


How Can I Efficiently Parse Large and Malformed JSON Files in .NET Using Json.NET?
Parsing Large and Malformed JSON Files in .NET Using Json.NET
Parsing large JSON files can be challenging in .NET, especially if the JSON is malformed. In this article, we will explore the challenges encountered when parsing very large JSON files and provide a solution using Json.NET.
The Issue: Nested Array Structure
One common issue with large JSON files is their complex structure. In your case, the JSON file contains an array of objects, but each array is enclosed within brackets that close and open immediately. This makes the JSON technically invalid when taken as a whole.
Using JsonTextReader with SupportMultipleContent
To handle this malformed JSON format, Json.NET offers a special setting in the JsonTextReader class. By setting the SupportMultipleContent flag to true, we can parse each item individually within a loop.
This approach allows for memory-efficient processing of non-standard JSON, regardless of its size or the number of nested arrays. The code below demonstrates this technique:
using (WebClient client = new WebClient()) using (Stream stream = client.OpenRead(stringUrl)) using (StreamReader streamReader = new StreamReader(stream)) using (JsonTextReader reader = new JsonTextReader(streamReader)) { reader.SupportMultipleContent = true; var serializer = new JsonSerializer(); while (reader.Read()) { if (reader.TokenType == JsonToken.StartObject) { Contact c = serializer.Deserialize<contact>(reader); Console.WriteLine(c.FirstName + " " + c.LastName); } } }</contact>
In this example, the JSONTextReader first checks the token type. If it encounters an object start token, it deserializes the current object into a Contact instance using JsonSerializer. Each contact's first and last names are then printed to the console.
Conclusion
By utilizing the SupportMultipleContent setting in JsonTextReader, you can effectively parse large and malformed JSON files in .NET. This technique allows for efficient memory usage and handles complex JSON structures, making it Ideal for processing JSON data streams.
The above is the detailed content of How Can I Efficiently Parse Large and Malformed JSON Files in .NET Using Json.NET?. For more information, please follow other related articles on the PHP Chinese website!
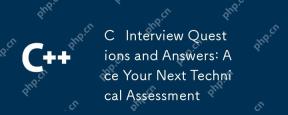
C In interviews, smart pointers are the key tools that help manage memory and reduce memory leaks. 1) std::unique_ptr provides exclusive ownership to ensure that resources are automatically released. 2) std::shared_ptr is used for shared ownership and is suitable for multi-reference scenarios. 3) std::weak_ptr can avoid circular references and ensure secure resource management.
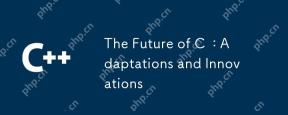
The future of C will focus on parallel computing, security, modularization and AI/machine learning: 1) Parallel computing will be enhanced through features such as coroutines; 2) Security will be improved through stricter type checking and memory management mechanisms; 3) Modulation will simplify code organization and compilation; 4) AI and machine learning will prompt C to adapt to new needs, such as numerical computing and GPU programming support.
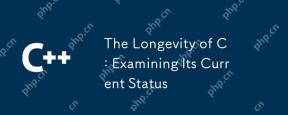
C is still important in modern programming because of its efficient, flexible and powerful nature. 1)C supports object-oriented programming, suitable for system programming, game development and embedded systems. 2) Polymorphism is the highlight of C, allowing the call to derived class methods through base class pointers or references to enhance the flexibility and scalability of the code.
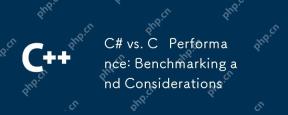
The performance differences between C# and C are mainly reflected in execution speed and resource management: 1) C usually performs better in numerical calculations and string operations because it is closer to hardware and has no additional overhead such as garbage collection; 2) C# is more concise in multi-threaded programming, but its performance is slightly inferior to C; 3) Which language to choose should be determined based on project requirements and team technology stack.
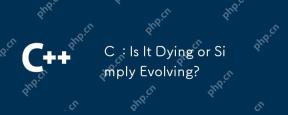
C isnotdying;it'sevolving.1)C remainsrelevantduetoitsversatilityandefficiencyinperformance-criticalapplications.2)Thelanguageiscontinuouslyupdated,withC 20introducingfeatureslikemodulesandcoroutinestoimproveusabilityandperformance.3)Despitechallen
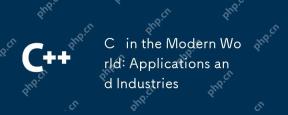
C is widely used and important in the modern world. 1) In game development, C is widely used for its high performance and polymorphism, such as UnrealEngine and Unity. 2) In financial trading systems, C's low latency and high throughput make it the first choice, suitable for high-frequency trading and real-time data analysis.
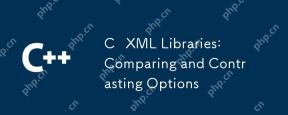
There are four commonly used XML libraries in C: TinyXML-2, PugiXML, Xerces-C, and RapidXML. 1.TinyXML-2 is suitable for environments with limited resources, lightweight but limited functions. 2. PugiXML is fast and supports XPath query, suitable for complex XML structures. 3.Xerces-C is powerful, supports DOM and SAX resolution, and is suitable for complex processing. 4. RapidXML focuses on performance and parses extremely fast, but does not support XPath queries.
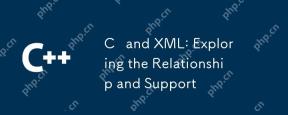
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
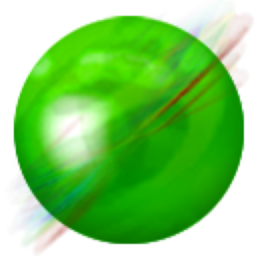
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Atom editor mac version download
The most popular open source editor

Notepad++7.3.1
Easy-to-use and free code editor
