


How can I send Multipart/Form-Data requests in Python using the Requests library?
Sending "Multipart/Form-Data" with Requests in Python
Multipart/form-data is a common encoding used for uploading files and other data to a web server. With the Requests library in Python, you can easily send multipart/form-data requests.
Sending Files
To send a file, you can use the files parameter of the post() method. This parameter expects a dictionary where the keys are the form field names and the values are the file objects to upload.
Sending Form Data
In addition to files, you can also send form data using the files parameter. However, it's important to note that Requests will send a multipart/form-data POST instead of the default application/x-www-form-urlencoded POST when you specify a files parameter.
To send form data using the files parameter, you can simply pass a string or bytes object as the value of the form field. For example:
import requests files = {'foo': 'bar'} response = requests.post('http://httpbin.org/post', files=files)
Customizing File Parameters
You can further control the filename, content type, and additional headers for each file by using a tuple instead of a single string or bytes object. The tuple should contain:
- Filename (optional)
- Content
- Content type (optional)
- Additional headers (optional)
For example:
files = {'foo': (None, 'bar')} # No filename parameter
Sending Multiple Fields with Same Name
You can also send multiple fields with the same name by providing a list of tuples as the value of the files parameter. For example:
files = {'foo': [(None, 'bar'), (None, 'baz')]}
Using Requests-Toolbelt
The requests-toolbelt project provides an advanced multipart encoder that simplifies the process of sending multipart/form-data requests. With this encoder, you can:
- Stream requests from open file objects
- Omit filename parameters by default
- Control the boundary used in the multipart header
For example:
from requests_toolbelt.multipart.encoder import MultipartEncoder mp_encoder = MultipartEncoder( fields={ 'foo': 'bar', 'spam': ('spam.txt', open('spam.txt', 'rb'), 'text/plain'), } ) headers = {'Content-Type': mp_encoder.content_type} response = requests.post('http://httpbin.org/post', data=mp_encoder, headers=headers)
The above is the detailed content of How can I send Multipart/Form-Data requests in Python using the Requests library?. For more information, please follow other related articles on the PHP Chinese website!
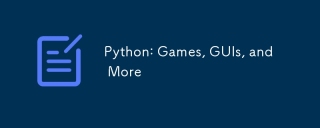
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
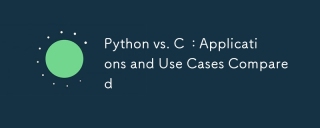
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
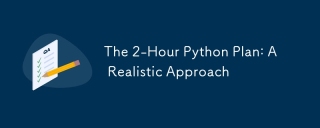
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
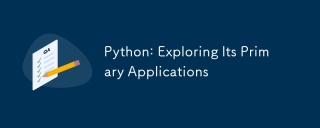
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
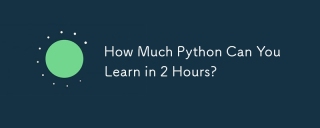
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
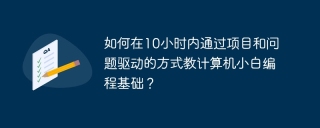
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
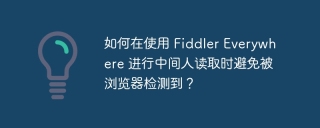
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
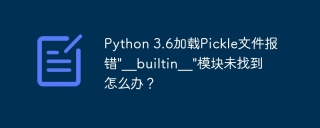
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
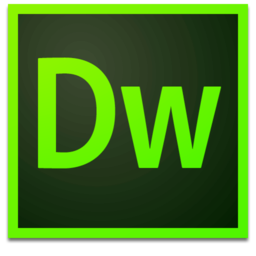
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.