Docker Volumes: The Ultimate Solution for Data Persistence in Containers
In containerized applications, data persistence is crucial. Docker containers, by default, lose all data when removed. The ideal solution? Docker Volumes. They ensure data survival even after removing or restarting containers, offering isolation and scalability.
Why choose Docker Volumes?
- Persistence: When linking volumes to containers, data persists even after the container is destroyed or recreated.
- Isolation: Separating data storage from container logic simplifies organization, replacements and updates.
- Scalability: In multi-container environments, volumes facilitate data sharing.
-
Accelerated Development:
Bind mounts
, in particular, they allow editing of files locally with immediate reflection in the container.
Imagine the container as a rental car – when you change cars, you lose everything inside it. The volume is your personal suitcase, which accompanies you in any vehicle (container).
Practical Example 1: Bind Mount
for File Upload
Consider a Go application that receives file uploads. This example demonstrates how to keep these uploads persistent on the local computer, avoiding losses when removing the container.
Image Uploader
This simplified example creates an HTTP server for uploading and storing files in the uploads/
folder. The complete code is available on my GitHub. Here's an excerpt from handler
:
func UploadHandler(w http.ResponseWriter, r *http.Request) { if r.Method != http.MethodPost { writeJSONError(w, http.StatusMethodNotAllowed, "Método não permitido") return } file, header, err := r.FormFile("file") if err != nil { writeJSONError(w, http.StatusBadRequest, "Erro ao ler arquivo do formulário") return } defer file.Close() err = services.SaveUploadedFile(file, header.Filename) if err != nil { writeJSONError(w, http.StatusInternalServerError, fmt.Sprintf("Erro ao gravar arquivo: %v", err)) return } writeJSONSuccess(w, http.StatusOK, "Upload realizado com sucesso!", header.Filename) }
Dockerfile
This Dockerfile compiles the binary and configures the execution environment:
# syntax=docker/dockerfile:1 FROM golang:1.23-alpine AS builder WORKDIR /app COPY go.mod ./ RUN go mod download COPY . . RUN go build -o server ./cmd/image-uploader FROM alpine:3.21 WORKDIR /app COPY --from=builder /app/server /app/server RUN mkdir -p /app/uploads EXPOSE 8080 CMD ["/app/server"]
Creating and Running the Container with Bind Mount
- Build the image:
docker build -t go-upload-app:latest .
- Run the container, mapping the host's
uploads/
folder to the container:
docker run -d \ --name meu_container_go \ -p 8080:8080 \ -v /caminho/no/host/uploads:/app/uploads \ go-upload-app:latest
Please note -v /caminho/no/host/uploads:/app/uploads
:
- Left: path on host.
- Right: path in the container (/app/uploads).
Files sent via /upload
will be stored in the container and on the host. Removing the container preserves the files on the host.
Named Volumes
To have Docker manage data in a named volume (without relying on a local folder), here is an example with PostgreSQL:
docker volume create pg_dados docker run -d \ --name meu_postgres \ -e POSTGRES_PASSWORD=123456 \ -v pg_dados:/var/lib/postgresql/data \ postgres:latest
pg_dados
persists regardless of the containers that use it.
Security: Encrypting Volumes
For sensitive data, consider encrypting the file system or using volume drivers with encryption:
- Storage in encrypted partitions.
- Cloud storage solutions with encryption at rest.
- Specialized drivers (rexray, portworx) with built-in encryption.
Your data are confidential documents; protect them with encryption.
Example with Docker Compose
Docker Compose makes it easy to orchestrate multiple services. This example demonstrates data persistence with a database:
func UploadHandler(w http.ResponseWriter, r *http.Request) { if r.Method != http.MethodPost { writeJSONError(w, http.StatusMethodNotAllowed, "Método não permitido") return } file, header, err := r.FormFile("file") if err != nil { writeJSONError(w, http.StatusBadRequest, "Erro ao ler arquivo do formulário") return } defer file.Close() err = services.SaveUploadedFile(file, header.Filename) if err != nil { writeJSONError(w, http.StatusInternalServerError, fmt.Sprintf("Erro ao gravar arquivo: %v", err)) return } writeJSONSuccess(w, http.StatusOK, "Upload realizado com sucesso!", header.Filename) }
Running with Docker Compose
Start services: docker compose up -d
. Check status: docker compose ps
. Test the upload:
# syntax=docker/dockerfile:1 FROM golang:1.23-alpine AS builder WORKDIR /app COPY go.mod ./ RUN go mod download COPY . . RUN go build -o server ./cmd/image-uploader FROM alpine:3.21 WORKDIR /app COPY --from=builder /app/server /app/server RUN mkdir -p /app/uploads EXPOSE 8080 CMD ["/app/server"]
Stop and remove: docker compose down
. db_data
persists.
Conclusion
Docker volumes are essential for data persistence in containers. Bind mounts
are ideal for development, while named volumes are recommended for production. Correct use guarantees resilience and organization. Try it and share your experiences!
The above is the detailed content of Docker Volumes. For more information, please follow other related articles on the PHP Chinese website!
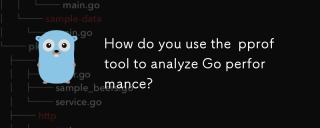
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
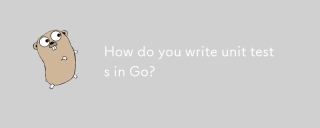
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
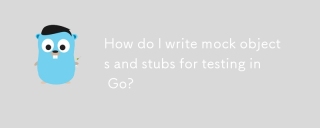
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
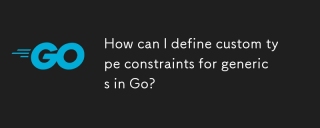
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
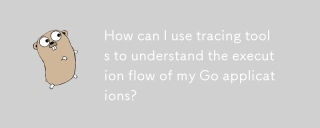
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
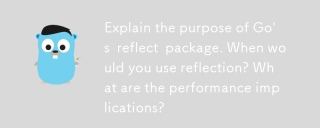
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
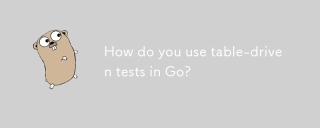
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
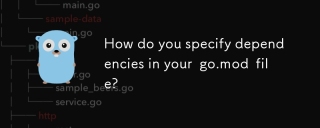
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
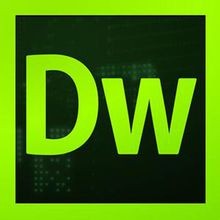
Dreamweaver CS6
Visual web development tools
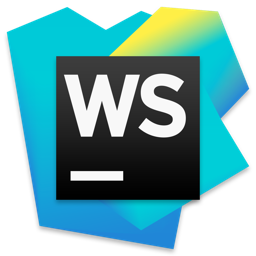
WebStorm Mac version
Useful JavaScript development tools

Notepad++7.3.1
Easy-to-use and free code editor

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.

Atom editor mac version download
The most popular open source editor
