


Feature flags, also known as feature toggles, are a powerful software development technique enabling dynamic feature activation or deactivation. This decoupling of feature deployment from code releases offers superior application control and mitigates new feature rollout risks.
Advantages of Feature Flags:
- Controlled Releases: Introduce new features incrementally to user subsets.
- A/B Testing: Compare feature variations to optimize user experience.
- Swift Rollbacks: Disable malfunctioning features instantly without complete redeployment.
- Continuous Deployment: Safely deploy code, even with incomplete features.
Feature Flag Mechanics:
Feature flags employ conditional logic within your application code. Here's a simplified implementation:
Step 1: Define a Feature Flag
- Choose a descriptive name (e.g.,
new-feature
). - Determine the flag's scope: global, user-specific, or environment-specific.
Step 2: Implement Conditional Logic
Integrate conditional logic to check the feature flag's status before executing the feature:
if (featureFlagService.isEnabled("new-feature")) { // New feature logic } else { // Fallback logic }
Step 3: Store and Manage Flags
Utilize one of these methods for feature flag storage:
- Configuration Files: Employ application properties or YAML files.
- Remote Service: Leverage a feature flag management tool like Unleash (recommended).
- Database: Store flags in a database for runtime updates.
Step 4: Manage Flag States
Dynamically update the flag's state (enabled/disabled) using your chosen storage method or management tool.
Step 5: Runtime Evaluation
The application dynamically checks the flag's state during execution, activating or deactivating features accordingly.
Step 6: Monitor Usage
Employ analytics tools or dashboards (often provided by feature flag services) to track the flag's impact on users and application performance.
Implementing Feature Flags with Spring Boot and Unleash:
This example demonstrates feature flag implementation using Spring Boot and the Unleash platform.
We'll create a Spring Boot service (a simple API) with the Unleash SDK, two feature beans, and an Unleash server to configure and control our flags.
Prerequisites:
- Gradle
- Git
- Docker
- Java IDE (IntelliJ, Eclipse, etc.)
Unleash Setup:
- Clone the Unleash repository:
git clone https://github.com/Unleash/unleash.git
- Navigate to the repository directory:
cd unleash
- Start the Unleash server using Docker:
docker compose up -d
- Access the Unleash server at
http://localhost:4242
(credentials: admin/unleash4all).
Creating a Feature Flag in Unleash:
- Create a new feature flag (e.g.,
featureFlagExample
) within the default project in Unleash. Note that API requests can be used instead of the SDK.
- Activate the flag for the
development
environment.
Generating a Project API Key:
Create an API token in Unleash's Project Settings to authenticate your Spring Boot application.
(Remember to securely store this token!)
Spring Boot Project (Product Discount Example):
This example uses a Spring Boot application managing product discounts based on a feature flag. The Github repository is available here.
(Note: Replace https://www.php.cn/link/
with the actual Github repository link.)
The project's layered architecture includes:
-
SpringUnleashFeatureFlagApplication
: Main application class. -
SpringUnleashFeatureFlagConfiguration
: Configures initial product data. -
ProductController
: REST controller for product access. -
Product
: Product data class. -
ProductRepository
,ProductRepositoryImpl
: Product data access layer. -
ProductService
,ProductServiceImpl
,ProductServiceWithDiscountImpl
: Product service implementations. -
Constant
: Constant values.
Unleash Library Integration:
The build.gradle
file includes the Unleash Spring Boot starter dependency:
if (featureFlagService.isEnabled("new-feature")) { // New feature logic } else { // Fallback logic }
Unleash Configuration in application.yaml
:
Configure the Unleash client in application.yaml
:
dependencies { // ... other dependencies ... implementation 'io.getunleash:springboot-unleash-starter:1.1.0' }
ProductService
Interface with Toggle:
The ProductService
interface uses the @Toggle
annotation to conditionally select the service implementation:
io: getunleash: app-name: spring-demo-flag instance-id: demo-flag-x environment: development api-url: http://localhost:4242/api api-token: <your_api_token>
Service Implementations:
-
ProductServiceImpl
: Returns products without discounts. -
ProductServiceWithDiscountImpl
: Applies discounts to products.
ProductController
:
The ProductController
uses @Qualifier
to inject the appropriate ProductService
implementation:
public interface ProductService { @Toggle(name = "featureFlagExample", alterBean = "productServiceWithDiscountImpl") List<Product> getProducts(); }
Testing:
Test the application with the feature flag enabled and disabled in Unleash to verify the discount logic.
Conclusion:
Feature flags provide a robust mechanism for managing feature deployments. This example showcases how to effectively integrate Unleash with Spring Boot for flexible and controlled feature releases, facilitating A/B testing and rapid rollbacks.
The above is the detailed content of Implementing Feature Flags with Spring: A Step-by-Step Guide for Feature Deployment. For more information, please follow other related articles on the PHP Chinese website!
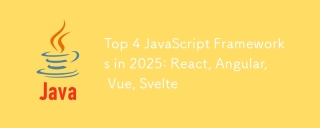
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
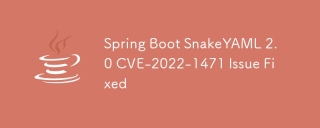
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
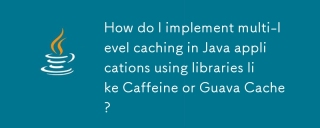
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
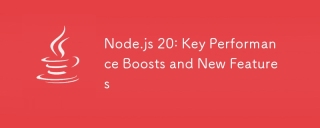
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
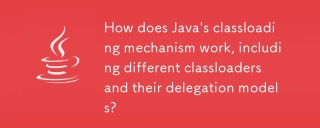
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
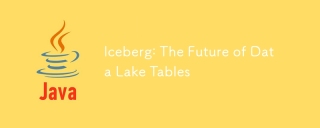
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
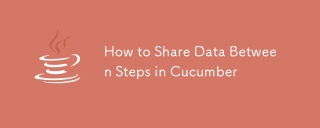
This article explores methods for sharing data between Cucumber steps, comparing scenario context, global variables, argument passing, and data structures. It emphasizes best practices for maintainability, including concise context use, descriptive
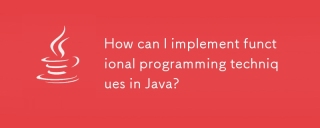
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
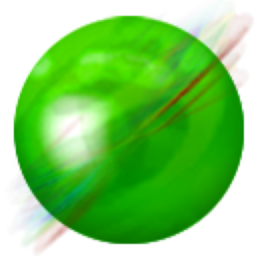
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

SublimeText3 English version
Recommended: Win version, supports code prompts!
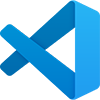
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft
