The flow control statements of Go language are the basis of its programming. Like other languages, they control the program execution flow and implement decision-making, looping and resource management. This article takes an in-depth look at Go’s flow control statements, including for
, if
, switch
, and defer
, and explains how to use them effectively in Go programs.
This article is part of the Go language tutorial series, designed to help developers understand the Go language more deeply. Whether you are a beginner or an experienced developer, this guide will give you the knowledge you need to write more efficient and readable Go code.
After reading this article, you will master:
- Different types of flow control statements in Go language.
- How to use these statements in real situations.
- Best practices and common pitfalls.
Let’s get started!
Core Concept
1. for
Loop
for
Loop is the only loop structure in Go language, but it is very flexible and can be used in a variety of scenarios:
Basicfor
Loop
for i := 0; i < 10; i++ { fmt.Println(i) }
This is a traditional `for` loop, initializing variables, setting conditions and incrementing variables.
for
Continuous execution of loop (similar to while
loop)
Go does not have the `while` keyword, but you can use a `for` loop to achieve the same effect:
sum := 1 for sum < 100 { sum += sum }
This loop continues to execute until the condition `sum
Infinite loop
If the condition is omitted, the `for` loop will execute infinitely:
for { fmt.Println("无限循环") }
This is useful in tasks that need to run continuously (such as servers).
-
if
Statement
if
Statement`if` statement is used for conditional execution.
Basicif
Statements
if x > 10 { fmt.Println("x大于10") }
if
statement with short statement
A short statement can be executed before the condition:
if x := 5; x < 10 { fmt.Println("x小于10") }
if
and else
You can also use `else` and `else if`:
if x > 10 { fmt.Println("x大于10") } else if x == 10 { fmt.Println("x等于10") } else { fmt.Println("x小于10") }
-
switch
Statement
switch
StatementThe `switch` statement is a powerful way to handle multiple conditions.
Basicswitch
Statements
switch os := runtime.GOOS; os { case "darwin": fmt.Println("OS X") case "linux": fmt.Println("Linux") default: fmt.Printf("%s.\n", os) }
switch
Execution order of statements
Go evaluates the cases of the `switch` statement from top to bottom, stopping once a match is successful.
Unconditionalswitch
Statement
The unconditional `switch` statement is equivalent to `switch true`:
t := time.Now() switch { case t.Hour() < 12: fmt.Println("上午") case t.Hour() < 18: fmt.Println("下午") default: fmt.Println("晚上") }
-
defer
Statement
defer
StatementThe `defer` statement defers the execution of a function until its surrounding function returns.
Basicdefer
Statements
func main() { defer fmt.Println("world") fmt.Println("hello") }
Output:
<code>hello world</code>
Stackeddefer
Statements
Delay functions are executed in last-in-first-out (LIFO) order:
func main() { defer fmt.Println("first") defer fmt.Println("second") defer fmt.Println("third") }
Output:
<code>third second first</code>
Practical example
Let’s look at a practical example demonstrating the use of these flow control statements. We will create a simple program that processes a task list and prints its status.
for i := 0; i < 10; i++ { fmt.Println(i) }
Detailed explanation of steps
- **Task structure**: We define a `Task` structure containing `Name` and `Complete` fields.
- **Task List**: We create a slice of `Task` objects.
- **for loop**: We use a `for` loop to iterate over tasks. For each task, we use an `if` statement to check if it has been completed.
- **switch statement**: We use the `switch` statement to check whether today is a weekend or a working day.
- **defer statement**: We use `defer` to print a message after all tasks are processed.
Best Practices
- **Use for loops wisely**: Since Go only has `for` loops, make sure to use them correctly. Avoid infinite loops unless necessary.
- **Keep if statements simple**: Use short statements in `if` conditions to make the code concise and easy to read.
- **Use switch to handle multiple conditions**: When dealing with multiple conditions, a `switch` statement is more readable than multiple `if-else` statements.
- **Use defer for cleanup**: `defer` is great for resource cleanup, such as closing files or releasing locks.
- **Avoid Deep Nesting**: Deeply nested `if` or `for` statements can make the code difficult to read. Consider refactoring into a function.
Conclusion
Flow control statements are essential tools in the Go language. They allow you to control the execution flow of your program. By mastering `for`, `if`, `switch`, and `defer`, you can write more efficient, readable, and maintainable Go code.
I encourage you to try the examples provided in this article and experiment with the concepts on your own.
Call to Action
This article is part of the Go language tutorial series, designed to help you become a more proficient Go developer. If you found this article helpful, be sure to check out previous and upcoming tutorials in this series. Check it out on my blog or Dev.to.
Happy programming! ?
The above is the detailed content of Flow Control Statements in Go. For more information, please follow other related articles on the PHP Chinese website!
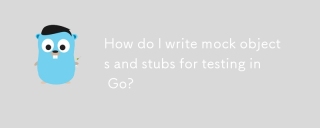
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
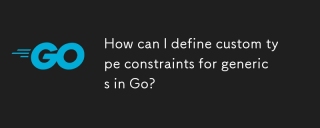
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
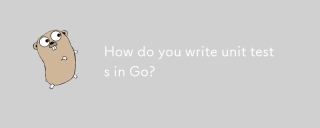
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
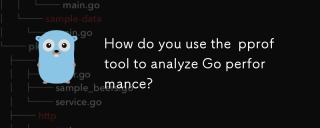
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
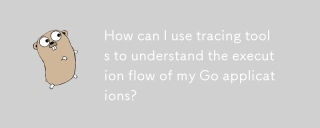
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
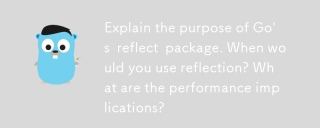
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
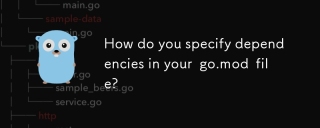
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
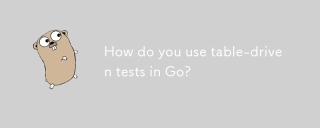
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
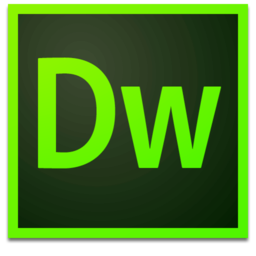
Dreamweaver Mac version
Visual web development tools

Atom editor mac version download
The most popular open source editor
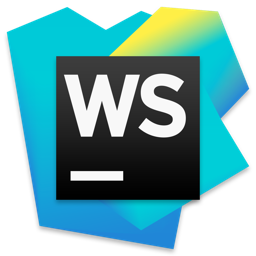
WebStorm Mac version
Useful JavaScript development tools
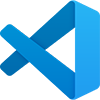
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

Notepad++7.3.1
Easy-to-use and free code editor
