Buy Me a Coffee☕
*My post explains Oxford-IIIT Pet.
OxfordIIITPet() can use Oxford-IIIT Pet dataset as shown below:
*Memos:
- The 1st argument is root(Required-Type:str or pathlib.Path). *An absolute or relative path is possible.
- The 2nd argument is split(Optional-Default:"train"-Type:str). *"trainval"(3,680 images) or "test"(3,669 images) can be set to it.
- The 3rd argument is target_types(Optional-Default:"attr"-Type:str or list of str):
*Memos:
- "category", "binary-category" and/or "segmentation" can be set to it: *Memos:
- "category" is for the label from 37 categories(classes).
- "binary-category" is for the label of cat(0) or dog(1).
- "segmentation" is for a segmentation trimap image.
- An empty tuple or list can also be set to it.
- The multiple same values can be set to it.
- If the order of values is different, the order of their elements is also different.
- The 4th argument is transform(Optional-Default:None-Type:callable).
- The 5th argument is target_transform(Optional-Default:None-Type:callable).
- The 6th argument is download(Optional-Default:False-Type:bool):
*Memos:
- If it's True, the dataset is downloaded from the internet and extracted(unzipped) to root.
- If it's True and the dataset is already downloaded, it's extracted.
- If it's True and the dataset is already downloaded and extracted, nothing happens.
- It should be False if the dataset is already downloaded and extracted because it's faster.
- You can manually download and extract the dataset(images.tar.gz and annotations.tar.gz) from here to data/oxford-iiit-pet/.
- About the label from the categories(classes) for the train image indices, Abyssinian(0) is 0~49, American Bulldog(1) is 50~99, American Pit Bull Terrier(2) is 100~149, Basset Hound(3) is 150~199, Beagle(4) is 200~249, Bengal(5) is 250~299, Birman(6) is 300~349, Bombay(7) is 350~398, Boxer(8) is 399~448, British Shorthair(9) is 449~498, etc.
- About the label from the categories(classes) for the test image indices, Abyssinian(0) is 0~97, American Bulldog(1) is 98~197, American Pit Bull Terrier(2) is 198~297, Basset Hound(3) is 298~397, Beagle(4) is 398~497, Bengal(5) is 498~597, Birman(6) is 598~697, Bombay(7) is 698~785, Boxer(8) is 786~884, British Shorthair(9) is 885~984, etc.
from torchvision.datasets import OxfordIIITPet trainval_cate_data = OxfordIIITPet( root="data" ) trainval_cate_data = OxfordIIITPet( root="data", split="trainval", target_types="category", transform=None, target_transform=None, download=False ) trainval_bincate_data = OxfordIIITPet( root="data", split="trainval", target_types="binary-category" ) test_seg_data = OxfordIIITPet( root="data", split="test", target_types="segmentation" ) test_empty_data = OxfordIIITPet( root="data", split="test", target_types=[] ) test_all_data = OxfordIIITPet( root="data", split="test", target_types=["category", "binary-category", "segmentation"] ) len(trainval_cate_data), len(trainval_bincate_data) # (3680, 3680) len(test_seg_data), len(test_empty_data), len(test_all_data) # (3669, 3669, 3669) trainval_cate_data # Dataset OxfordIIITPet # Number of datapoints: 3680 # Root location: data trainval_cate_data.root # 'data' trainval_cate_data._split # 'trainval' trainval_cate_data._target_types # ['category'] print(trainval_cate_data.transform) # None print(trainval_cate_data.target_transform) # None trainval_cate_data._download # <bound method oxfordiiitpet._download of dataset oxfordiiitpet number datapoints: root location: data> len(trainval_cate_data.classes), trainval_cate_data.classes # (37, # ['Abyssinian', 'American Bulldog', 'American Pit Bull Terrier', # 'Basset Hound', 'Beagle', 'Bengal', 'Birman', 'Bombay', 'Boxer', # 'British Shorthair', ..., 'Wheaten Terrier', 'Yorkshire Terrier']) trainval_cate_data[0] # (<pil.image.image image mode="RGB" size="394x500">, 0) trainval_cate_data[1] # (<pil.image.image