Handling multiple JWT issuers in ASP.NET Core 2
ASP.NET Core 2 supports handling multiple JWT token providers. This functionality is critical when your API needs to integrate with external services that use different JWT token issuance mechanisms.
To achieve this function, please use the following code:
services .AddAuthentication() .AddJwtBearer("Firebase", options => { options.Authority = "https://securetoken.google.com/my-firebase-project"; options.TokenValidationParameters = new TokenValidationParameters { ValidateIssuer = true, ValidIssuer = "my-firebase-project", ValidateAudience = true, ValidAudience = "my-firebase-project", ValidateLifetime = true }; }) .AddJwtBearer("Custom", options => { // 在此处配置自定义 JWT 令牌选项 }); services .AddAuthorization(options => { options.DefaultPolicy = new AuthorizationPolicyBuilder() .RequireAuthenticatedUser() .AddAuthenticationSchemes("Firebase", "Custom") .Build(); });
This code differs from the original code snippet in several key ways:
-
Authentication scheme overloads: The
AddJwtBearer
method has multiple overloads, one of which allows specifying the authentication scheme name. Since we have multiple scenarios, this overload must be used. -
No default authentication scheme: The
AddAuthentication
method is used without parameters to avoid setting a default authentication scheme. This is because handling multiple authentication schemes requires each request to go through all schemes. -
Policy Update:
DefaultPolicy
has been modified to allow "Firebase" and "Custom" authentication schemes. This ensures that the authorization system attempts to authenticate the request using both schemes. -
Authentication Handling: If you handle the
AuthenticationFailed
event, please note that for non-firstAddJwtBearer
policies, the error "IDX10501: Signature validation failed" may occur. This is because the system attempts to match the signature of each policy in turn.
Additional Notes for .NET Core 6 and above
In .NET Core 6 and above, a default authorization scheme must be specified. So use the following code instead:
builder.Services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme) .AddJwtBearer(options => { // Firebase 配置 }) .AddJwtBearer("AzureAD", options => { // Azure AD 配置 }); builder.Services.AddAuthorization(options => { var defaultAuthorizationPolicyBuilder = new AuthorizationPolicyBuilder( JwtBearerDefaults.AuthenticationScheme, "AzureAD"); defaultAuthorizationPolicyBuilder = defaultAuthorizationPolicyBuilder.RequireAuthenticatedUser(); options.DefaultPolicy = defaultAuthorizationPolicyBuilder.Build(); });
By implementing the provided solution, you can seamlessly manage multiple JWT token issuers in your ASP.NET Core 2 application.
The above is the detailed content of How to Handle Multiple JWT Issuers in ASP.NET Core?. For more information, please follow other related articles on the PHP Chinese website!
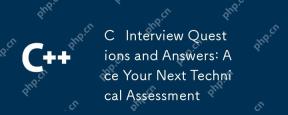
C In interviews, smart pointers are the key tools that help manage memory and reduce memory leaks. 1) std::unique_ptr provides exclusive ownership to ensure that resources are automatically released. 2) std::shared_ptr is used for shared ownership and is suitable for multi-reference scenarios. 3) std::weak_ptr can avoid circular references and ensure secure resource management.
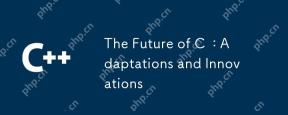
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
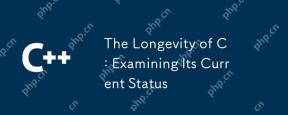
C is still important in modern programming because of its efficient, flexible and powerful nature. 1)C supports object-oriented programming, suitable for system programming, game development and embedded systems. 2) Polymorphism is the highlight of C, allowing the call to derived class methods through base class pointers or references to enhance the flexibility and scalability of the code.
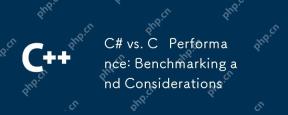
The performance differences between C# and C are mainly reflected in execution speed and resource management: 1) C usually performs better in numerical calculations and string operations because it is closer to hardware and has no additional overhead such as garbage collection; 2) C# is more concise in multi-threaded programming, but its performance is slightly inferior to C; 3) Which language to choose should be determined based on project requirements and team technology stack.
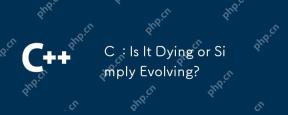
C isnotdying;it'sevolving.1)C remainsrelevantduetoitsversatilityandefficiencyinperformance-criticalapplications.2)Thelanguageiscontinuouslyupdated,withC 20introducingfeatureslikemodulesandcoroutinestoimproveusabilityandperformance.3)Despitechallen
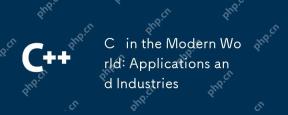
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
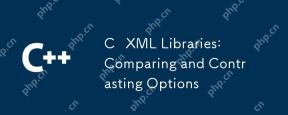
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
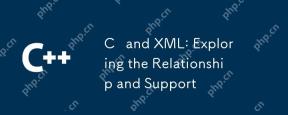
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
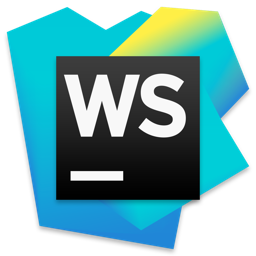
WebStorm Mac version
Useful JavaScript development tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
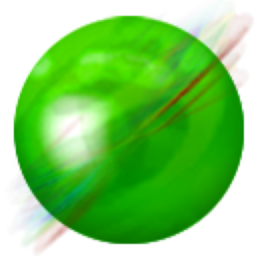
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
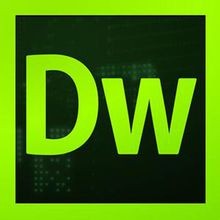
Dreamweaver CS6
Visual web development tools
