Welcome to part two of our Go Programming Tutorial Series, designed to build a solid Go (Golang) foundation. This article, focusing on Go Fundamentals: Syntax and Structure, covers everything from your first "Hello, World!" program to variables, constants, data types, and more. Whether you're a novice or seeking to refine your skills, this guide provides the knowledge for writing efficient, clean Go code.
Upon completion, you will be able to:
- Create your first Go program: Hello, World!
- Understand the
main
package andmain
function. - Work with variables, constants, and data types.
- Grasp the concept of zero values in Go.
- Utilize type inference and type conversion.
Let's begin!
Core Concepts
1. Your First Go Program: Hello, World!
Every programming journey starts with "Hello, World!". In Go:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
Explanation:
-
package main
: Every Go program begins with a package declaration.main
signifies an executable program. -
import "fmt"
: Imports thefmt
package for console output (likePrintln
). -
func main()
: The program's entry point. Execution starts here. -
fmt.Println("Hello, World!")
: Prints "Hello, World!" to the console.
2. Understanding the main
Package and main
Function
- The
main
package is essential for creating executable Go programs. Without it, your code won't run independently. - The
main
function is mandatory within themain
package; it's the program's starting point.
3. Basic Syntax: Variables, Constants, and Data Types
Go is statically typed—you must specify a variable's data type. However, Go also supports type inference for concise code.
Variables
Variables are declared using var
:
var name string = "Go Programmer" var age int = 30
Shorthand (within functions):
name := "Go Programmer" age := 30
Constants
Constants are immutable values, declared with const
:
const pi float64 = 3.14159
Data Types
Go offers various built-in data types:
-
Basic types:
int
,float64
,string
,bool
. Example:
var age int = 35 var price float64 = 29.99 var name string = "Bob" var isActive bool = true
-
Composite types:
array
,slice
,struct
,map
. Example:
// Array var scores [3]int = [3]int{95, 80, 92} // Slice var grades []float64 = []float64{88.2, 91.5, 78.9} // Struct type Person struct { FirstName string LastName string Age int } var person = Person{"Jane", "Doe", 28} // Map var capitals map[string]string = map[string]string{ "France": "Paris", "Italy": "Rome", }
4. Zero Values in Go
Variables without explicit initialization receive their zero value:
- 0 for numeric types.
-
false
for booleans. -
""
(empty string) for strings. -
nil
for pointers, slices, maps, and channels.
Example:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
5. Type Inference and Type Conversion
Go infers variable types from assigned values:
var name string = "Go Programmer" var age int = 30
Type conversion requires explicit casting:
name := "Go Programmer" age := 30
Practical Example
Let's create a program demonstrating variables, constants, data types, zero values, type inference, and type conversion:
const pi float64 = 3.14159
(The code would be identical to the example in the original text, with potentially slightly different variable names to maintain clarity and avoid repetition.)
Output (Similar to the original output)
(The output would be similar to the original example, reflecting the values and types of the variables.)
Explanation:
(The explanation would be the same as in the original, explaining each section of the code.)
Best Practices
- Descriptive Variable Names: Use clear, meaningful names.
var age int = 35 var price float64 = 29.99 var name string = "Bob" var isActive bool = true
-
Type Inference: Use
:=
when the type is obvious.
// Array var scores [3]int = [3]int{95, 80, 92} // Slice var grades []float64 = []float64{88.2, 91.5, 78.9} // Struct type Person struct { FirstName string LastName string Age int } var person = Person{"Jane", "Doe", 28} // Map var capitals map[string]string = map[string]string{ "France": "Paris", "Italy": "Rome", }