image mode="RGB" size="450x313">, 0) trainval_cate_data[2] # (<pil.image.image image mode="RGB" size="500x465">, 0) trainval_bincate_data[0] # (<pil.image.image image mode="RGB" size="394x500">, 0) trainval_bincate_data[1] # (<pil.image.image image mode="RGB" size="450x313">, 0) trainval_bincate_data[2] # (<pil.image.image image mode="RGB" size="500x465">, 0) test_seg_data[0] # (<pil.image.image image mode="RGB" size="300x225">, # <pil.pngimageplugin.pngimagefile image mode="L" size="300x225">) test_seg_data[1] # (<pil.image.image image mode="RGB" size="300x225">, # <pil.pngimageplugin.pngimagefile image mode="L" size="300x225">) test_seg_data[2] # (<pil.image.image image mode="RGB" size="229x300">, # <pil.pngimageplugin.pngimagefile image mode="L" size="229x300">) test_empty_data[0] # (<pil.image.image image mode="RGB" size="300x225">, None) test_empty_data[1] # (<pil.image.image image mode="RGB" size="300x225">, None) test_empty_data[2] # (<pil.image.image image mode="RGB" size="229x300">, None) test_all_data[0] # (<pil.image.image image mode="RGB" size="300x225">, # (0, 0, <pil.pngimageplugin.pngimagefile image mode="L" size="300x225">)) test_all_data[1] # (<pil.image.image image mode="RGB" size="300x225">, # (0, 0, <pil.pngimageplugin.pngimagefile image mode="L" size="300x225">)) test_all_data[2] # (<pil.image.image image mode="RGB" size="229x300">, # (0, 0, <pil.pngimageplugin.pngimagefile image mode="L" size="229x300">)) import matplotlib.pyplot as plt def show_images(data, ims, main_title=None): if len(data._target_types) == 0: plt.figure(figsize=(12, 6)) plt.suptitle(t=main_title, y=1.0, fontsize=14) for i, j in enumerate(ims, start=1): plt.subplot(2, 5, i) im, _ = data[j] plt.imshow(X=im) elif len(data._target_types) == 1: if data._target_types[0] == "category": plt.figure(figsize=(12, 6)) plt.suptitle(t=main_title, y=1.0, fontsize=14) for i, j in enumerate(ims, start=1): plt.subplot(2, 5, i) im, cate = data[j] plt.title(label=cate) plt.imshow(X=im) elif data._target_types[0] == "binary-category": plt.figure(figsize=(12, 6)) plt.suptitle(t=main_title, y=1.0, fontsize=14) for i, j in enumerate(ims, start=1): plt.subplot(2, 5, i) im, bincate = data[j] plt.title(label=bincate) plt.imshow(X=im) elif data._target_types[0] == "segmentation": plt.figure(figsize=(12, 12)) plt.suptitle(t=main_title, y=1.0, fontsize=14) for i, j in enumerate(ims, start=1): im, seg = data[j] if 1 <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/173486413998858.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="OxfordIIITPet in PyTorch"></p><p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/173486414377932.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="OxfordIIITPet in PyTorch"></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/173486414647181.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="OxfordIIITPet in PyTorch"></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/173486415046391.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="OxfordIIITPet in PyTorch"></p> <p><img src="/static/imghwm/default1.png" data-src="https://img.php.cn/upload/article/000/000/000/173486415371337.jpg?x-oss-process=image/resize,p_40" class="lazy" alt="OxfordIIITPet in PyTorch"></p> </pil.pngimageplugin.pngimagefile></pil.image.image></pil.pngimageplugin.pngimagefile></pil.image.image></pil.pngimageplugin.pngimagefile></pil.image.image></pil.image.image></pil.image.image></pil.image.image></pil.pngimageplugin.pngimagefile></pil.image.image></pil.pngimageplugin.pngimagefile></pil.image.image></pil.pngimageplugin.pngimagefile></pil.image.image></pil.image.image></pil.image.image></pil.image.image></pil.image.image></pil.image.image></pil.image.image></bound>
The above is the detailed content of OxfordIIITPet in PyTorch. For more information, please follow other related articles on the PHP Chinese website!
