


Too Many Open Files in mgo Go Server
When encountering the error "Accept error: accept tcp [::]:80: accept4: too many open files," in a MongoDB server written in Go using mgo, it indicates that the maximum number of simultaneous file descriptors has been reached. This error suggests that something is potentially being opened and not closed on every request.
Solution
The fundamental issue lies in the incorrect usage of MongoDB connections. Instead of storing an mgo.Database instance, it is essential to store an mgo.Session. When interacting with MongoDB, always acquire a copy or clone of the session and diligently close it when not needed. This approach ensures that connections are not leaked.
Furthermore, it is crucial to thoroughly check for errors in all database operations. Printing or logging errors is the minimum step to take when an error occurs.
Code Sample
Below is an improved code sample that addresses these issues:
var session *mgo.Session func init() { var err error if session, err = mgo.Dial("localhost"); err != nil { log.Fatal(err) } } func someHandler(w http.ResponseWriter, r *http.Request) { sess := session.Copy() defer sess.Close() // Must close! c := sess.DB("mapdb").C("tiles") // Do something with the collection, e.g. var tile bson.M if err := c.FindId("someTileID").One(&result); err != nil { // Tile does not exist, send back error, e.g.: log.Printf("Tile with ID not found: %v, err: %v", "someTileID", err) http.NotFound(w, r) return } // Do something with tile }
By implementing these modifications, the code effectively manages connections and ensures that they are properly closed. This resolves the issue of too many open files and improves the overall performance of the MongoDB server.
The above is the detailed content of Why Am I Getting 'Accept error: accept tcp [::]:80: accept4: too many open files' in my Go MongoDB Server?. For more information, please follow other related articles on the PHP Chinese website!
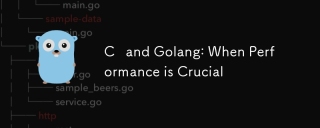
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
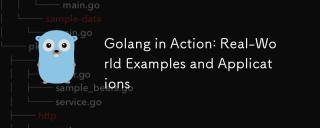
Golang excels in practical applications and is known for its simplicity, efficiency and concurrency. 1) Concurrent programming is implemented through Goroutines and Channels, 2) Flexible code is written using interfaces and polymorphisms, 3) Simplify network programming with net/http packages, 4) Build efficient concurrent crawlers, 5) Debugging and optimizing through tools and best practices.
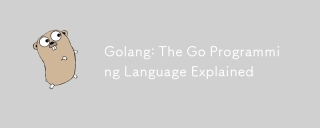
The core features of Go include garbage collection, static linking and concurrency support. 1. The concurrency model of Go language realizes efficient concurrent programming through goroutine and channel. 2. Interfaces and polymorphisms are implemented through interface methods, so that different types can be processed in a unified manner. 3. The basic usage demonstrates the efficiency of function definition and call. 4. In advanced usage, slices provide powerful functions of dynamic resizing. 5. Common errors such as race conditions can be detected and resolved through getest-race. 6. Performance optimization Reuse objects through sync.Pool to reduce garbage collection pressure.
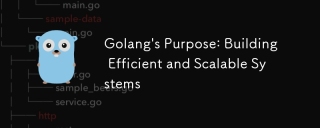
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
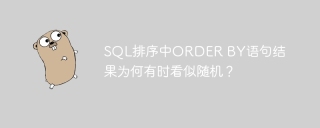
Confused about the sorting of SQL query results. In the process of learning SQL, you often encounter some confusing problems. Recently, the author is reading "MICK-SQL Basics"...
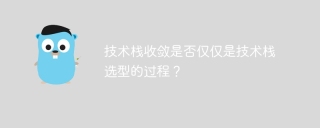
The relationship between technology stack convergence and technology selection In software development, the selection and management of technology stacks are a very critical issue. Recently, some readers have proposed...
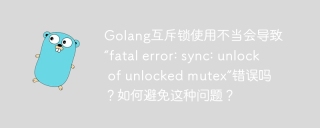
Golang ...
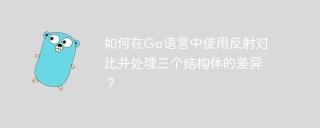
How to compare and handle three structures in Go language. In Go programming, it is sometimes necessary to compare the differences between two structures and apply these differences to the...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.

MinGW - Minimalist GNU for Windows
This project is in the process of being migrated to osdn.net/projects/mingw, you can continue to follow us there. MinGW: A native Windows port of the GNU Compiler Collection (GCC), freely distributable import libraries and header files for building native Windows applications; includes extensions to the MSVC runtime to support C99 functionality. All MinGW software can run on 64-bit Windows platforms.
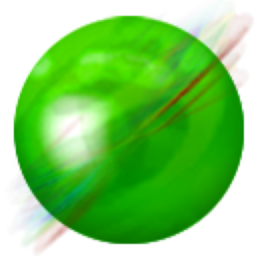
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment
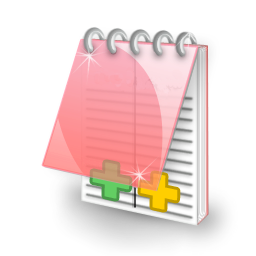
EditPlus Chinese cracked version
Small size, syntax highlighting, does not support code prompt function

Zend Studio 13.0.1
Powerful PHP integrated development environment