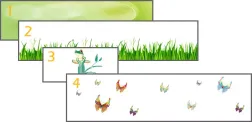
This tutorial demonstrates how to create a captivating parallax background effect using jQuery. We'll build a header banner with layered images that create a stunning visual depth. The updated plugin works with jQuery 1.6.4 and later. Download the
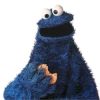
This JavaScript library leverages the window.name property to manage session data without relying on cookies. It offers a robust solution for storing and retrieving session variables across browsers. The library provides three core methods: Session


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use
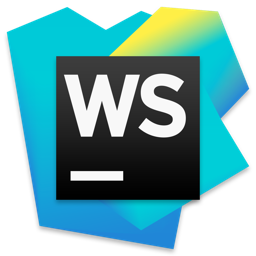
WebStorm Mac version
Useful JavaScript development tools

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.
