


How Can I Efficiently Determine the X and Y Coordinates of an Element in a GridLayout?
Efficiently Determining Element Coordinates Within a GridLayout
Identifying the x and y coordinates of a specific element within a GridLayout can often pose a challenge. While a common approach involves traversing a bidimensional array of buttons to establish their relationship, a more efficient method exists.
This alternative approach leverages the getComponentXIndex() and getComponentYIndex() methods of the containing component. By referencing the source of an event, these methods can swiftly provide the desired coordinates.
For instance, consider the following Java code snippet:
JButton button = (JButton) ev.getSource(); int x = this.getContentPane().getComponentXIndex(button); int y = this.getContentPane().getComponentYIndex(button);
This code effectively retrieves the x and y indices of the button based on its event source.
In the provided Java Swing Application example, the getGridButton() method plays a pivotal role in obtaining the button reference efficiently using the grid coordinates. Furthermore, the action listener demonstrates the equivalence of the clicked and found buttons.
The enhanced GridButtonPanel class exemplifies this approach, where each button is uniquely identified by its coordinates within the grid. Upon clicking any button, the code verifies the consistency between the expected and actual button references.
package gui; import java.awt.EventQueue; import java.awt.GridLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.util.ArrayList; import java.util.List; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; /** * @see http://stackoverflow.com/questions/7702697 */ public class GridButtonPanel { private static final int N = 5; private final List<jbutton> list = new ArrayList(); private JButton getGridButton(int r, int c) { int index = r * N + c; return list.get(index); } private JButton createGridButton(final int row, final int col) { final JButton b = new JButton("r" + row + ",c" + col); b.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { JButton gb = GridButtonPanel.this.getGridButton(row, col); System.out.println("r" + row + ",c" + col + " " + (b == gb) + " " + (b.equals(gb))); } }); return b; } private JPanel createGridPanel() { JPanel p = new JPanel(new GridLayout(N, N)); for (int i = 0; i <p>This enhanced approach simplifies the process of obtaining element coordinates within a GridLayout, eliminating the need for complex traversals and improving efficiency.</p></jbutton>
The above is the detailed content of How Can I Efficiently Determine the X and Y Coordinates of an Element in a GridLayout?. For more information, please follow other related articles on the PHP Chinese website!
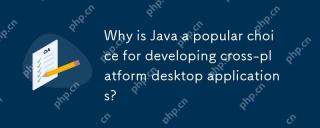
Javaispopularforcross-platformdesktopapplicationsduetoits"WriteOnce,RunAnywhere"philosophy.1)ItusesbytecodethatrunsonanyJVM-equippedplatform.2)LibrarieslikeSwingandJavaFXhelpcreatenative-lookingUIs.3)Itsextensivestandardlibrarysupportscompr
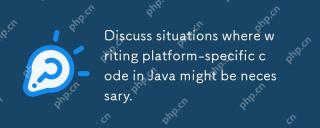
Reasons for writing platform-specific code in Java include access to specific operating system features, interacting with specific hardware, and optimizing performance. 1) Use JNA or JNI to access the Windows registry; 2) Interact with Linux-specific hardware drivers through JNI; 3) Use Metal to optimize gaming performance on macOS through JNI. Nevertheless, writing platform-specific code can affect the portability of the code, increase complexity, and potentially pose performance overhead and security risks.
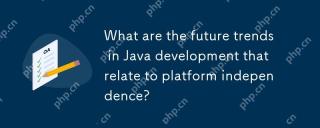
Java will further enhance platform independence through cloud-native applications, multi-platform deployment and cross-language interoperability. 1) Cloud native applications will use GraalVM and Quarkus to increase startup speed. 2) Java will be extended to embedded devices, mobile devices and quantum computers. 3) Through GraalVM, Java will seamlessly integrate with languages such as Python and JavaScript to enhance cross-language interoperability.
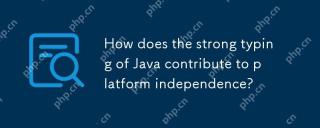
Java's strong typed system ensures platform independence through type safety, unified type conversion and polymorphism. 1) Type safety performs type checking at compile time to avoid runtime errors; 2) Unified type conversion rules are consistent across all platforms; 3) Polymorphism and interface mechanisms make the code behave consistently on different platforms.
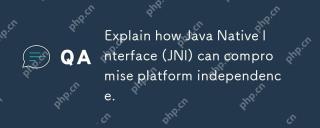
JNI will destroy Java's platform independence. 1) JNI requires local libraries for a specific platform, 2) local code needs to be compiled and linked on the target platform, 3) Different versions of the operating system or JVM may require different local library versions, 4) local code may introduce security vulnerabilities or cause program crashes.
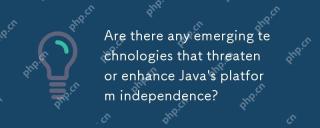
Emerging technologies pose both threats and enhancements to Java's platform independence. 1) Cloud computing and containerization technologies such as Docker enhance Java's platform independence, but need to be optimized to adapt to different cloud environments. 2) WebAssembly compiles Java code through GraalVM, extending its platform independence, but it needs to compete with other languages for performance.
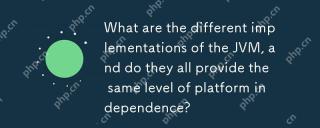
Different JVM implementations can provide platform independence, but their performance is slightly different. 1. OracleHotSpot and OpenJDKJVM perform similarly in platform independence, but OpenJDK may require additional configuration. 2. IBMJ9JVM performs optimization on specific operating systems. 3. GraalVM supports multiple languages and requires additional configuration. 4. AzulZingJVM requires specific platform adjustments.
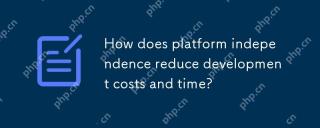
Platform independence reduces development costs and shortens development time by running the same set of code on multiple operating systems. Specifically, it is manifested as: 1. Reduce development time, only one set of code is required; 2. Reduce maintenance costs and unify the testing process; 3. Quick iteration and team collaboration to simplify the deployment process.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.
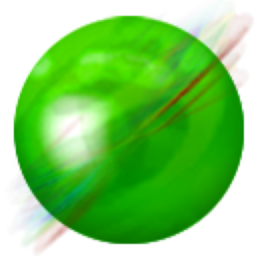
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Zend Studio 13.0.1
Powerful PHP integrated development environment

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
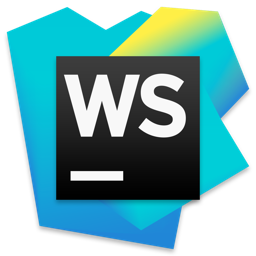
WebStorm Mac version
Useful JavaScript development tools
