


This document describes a Python project that retrieves weather data and stores it in an AWS S3 bucket. Let's rephrase it for clarity and improved flow, maintaining the original language and image positions.
Weather Dashboard Project
This Python project, the Weather Dashboard, retrieves weather data via the OpenWeather API and securely uploads it to an AWS S3 bucket. It provides a straightforward interface for viewing weather information for various cities and seamlessly saves the results to the cloud. The project's scalability is enhanced by leveraging AWS S3 for data storage.
Table of Contents
- Prerequisites
- Project Overview
- Core Functionality
- Technologies Used
- Project Setup
- Environment Configuration
- Running the Application
Prerequisites
Before starting, ensure you have:
- Python 3.x: Download and install from the official Python website.
- AWS Account: Create an account to access AWS S3.
- OpenWeather API Key: Obtain a key from the OpenWeather website.
- AWS CLI: Download and install the AWS Command Line Interface.
- Python Proficiency: Basic understanding of Python scripting, API interaction, and environment variables.
- Code Editor/IDE: Use VS Code, PyCharm, or a similar development environment.
- Git: Install Git for version control (available from the Git website).
Project Overview
This Weather Dashboard utilizes the OpenWeather API to fetch weather information for specified locations. This data is then uploaded to an AWS S3 bucket for convenient remote access. The system's design allows users to input different cities and receive real-time weather updates.
Core Functionality
- Retrieves weather data from the OpenWeather API.
- Uploads weather data to an AWS S3 bucket.
- Securely manages API keys and AWS credentials using environment variables.
Technologies Used
The project utilizes:
- Python 3.x: The primary programming language.
- boto3: The AWS SDK for Python, enabling interaction with AWS S3.
-
python-dotenv: Facilitates secure storage and retrieval of environment variables from a
.env
file. - requests: A streamlined HTTP library for making API calls to OpenWeather.
- AWS CLI: The command-line interface for managing AWS services (including key configuration and S3 bucket management).
Project Setup
Follow these steps to set up the project locally:
1. Create Project Directory Structure
<code>weather-dashboard/ ├── src/ │ ├── __init__.py │ └── weather_dashboard.py ├── .env ├── tests/ ├── data/ ├── .gitignore └── README.md</code>
Create the directories and files using these commands:
mkdir weather_dashboard_demo cd weather_dashboard_demo mkdir src tests data
2. Create Files
Create the necessary Python and configuration files:
touch src/__init__.py src/weather_dashboard.py touch requirements.txt README.md .env
3. Initialize Git Repository
Initialize a Git repository and set the main branch:
git init git branch -M main
4. Configure .gitignore
Create a .gitignore
file to exclude unnecessary files:
echo ".env" >> .gitignore echo "__pycache__/" >> .gitignore echo "*.zip" >> .gitignore
5. Add Dependencies
Add required packages to requirements.txt
:
echo "boto3==1.26.137" >> requirements.txt echo "python-dotenv==1.0.0" >> requirements.txt echo "requests==2.28.2" >> requirements.txt
6. Install Dependencies
Install the dependencies:
<code>weather-dashboard/ ├── src/ │ ├── __init__.py │ └── weather_dashboard.py ├── .env ├── tests/ ├── data/ ├── .gitignore └── README.md</code>
Environment Configuration
1. AWS CLI Configuration
Configure the AWS CLI with your access keys:
mkdir weather_dashboard_demo cd weather_dashboard_demo mkdir src tests data
You'll be prompted for your Access Key ID, Secret Access Key, region, and output format. Obtain your credentials from the AWS Management Console (IAM > Users > Your User > Security Credentials).
Check the installation with:
touch src/__init__.py src/weather_dashboard.py touch requirements.txt README.md .env
2. Configure .env
Create a .env
file containing your API key and bucket name:
git init git branch -M main
Replace placeholders with your actual values.
Running the Application
Here's the Python script (weather_dashboard.py
):
echo ".env" >> .gitignore echo "__pycache__/" >> .gitignore echo "*.zip" >> .gitignore
1. Run the Script
Execute the script:
echo "boto3==1.26.137" >> requirements.txt echo "python-dotenv==1.0.0" >> requirements.txt echo "requests==2.28.2" >> requirements.txt
This fetches weather data and uploads it to your S3 bucket.
