


Expanding the Custom Membership Provider for ASP.NET MVC 2
Incorporating a custom membership provider into an ASP.NET MVC 2 application can enhance the user authentication and authorization process.
Implementing a Custom Membership Provider
To create a custom membership provider, inherit from the MembershipProvider abstract class and override the ValidateUser method to authenticate users against a database or other data source.
public override bool ValidateUser(string username, string password) { // Validate user credentials against a database or other data source }
Integrating the Membership Provider with ASP.NET MVC 2
Once the custom membership provider is created, it can be integrated into the ASP.NET MVC 2 project by adding a reference and setting it as the default provider in the web.config file:
<membership defaultprovider="MyMembershipProvider"> <providers> <add name="MyMembershipProvider" type="MyApp.MyMembershipProvider"></add> </providers> </membership>
Creating a Custom Role Provider
To implement role-based authorization, create a custom role provider that inherits from the RoleProvider abstract class and overrides the GetRolesForUser method to retrieve the roles assigned to a user.
public override string[] GetRolesForUser(string username) { // Get roles for the user from a database or other data source }
Integrating the Role Provider with ASP.NET MVC 2
Connect the role provider to the ASP.NET MVC 2 application in the web.config file:
<rolemanager enabled="true" defaultprovider="MyRoleProvider"> <providers> <add name="MyRoleProvider" type="MyApp.MyRoleProvider"></add> </providers> </rolemanager>
Applying Authorization to Controllers and Actions
Protect controller actions by applying the Authorize attribute with the desired roles:
[Authorize(Roles = "Customer Manager,Content Editor")] public class MyController : Controller { // Controller logic }
Customizing Authorization Failure Handling
Create a custom Authorize attribute to provide custom error handling, such as redirecting to an "Access Denied" page:
public class MyAuthorizationAttribute : AuthorizeAttribute { // Custom error handling logic }
Summary
By implementing a custom membership and role provider, ASP.NET MVC 2 applications can leverage tailored authentication and authorization mechanisms to enhance security and access control.
The above is the detailed content of How to Extend ASP.NET MVC 2's Authentication and Authorization with Custom Membership and Role Providers?. For more information, please follow other related articles on the PHP Chinese website!
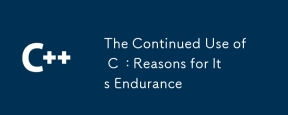
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
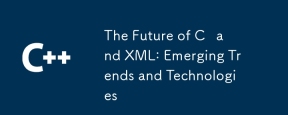
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
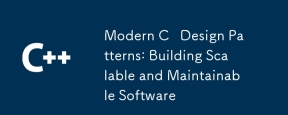
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
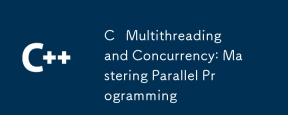
C The core concepts of multithreading and concurrent programming include thread creation and management, synchronization and mutual exclusion, conditional variables, thread pooling, asynchronous programming, common errors and debugging techniques, and performance optimization and best practices. 1) Create threads using the std::thread class. The example shows how to create and wait for the thread to complete. 2) Synchronize and mutual exclusion to use std::mutex and std::lock_guard to protect shared resources and avoid data competition. 3) Condition variables realize communication and synchronization between threads through std::condition_variable. 4) The thread pool example shows how to use the ThreadPool class to process tasks in parallel to improve efficiency. 5) Asynchronous programming uses std::as
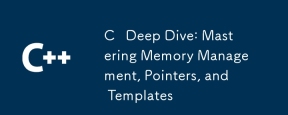
C's memory management, pointers and templates are core features. 1. Memory management manually allocates and releases memory through new and deletes, and pay attention to the difference between heap and stack. 2. Pointers allow direct operation of memory addresses, and use them with caution. Smart pointers can simplify management. 3. Template implements generic programming, improves code reusability and flexibility, and needs to understand type derivation and specialization.
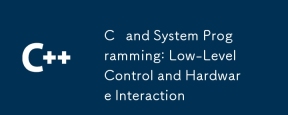
C is suitable for system programming and hardware interaction because it provides control capabilities close to hardware and powerful features of object-oriented programming. 1)C Through low-level features such as pointer, memory management and bit operation, efficient system-level operation can be achieved. 2) Hardware interaction is implemented through device drivers, and C can write these drivers to handle communication with hardware devices.
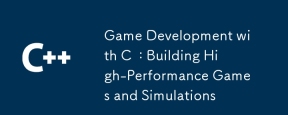
C is suitable for building high-performance gaming and simulation systems because it provides close to hardware control and efficient performance. 1) Memory management: Manual control reduces fragmentation and improves performance. 2) Compilation-time optimization: Inline functions and loop expansion improve running speed. 3) Low-level operations: Direct access to hardware, optimize graphics and physical computing.
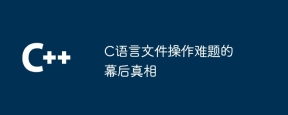
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
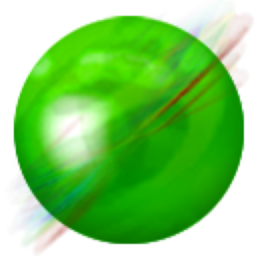
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

Atom editor mac version download
The most popular open source editor