Why Must `this()` and `super()` Be the First Statement in a Java Constructor?
Enforcing Constructor Execution Order: Why this() and super() Must Be the First Statement
Java strictly enforces that if this() or super() are invoked within a constructor, they must be the first statement. This restriction ensures that the constructor initialization sequence for parent and child classes occurs in the correct order.
Consider the following example:
public class MyClass { public MyClass(int x) {} } public class MySubClass extends MyClass { public MySubClass(int a, int b) { super(c); // Compile error int c = a + b; } }
The call to super(c) in the MySubClass constructor will result in a compile-time error because it is not the first statement. The compiler requires that the parent class's constructor be called first, initializing the parent class's state before the subclass constructor can execute.
One way to resolve this error is to rearrange the code:
public class MySubClass extends MyClass { public MySubClass(int a, int b) { super(a + b); // OK } }
Here, the calculation of c is performed within the argument to super(), allowing the parent class's constructor to be called first.
Another example demonstrates how the restriction prevents erroneous code from executing:
public class MyClass { public MyClass(List list) {} } public class MySubClassA extends MyClass { public MySubClassA(Object item) { List list = new ArrayList(); list.add(item); super(list); // Compile error } }
The code attempts to create a list and pass it to the parent class's constructor, which is not allowed. If the restriction were not in place, the code could fail with an error when calling methods on the parent class before it is initialized.
Enforcing this() and super() to be the first statement ensures that the constructor invocation sequence is correct, guaranteeing the proper initialization of parent and child classes in Java.
The above is the detailed content of Why Must `this()` and `super()` Be the First Statement in a Java Constructor?. For more information, please follow other related articles on the PHP Chinese website!
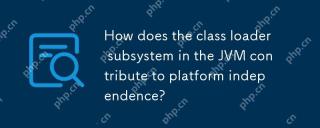
The class loader ensures the consistency and compatibility of Java programs on different platforms through unified class file format, dynamic loading, parent delegation model and platform-independent bytecode, and achieves platform independence.
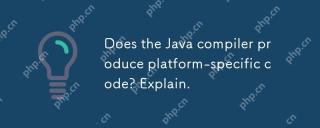
The code generated by the Java compiler is platform-independent, but the code that is ultimately executed is platform-specific. 1. Java source code is compiled into platform-independent bytecode. 2. The JVM converts bytecode into machine code for a specific platform, ensuring cross-platform operation but performance may be different.
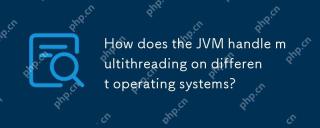
Multithreading is important in modern programming because it can improve program responsiveness and resource utilization and handle complex concurrent tasks. JVM ensures the consistency and efficiency of multithreads on different operating systems through thread mapping, scheduling mechanism and synchronization lock mechanism.
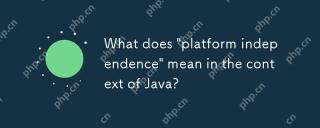
Java's platform independence means that the code written can run on any platform with JVM installed without modification. 1) Java source code is compiled into bytecode, 2) Bytecode is interpreted and executed by the JVM, 3) The JVM provides memory management and garbage collection functions to ensure that the program runs on different operating systems.
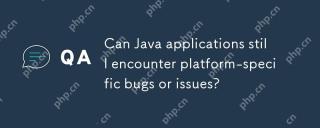
Javaapplicationscanindeedencounterplatform-specificissuesdespitetheJVM'sabstraction.Reasonsinclude:1)Nativecodeandlibraries,2)Operatingsystemdifferences,3)JVMimplementationvariations,and4)Hardwaredependencies.Tomitigatethese,developersshould:1)Conduc
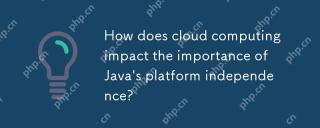
Cloud computing significantly improves Java's platform independence. 1) Java code is compiled into bytecode and executed by the JVM on different operating systems to ensure cross-platform operation. 2) Use Docker and Kubernetes to deploy Java applications to improve portability and scalability.
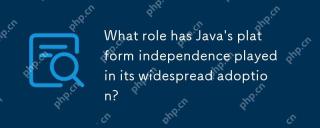
Java'splatformindependenceallowsdeveloperstowritecodeonceandrunitonanydeviceorOSwithaJVM.Thisisachievedthroughcompilingtobytecode,whichtheJVMinterpretsorcompilesatruntime.ThisfeaturehassignificantlyboostedJava'sadoptionduetocross-platformdeployment,s
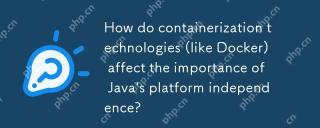
Containerization technologies such as Docker enhance rather than replace Java's platform independence. 1) Ensure consistency across environments, 2) Manage dependencies, including specific JVM versions, 3) Simplify the deployment process to make Java applications more adaptable and manageable.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
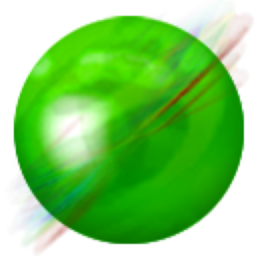
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
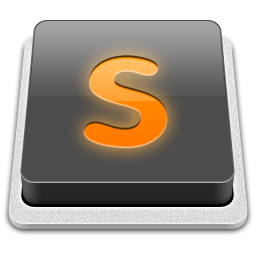
SublimeText3 Mac version
God-level code editing software (SublimeText3)

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
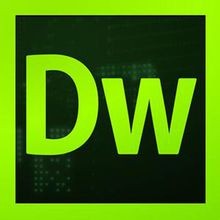
Dreamweaver CS6
Visual web development tools