Expanding Tuples into Variadic Template Function Arguments
In C 17 and later, the std::apply function provides a straightforward solution to this issue. It takes a callable object and a tuple as arguments, essentially expanding the tuple into individual arguments for the function:
#include <tuple> template<typename tret typename... t> Tret func(const T&... t); int main() { std::tuple<int float> my_tuple; auto result = std::apply(func<int int float>, my_tuple); return 0; }</int></int></typename></tuple>
In Clang versions 3.9 onwards, std::experimental::apply can be used for similar functionality.
Handling Template Function Arguments
If the template function takes variadic template arguments, a workaround can be employed:
#include <tuple> template<typename t> void my_func(T&& t) {} int main() { std::tuple<int float> my_tuple; std::apply([](auto&&... args) { my_func(args...); }, my_tuple); return 0; }</int></typename></tuple>
This approach enables template functions to accept tuple arguments, albeit with slightly less type safety. For a more general and robust solution, refer to this resource: https://blog.tartanllama.xyz/passing-overload-sets/.
The above is the detailed content of How Can I Expand Tuples into Variadic Template Function Arguments in C ?. For more information, please follow other related articles on the PHP Chinese website!
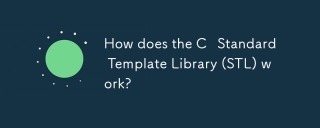
This article explains the C Standard Template Library (STL), focusing on its core components: containers, iterators, algorithms, and functors. It details how these interact to enable generic programming, improving code efficiency and readability t
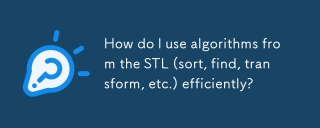
This article details efficient STL algorithm usage in C . It emphasizes data structure choice (vectors vs. lists), algorithm complexity analysis (e.g., std::sort vs. std::partial_sort), iterator usage, and parallel execution. Common pitfalls like
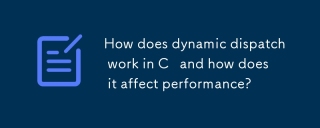
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
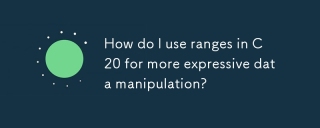
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
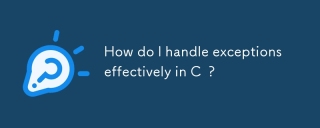
This article details effective exception handling in C , covering try, catch, and throw mechanics. It emphasizes best practices like RAII, avoiding unnecessary catch blocks, and logging exceptions for robust code. The article also addresses perf
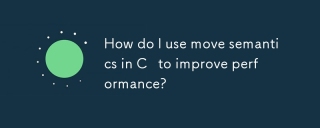
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
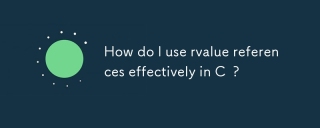
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
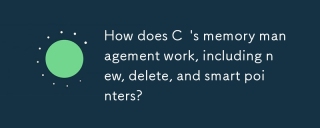
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
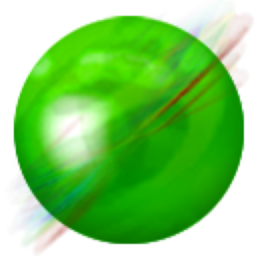
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

Atom editor mac version download
The most popular open source editor
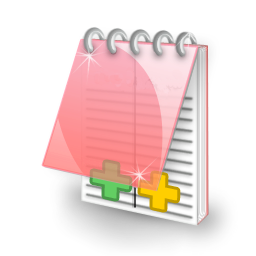
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function
