


How Can I Elegantly Generate Prime Numbers in Java Using the Sieve of Eratosthenes?
Sieve of Eratosthenes in Java: Generate prime numbers elegantly
Introduction
Prime number generation is a fundamental problem in computer science, with a variety of algorithms to choose from. Among them, the sieve of Eratosthenes is known for its simplicity and efficiency. This article provides an elegant Java implementation that uses the sieve of Eratosthenes to generate the first n prime numbers.
Sieve of Eratosthenes
The Sieve of Eratosthenes is a probabilistic algorithm that identifies prime numbers by iteratively eliminating multiples of prime numbers. It first initializes an array of boolean flags, each flag representing a number up to the specified limit. The algorithm then iterates through the array starting with the first prime number 2 and marks all multiples of it as non-prime. This process continues until all numbers within the limit have been eliminated, leaving only prime numbers.
Elegant implementation
An elegant Java implementation of the Sieve of Eratosthenes looks like this:
public static BitSet computePrimes(int limit) { final BitSet primes = new BitSet(); primes.set(0, false); primes.set(1, false); primes.set(2, limit, true); for (int i = 2; i * i <= limit; i++) { if (primes.get(i)) { for (int j = i * i; j <= limit; j += i) { primes.set(j, false); } } } return primes; }
Description
This implementation creates a BitSet where each bit represents a number up to the specified limit. Initially, 0 and 1 are marked as non-prime and all other numbers are marked as prime.
The outer loop iterates through the array starting from the first prime number 2. If the bit at the current position is set (indicating that it is prime), the inner loop marks all multiples of that prime number as non-prime. This process continues until all numbers within the limit have been eliminated.
Finally, return the BitSet containing the prime numbers.
Conclusion
This Java implementation of the Sieve of Eratosthenes demonstrates the elegance and simplicity of the algorithm. It generates prime numbers efficiently and has a clear, logical structure. The code is optimized for performance and understandability, making it a valuable tool for programmers who need a prime number generator.
The above is the detailed content of How Can I Elegantly Generate Prime Numbers in Java Using the Sieve of Eratosthenes?. For more information, please follow other related articles on the PHP Chinese website!
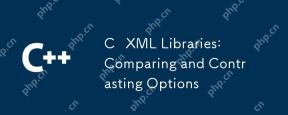
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
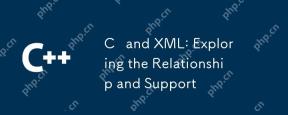
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
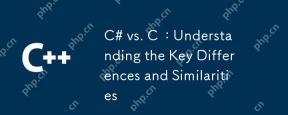
The main differences between C# and C are syntax, performance and application scenarios. 1) The C# syntax is more concise, supports garbage collection, and is suitable for .NET framework development. 2) C has higher performance and requires manual memory management, which is often used in system programming and game development.
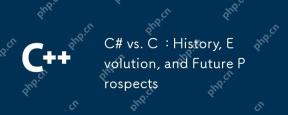
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
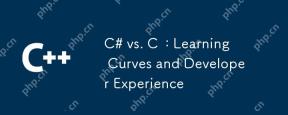
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
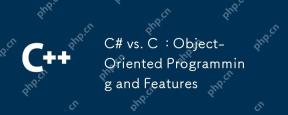
There are significant differences in how C# and C implement and features in object-oriented programming (OOP). 1) The class definition and syntax of C# are more concise and support advanced features such as LINQ. 2) C provides finer granular control, suitable for system programming and high performance needs. Both have their own advantages, and the choice should be based on the specific application scenario.
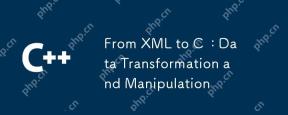
Converting from XML to C and performing data operations can be achieved through the following steps: 1) parsing XML files using tinyxml2 library, 2) mapping data into C's data structure, 3) using C standard library such as std::vector for data operations. Through these steps, data converted from XML can be processed and manipulated efficiently.
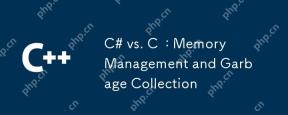
C# uses automatic garbage collection mechanism, while C uses manual memory management. 1. C#'s garbage collector automatically manages memory to reduce the risk of memory leakage, but may lead to performance degradation. 2.C provides flexible memory control, suitable for applications that require fine management, but should be handled with caution to avoid memory leakage.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
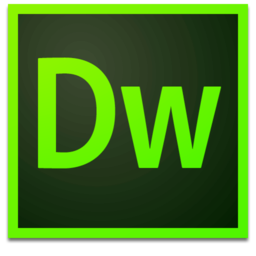
Dreamweaver Mac version
Visual web development tools
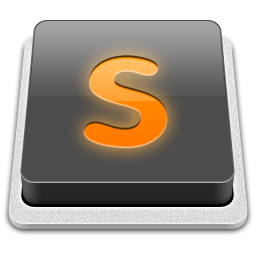
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
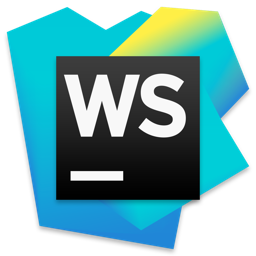
WebStorm Mac version
Useful JavaScript development tools