


Fixing "Variable used in lambda expression should be final or effectively final" Error
This error occurs when attempting to access a non-final variable within a lambda expression. To resolve it, the variable must be declared final or effectively final.
Effective Finality
Effective finality means the variable cannot be reassigned after being initialized. This can be achieved by:
- Declaring the variable as final in the outer scope
- Ensuring the variable is only assigned to once within the lambda
Why is this enforced?
The Java Language Specification (JLS) states that "the restriction to effectively final variables prohibits access to dynamically-changing local variables, whose capture would likely introduce concurrency problems." By enforcing this, Java prevents potential data inconsistencies and threading issues.
Example
Consider the following code:
private TimeZone extractCalendarTimeZoneComponent(Calendar cal, TimeZone calTz) { try { cal.getComponents().getComponents("VTIMEZONE").forEach(component -> { VTimeZone v = (VTimeZone) component; v.getTimeZoneId(); if (calTz == null) { calTz = TimeZone.getTimeZone(v.getTimeZoneId().getValue()); } }); } catch (Exception e) { log.warn("Unable to determine ical timezone", e); } return null; }
The calTz variable is not declared final, leading to the error. To fix it, we can modify the code as follows:
private TimeZone extractCalendarTimeZoneComponent(Calendar cal, final TimeZone calTz) { ... }
Alternatively, we can ensure calTz is only assigned to once within the lambda:
private TimeZone extractCalendarTimeZoneComponent(Calendar cal, TimeZone calTz) { ... if (calTz == null) { calTz = TimeZone.getTimeZone(v.getTimeZoneId().getValue()); } ... }
Additional Considerations
This requirement also applies to anonymous inner classes. For example, the following code would also cause the error:
new Thread(() -> { int i = 0; // Effectively final as it's only assigned once i++; // Error: cannot mutate effectively final variable }).start();
By enforcing these rules, Java helps prevent potential bugs and promotes concurrency safety in multithreaded applications.
The above is the detailed content of Why Does Java Require Variables in Lambda Expressions to Be Final or Effectively Final?. For more information, please follow other related articles on the PHP Chinese website!
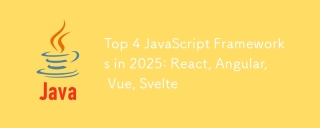
This article analyzes the top four JavaScript frameworks (React, Angular, Vue, Svelte) in 2025, comparing their performance, scalability, and future prospects. While all remain dominant due to strong communities and ecosystems, their relative popul
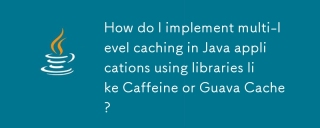
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
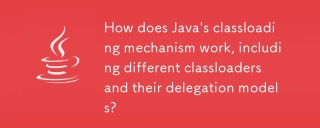
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
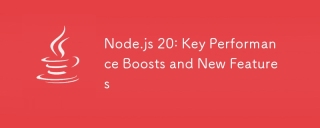
Node.js 20 significantly enhances performance via V8 engine improvements, notably faster garbage collection and I/O. New features include better WebAssembly support and refined debugging tools, boosting developer productivity and application speed.
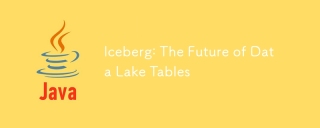
Iceberg, an open table format for large analytical datasets, improves data lake performance and scalability. It addresses limitations of Parquet/ORC through internal metadata management, enabling efficient schema evolution, time travel, concurrent w
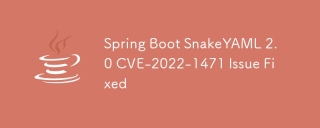
This article addresses the CVE-2022-1471 vulnerability in SnakeYAML, a critical flaw allowing remote code execution. It details how upgrading Spring Boot applications to SnakeYAML 1.33 or later mitigates this risk, emphasizing that dependency updat
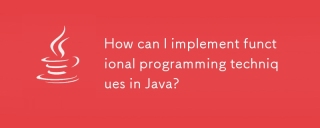
This article explores integrating functional programming into Java using lambda expressions, Streams API, method references, and Optional. It highlights benefits like improved code readability and maintainability through conciseness and immutability

The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools
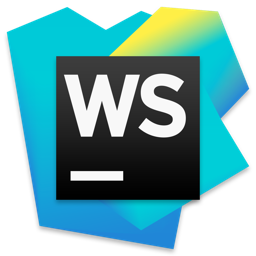
WebStorm Mac version
Useful JavaScript development tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
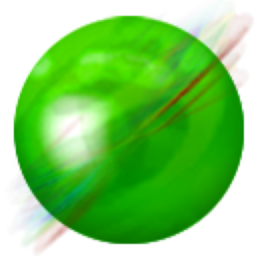
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
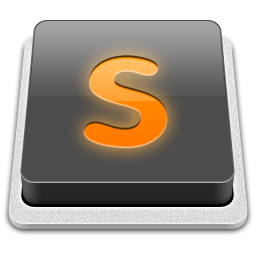
SublimeText3 Mac version
God-level code editing software (SublimeText3)

SublimeText3 English version
Recommended: Win version, supports code prompts!