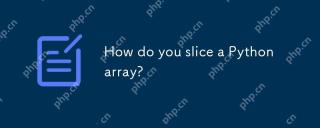
The basic syntax for Python list slicing is list[start:stop:step]. 1.start is the first element index included, 2.stop is the first element index excluded, and 3.step determines the step size between elements. Slices are not only used to extract data, but also to modify and invert lists.
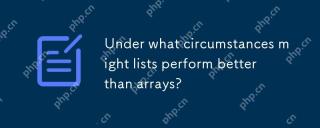
Listsoutperformarraysin:1)dynamicsizingandfrequentinsertions/deletions,2)storingheterogeneousdata,and3)memoryefficiencyforsparsedata,butmayhaveslightperformancecostsincertainoperations.
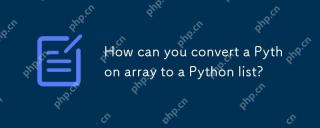
ToconvertaPythonarraytoalist,usethelist()constructororageneratorexpression.1)Importthearraymoduleandcreateanarray.2)Uselist(arr)or[xforxinarr]toconvertittoalist,consideringperformanceandmemoryefficiencyforlargedatasets.
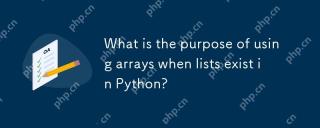
ChoosearraysoverlistsinPythonforbetterperformanceandmemoryefficiencyinspecificscenarios.1)Largenumericaldatasets:Arraysreducememoryusage.2)Performance-criticaloperations:Arraysofferspeedboostsfortaskslikeappendingorsearching.3)Typesafety:Arraysenforc
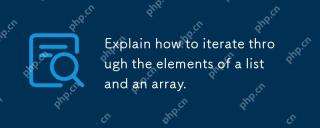
In Python, you can use for loops, enumerate and list comprehensions to traverse lists; in Java, you can use traditional for loops and enhanced for loops to traverse arrays. 1. Python list traversal methods include: for loop, enumerate and list comprehension. 2. Java array traversal methods include: traditional for loop and enhanced for loop.
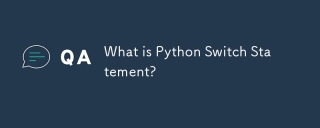
The article discusses Python's new "match" statement introduced in version 3.10, which serves as an equivalent to switch statements in other languages. It enhances code readability and offers performance benefits over traditional if-elif-el
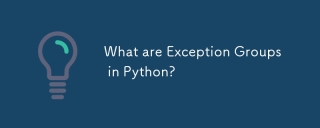
Exception Groups in Python 3.11 allow handling multiple exceptions simultaneously, improving error management in concurrent scenarios and complex operations.
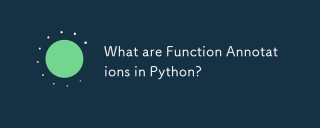
Function annotations in Python add metadata to functions for type checking, documentation, and IDE support. They enhance code readability, maintenance, and are crucial in API development, data science, and library creation.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

SublimeText3 Linux new version
SublimeText3 Linux latest version
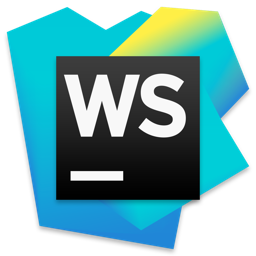
WebStorm Mac version
Useful JavaScript development tools
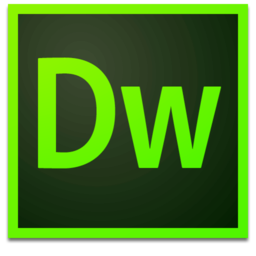
Dreamweaver Mac version
Visual web development tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

Zend Studio 13.0.1
Powerful PHP integrated development environment