2. Verify S3 Bucket
Access your AWS S3 bucket to confirm the upload. Remember to delete the data afterward to avoid unnecessary charges.
This revised version maintains the original information while improving readability and flow. Remember to replace placeholder values with your actual API key and bucket name.
The above is the detailed content of Building a Scalable Real-Time Weather Dashboard with Python, OpenWeather API, and AWS S3. For more information, please follow other related articles on the PHP Chinese website!
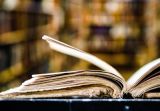
This tutorial demonstrates how to use Python to process the statistical concept of Zipf's law and demonstrates the efficiency of Python's reading and sorting large text files when processing the law. You may be wondering what the term Zipf distribution means. To understand this term, we first need to define Zipf's law. Don't worry, I'll try to simplify the instructions. Zipf's Law Zipf's law simply means: in a large natural language corpus, the most frequently occurring words appear about twice as frequently as the second frequent words, three times as the third frequent words, four times as the fourth frequent words, and so on. Let's look at an example. If you look at the Brown corpus in American English, you will notice that the most frequent word is "th
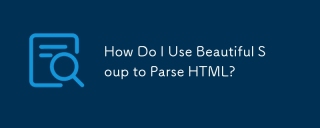
This article explains how to use Beautiful Soup, a Python library, to parse HTML. It details common methods like find(), find_all(), select(), and get_text() for data extraction, handling of diverse HTML structures and errors, and alternatives (Sel
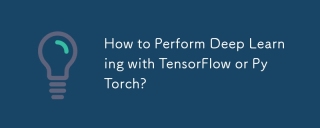
This article compares TensorFlow and PyTorch for deep learning. It details the steps involved: data preparation, model building, training, evaluation, and deployment. Key differences between the frameworks, particularly regarding computational grap
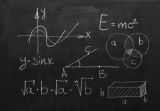
Python's statistics module provides powerful data statistical analysis capabilities to help us quickly understand the overall characteristics of data, such as biostatistics and business analysis. Instead of looking at data points one by one, just look at statistics such as mean or variance to discover trends and features in the original data that may be ignored, and compare large datasets more easily and effectively. This tutorial will explain how to calculate the mean and measure the degree of dispersion of the dataset. Unless otherwise stated, all functions in this module support the calculation of the mean() function instead of simply summing the average. Floating point numbers can also be used. import random import statistics from fracti
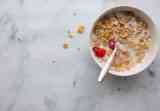
Serialization and deserialization of Python objects are key aspects of any non-trivial program. If you save something to a Python file, you do object serialization and deserialization if you read the configuration file, or if you respond to an HTTP request. In a sense, serialization and deserialization are the most boring things in the world. Who cares about all these formats and protocols? You want to persist or stream some Python objects and retrieve them in full at a later time. This is a great way to see the world on a conceptual level. However, on a practical level, the serialization scheme, format or protocol you choose may determine the speed, security, freedom of maintenance status, and other aspects of the program
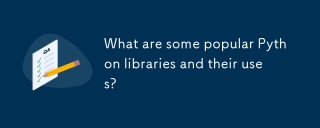
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
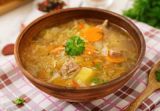
This tutorial builds upon the previous introduction to Beautiful Soup, focusing on DOM manipulation beyond simple tree navigation. We'll explore efficient search methods and techniques for modifying HTML structure. One common DOM search method is ex
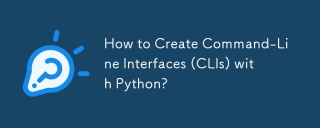
This article guides Python developers on building command-line interfaces (CLIs). It details using libraries like typer, click, and argparse, emphasizing input/output handling, and promoting user-friendly design patterns for improved CLI usability.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.

SublimeText3 Linux new version
SublimeText3 Linux latest version
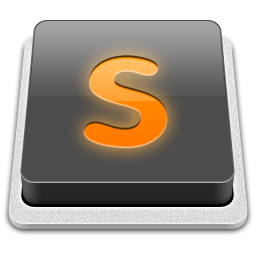
SublimeText3 Mac version
God-level code editing software (SublimeText3)

Zend Studio 13.0.1
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use
