Using Python Lists as Parameters in SQL Queries
This guide demonstrates how to efficiently query an SQL database using a Python list to specify parameter values. This technique allows for flexible data retrieval based on a set of values from your Python code.
Here's a step-by-step approach:
-
Construct the SQL Query: Create your SQL query with a placeholder for the list values. For instance, to select names from a "students" table where the "id" is in a Python list:
SELECT name FROM students WHERE id IN (?)
-
Generate Placeholders: Transform your Python list into a comma-separated string of placeholders. For the list
[1, 5, 8]
, this would be:placeholders = ', '.join(['?'] * len([1, 5, 8])) # Results in '?, ?, ?'
-
Create the Parameterized Query: Integrate the placeholder string into your SQL query using your database library's formatting methods. The example below uses f-strings (Python 3.6 ):
query = f'SELECT name FROM students WHERE id IN ({placeholders})'
-
Execute the Query: Execute the query, supplying your Python list as a tuple to the database cursor's
execute()
method. This ensures proper parameter binding and prevents SQL injection vulnerabilities.cursor.execute(query, tuple([1, 5, 8])) results = cursor.fetchall()
This method ensures efficient and secure data retrieval from your SQL database using values directly from a Python list, whether those values are integers, strings, or other compatible data types. Remember to adapt the code to your specific database library (e.g., sqlite3
, psycopg2
).
The above is the detailed content of How Can I Query an SQL Database Using a Python List as a Parameter?. For more information, please follow other related articles on the PHP Chinese website!
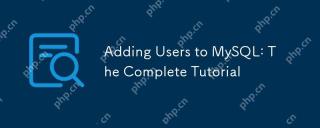
Mastering the method of adding MySQL users is crucial for database administrators and developers because it ensures the security and access control of the database. 1) Create a new user using the CREATEUSER command, 2) Assign permissions through the GRANT command, 3) Use FLUSHPRIVILEGES to ensure permissions take effect, 4) Regularly audit and clean user accounts to maintain performance and security.
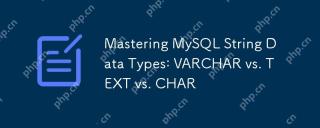
ChooseCHARforfixed-lengthdata,VARCHARforvariable-lengthdata,andTEXTforlargetextfields.1)CHARisefficientforconsistent-lengthdatalikecodes.2)VARCHARsuitsvariable-lengthdatalikenames,balancingflexibilityandperformance.3)TEXTisidealforlargetextslikeartic
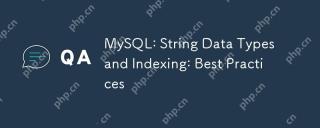
Best practices for handling string data types and indexes in MySQL include: 1) Selecting the appropriate string type, such as CHAR for fixed length, VARCHAR for variable length, and TEXT for large text; 2) Be cautious in indexing, avoid over-indexing, and create indexes for common queries; 3) Use prefix indexes and full-text indexes to optimize long string searches; 4) Regularly monitor and optimize indexes to keep indexes small and efficient. Through these methods, we can balance read and write performance and improve database efficiency.
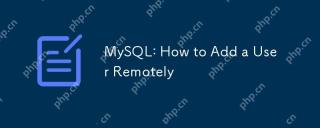
ToaddauserremotelytoMySQL,followthesesteps:1)ConnecttoMySQLasroot,2)Createanewuserwithremoteaccess,3)Grantnecessaryprivileges,and4)Flushprivileges.BecautiousofsecurityrisksbylimitingprivilegesandaccesstospecificIPs,ensuringstrongpasswords,andmonitori
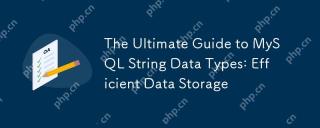
TostorestringsefficientlyinMySQL,choosetherightdatatypebasedonyourneeds:1)UseCHARforfixed-lengthstringslikecountrycodes.2)UseVARCHARforvariable-lengthstringslikenames.3)UseTEXTforlong-formtextcontent.4)UseBLOBforbinarydatalikeimages.Considerstorageov
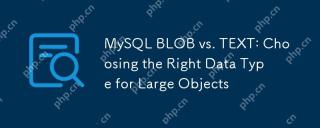
When selecting MySQL's BLOB and TEXT data types, BLOB is suitable for storing binary data, and TEXT is suitable for storing text data. 1) BLOB is suitable for binary data such as pictures and audio, 2) TEXT is suitable for text data such as articles and comments. When choosing, data properties and performance optimization must be considered.
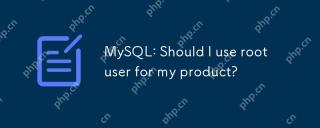
No,youshouldnotusetherootuserinMySQLforyourproduct.Instead,createspecificuserswithlimitedprivilegestoenhancesecurityandperformance:1)Createanewuserwithastrongpassword,2)Grantonlynecessarypermissionstothisuser,3)Regularlyreviewandupdateuserpermissions
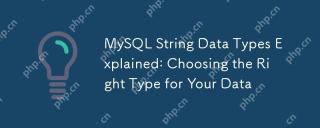
MySQLstringdatatypesshouldbechosenbasedondatacharacteristicsandusecases:1)UseCHARforfixed-lengthstringslikecountrycodes.2)UseVARCHARforvariable-lengthstringslikenames.3)UseBINARYorVARBINARYforbinarydatalikecryptographickeys.4)UseBLOBorTEXTforlargeuns


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Linux new version
SublimeText3 Linux latest version
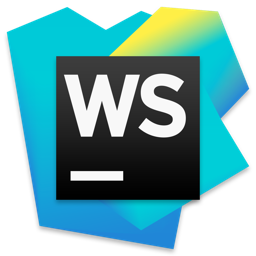
WebStorm Mac version
Useful JavaScript development tools
