Natural Sorting in Strings
Problem Statement
Sorting strings lexicographically does not always yield the desired order. For instance, lists containing numerical values may be sorted by digit instead of the whole number. This issue stems from the default sorting mechanism, which treats each character independently.
Built-in Function
Although Python does not provide a built-in function specifically for natural string sorting, there are third-party libraries that can address this issue. One such library is natsort, which offers various methods to perform natural sorting.
natsort Library
Natsort provides two primary approaches for natural sorting: using a sorting function or a sorting key.
Sorting Function
To utilize the natsort sorting function, begin by importing it as follows:
from natsort import natsorted
You can then sort a list of strings using this function:
x = ['Elm11', 'Elm12', 'Elm2', 'elm0', 'elm1', 'elm10', 'elm13', 'elm9'] sorted_list = natsorted(x, key=lambda y: y.lower())
Alternatively, you can specify an algorithm to ignore case sensitivity:
sorted_list = natsorted(x, alg=ns.IGNORECASE)
Sorting Key
If you need to sort a list of strings using a sorting key, import the following:
from natsort import natsort_keygen
Creating a sorting key involves specifying a function to extract the key from each string, which is typically used when the strings have embedded data. For example:
keygen = natsort_keygen(key=lambda y: y.lower()) sorted_list = sorted(l1, key=keygen)
Other Options
For more complex natural sorting scenarios, the natsort library provides additional functionality and customization options. Please consult the library's documentation for further details. Additionally, as of version 7.1.0, natsort offers an os_sorted function to sort strings in the order of the local file system browser.
The above is the detailed content of How Can Python Efficiently Perform Natural String Sorting?. For more information, please follow other related articles on the PHP Chinese website!
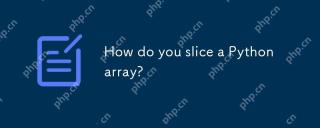
The basic syntax for Python list slicing is list[start:stop:step]. 1.start is the first element index included, 2.stop is the first element index excluded, and 3.step determines the step size between elements. Slices are not only used to extract data, but also to modify and invert lists.
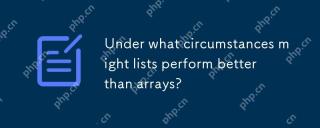
Listsoutperformarraysin:1)dynamicsizingandfrequentinsertions/deletions,2)storingheterogeneousdata,and3)memoryefficiencyforsparsedata,butmayhaveslightperformancecostsincertainoperations.
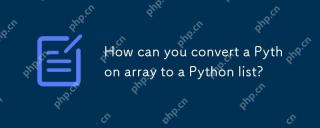
ToconvertaPythonarraytoalist,usethelist()constructororageneratorexpression.1)Importthearraymoduleandcreateanarray.2)Uselist(arr)or[xforxinarr]toconvertittoalist,consideringperformanceandmemoryefficiencyforlargedatasets.
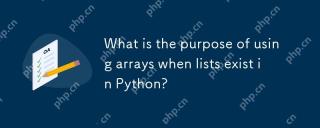
ChoosearraysoverlistsinPythonforbetterperformanceandmemoryefficiencyinspecificscenarios.1)Largenumericaldatasets:Arraysreducememoryusage.2)Performance-criticaloperations:Arraysofferspeedboostsfortaskslikeappendingorsearching.3)Typesafety:Arraysenforc
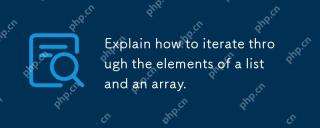
In Python, you can use for loops, enumerate and list comprehensions to traverse lists; in Java, you can use traditional for loops and enhanced for loops to traverse arrays. 1. Python list traversal methods include: for loop, enumerate and list comprehension. 2. Java array traversal methods include: traditional for loop and enhanced for loop.
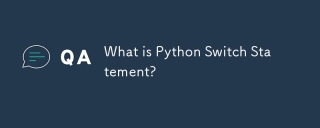
The article discusses Python's new "match" statement introduced in version 3.10, which serves as an equivalent to switch statements in other languages. It enhances code readability and offers performance benefits over traditional if-elif-el
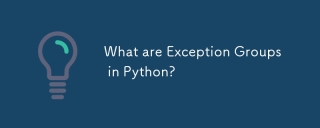
Exception Groups in Python 3.11 allow handling multiple exceptions simultaneously, improving error management in concurrent scenarios and complex operations.
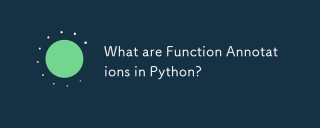
Function annotations in Python add metadata to functions for type checking, documentation, and IDE support. They enhance code readability, maintenance, and are crucial in API development, data science, and library creation.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
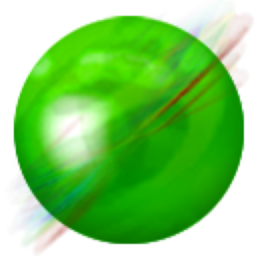
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

SublimeText3 English version
Recommended: Win version, supports code prompts!

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