- Avoid Unnecessary Type Conversions: Convert only when needed.
- Explicit Variable Initialization: Initialize variables clearly.
-
Clean
main
Function: Keepmain
concise; delegate logic to other functions.
Conclusion
This article covered Go's basic syntax and structure, including the "Hello, World!" program, the main
package and function, variables, constants, data types, zero values, type inference, and type conversion. Mastering these fundamentals is crucial for your Go programming journey.
Experiment with the example program or create your own to solidify your understanding.
Call to Action
This article is part of our ongoing Go Tutorial Series. Look for the next tutorial on Control Structures in Go!
Happy coding! ?
The above is the detailed content of Go Basics: Syntax and Structure. For more information, please follow other related articles on the PHP Chinese website!
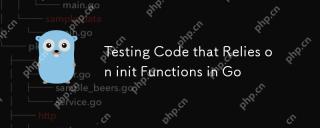
WhentestingGocodewithinitfunctions,useexplicitsetupfunctionsorseparatetestfilestoavoiddependencyoninitfunctionsideeffects.1)Useexplicitsetupfunctionstocontrolglobalvariableinitialization.2)Createseparatetestfilestobypassinitfunctionsandsetupthetesten
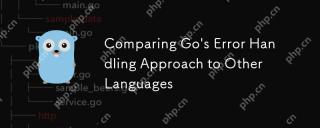
Go'serrorhandlingreturnserrorsasvalues,unlikeJavaandPythonwhichuseexceptions.1)Go'smethodensuresexpliciterrorhandling,promotingrobustcodebutincreasingverbosity.2)JavaandPython'sexceptionsallowforcleanercodebutcanleadtooverlookederrorsifnotmanagedcare
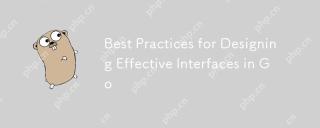
AneffectiveinterfaceinGoisminimal,clear,andpromotesloosecoupling.1)Minimizetheinterfaceforflexibilityandeaseofimplementation.2)Useinterfacesforabstractiontoswapimplementationswithoutchangingcallingcode.3)Designfortestabilitybyusinginterfacestomockdep
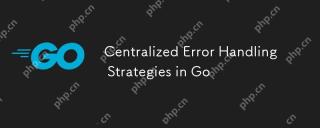
Centralized error handling can improve the readability and maintainability of code in Go language. Its implementation methods and advantages include: 1. Separate error handling logic from business logic and simplify code. 2. Ensure the consistency of error handling by centrally handling. 3. Use defer and recover to capture and process panics to enhance program robustness.
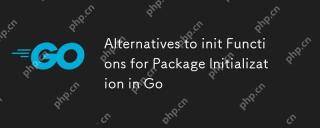
InGo,alternativestoinitfunctionsincludecustominitializationfunctionsandsingletons.1)Custominitializationfunctionsallowexplicitcontroloverwheninitializationoccurs,usefulfordelayedorconditionalsetups.2)Singletonsensureone-timeinitializationinconcurrent
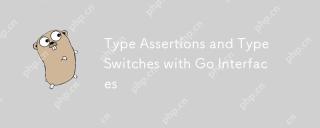
Gohandlesinterfacesandtypeassertionseffectively,enhancingcodeflexibilityandrobustness.1)Typeassertionsallowruntimetypechecking,asseenwiththeShapeinterfaceandCircletype.2)Typeswitcheshandlemultipletypesefficiently,usefulforvariousshapesimplementingthe
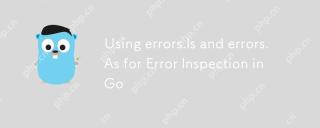
Go language error handling becomes more flexible and readable through errors.Is and errors.As functions. 1.errors.Is is used to check whether the error is the same as the specified error and is suitable for the processing of the error chain. 2.errors.As can not only check the error type, but also convert the error to a specific type, which is convenient for extracting error information. Using these functions can simplify error handling logic, but pay attention to the correct delivery of error chains and avoid excessive dependence to prevent code complexity.
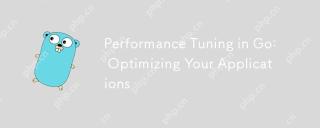
TomakeGoapplicationsrunfasterandmoreefficiently,useprofilingtools,leverageconcurrency,andmanagememoryeffectively.1)UsepprofforCPUandmemoryprofilingtoidentifybottlenecks.2)Utilizegoroutinesandchannelstoparallelizetasksandimproveperformance.3)Implement


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
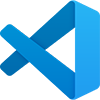
VSCode Windows 64-bit Download
A free and powerful IDE editor launched by Microsoft

SublimeText3 Linux new version
SublimeText3 Linux latest version

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
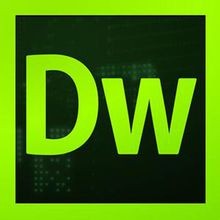
Dreamweaver CS6
Visual web development tools
