Prime Numbers:
Numbers which are divisible by 1 and itself are called as prime numbers.(Eg-->3,5,7)
1) Find prime number or not:
no = int(input("Enter no. ")) div = 2 while div<no: if no print prime break div else:> <p>Output:<br> </p> <pre class="brush:php;toolbar:false">1)Enter no. 5 Prime 2)Enter no. 6 Not Prime
2) Reversing the input number and find whether that reversed number is prime number or not:
def reverse_a_no(no): reverse = 0 while no>0: rem = no%10 reverse = (reverse*10) + rem no//=10 #no=no//10 return reverse no = int(input("Enter no. ")) reversed_no = reverse_a_no(no) #31 71 print(reversed_no) def find_prime(no): div = 2 while div<no: if no return false break div else: true result1="find_prime(no)" result2="find_prime(reversed_no)" result2: print number emirp> <p>Output:<br> </p> <pre class="brush:php;toolbar:false">1)Enter no. 15 51 EMIRP number 2)Enter no. 14 41 not EMIRP number
Perfect Number
Perfect number means sum of its divisible numbers will be equal that number.(eg-->6 is divisible by 1,2,3 and 1 2 3=6)
def find_perfect(no): total = 0 div = 1 while div<no: if no total="total" div else: no: return true false no. result="find_perfect(no)" true: print number perfect> <p>Output:<br> </p> <pre class="brush:php;toolbar:false">Enter no. 6 Perfect Number
Square root:
Find square of an input number and sum of digits of that square root number.
def square(no): return no**2 no=int(input("Enter the number:")) result=square(no) def sum_of_digits(num): sum=0 while num>0: sum=sum+num%10 num=num//10 return sum if result <p>Output:<br> </p> <pre class="brush:php;toolbar:false">Enter the number:4 7
In above example given input number is 4,
-->square root of 4 is 4x4=16
-->sum of digits of that square number 1 6=7.
Task -1 **
**Automorphic Number
Check if a number’s square ends with the same number.
Example: 5 → Automorphic (5²=25), 6 → Automorphic (6²=36), 7 → Not Automorphic.
def square(no): return no**2 no=int(input("Enter the number:")) result=square(no) print(result) while result>0: rem=result%10 if rem==no: print("Automorphic number") break else: print("Not Automorphic number") break
Output:
1)Enter the number:5 25 Automorphic number 2)Enter the number:4 16 Not Automorphic number
Task:2
Fibonacci Sequence
Generate the Fibonacci sequence up to a given number.
Example: Input: 10 → Output: 0, 1, 1, 2, 3, 5, 8.
no = int(input("Enter the number of required sequence: ")) first_num,sec_num =0 ,1 while first_num <p>Output:<br> </p> <pre class="brush:php;toolbar:false">Enter the number of required sequence: 10 0 1 1 2 3 5 8
The above is the detailed content of Python Day- Looping-Exercises and tasks. For more information, please follow other related articles on the PHP Chinese website!
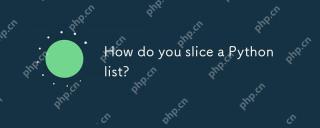
SlicingaPythonlistisdoneusingthesyntaxlist[start:stop:step].Here'showitworks:1)Startistheindexofthefirstelementtoinclude.2)Stopistheindexofthefirstelementtoexclude.3)Stepistheincrementbetweenelements.It'susefulforextractingportionsoflistsandcanuseneg

NumPyallowsforvariousoperationsonarrays:1)Basicarithmeticlikeaddition,subtraction,multiplication,anddivision;2)Advancedoperationssuchasmatrixmultiplication;3)Element-wiseoperationswithoutexplicitloops;4)Arrayindexingandslicingfordatamanipulation;5)Ag
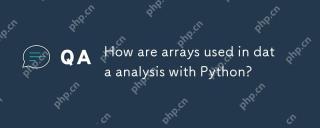
ArraysinPython,particularlythroughNumPyandPandas,areessentialfordataanalysis,offeringspeedandefficiency.1)NumPyarraysenableefficienthandlingoflargedatasetsandcomplexoperationslikemovingaverages.2)PandasextendsNumPy'scapabilitieswithDataFramesforstruc
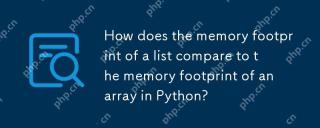
ListsandNumPyarraysinPythonhavedifferentmemoryfootprints:listsaremoreflexiblebutlessmemory-efficient,whileNumPyarraysareoptimizedfornumericaldata.1)Listsstorereferencestoobjects,withoverheadaround64byteson64-bitsystems.2)NumPyarraysstoredatacontiguou
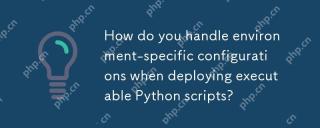
ToensurePythonscriptsbehavecorrectlyacrossdevelopment,staging,andproduction,usethesestrategies:1)Environmentvariablesforsimplesettings,2)Configurationfilesforcomplexsetups,and3)Dynamicloadingforadaptability.Eachmethodoffersuniquebenefitsandrequiresca
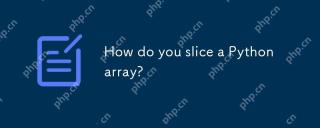
The basic syntax for Python list slicing is list[start:stop:step]. 1.start is the first element index included, 2.stop is the first element index excluded, and 3.step determines the step size between elements. Slices are not only used to extract data, but also to modify and invert lists.
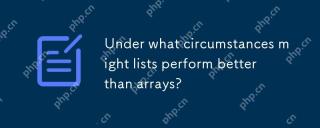
Listsoutperformarraysin:1)dynamicsizingandfrequentinsertions/deletions,2)storingheterogeneousdata,and3)memoryefficiencyforsparsedata,butmayhaveslightperformancecostsincertainoperations.
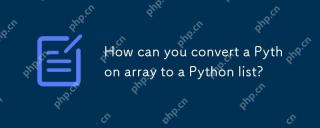
ToconvertaPythonarraytoalist,usethelist()constructororageneratorexpression.1)Importthearraymoduleandcreateanarray.2)Uselist(arr)or[xforxinarr]toconvertittoalist,consideringperformanceandmemoryefficiencyforlargedatasets.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
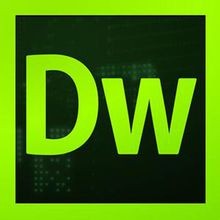
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
