


How Can Pandas' `groupby()` Function Calculate the Sum of Values Within Groups?
Understanding GroupBy for Aggregated Calculations in Pandas
When working with large datasets, pandas offers a powerful function called groupby() to group data by specific columns and perform calculations on the grouped data. In this context, let's explore how to utilize groupby() to calculate the sum of values within groups.
Consider the following dataframe, where we have details about fruit purchases by individuals over several dates:
| Fruit | Date | Name | Number | |---|---|---|---| | Apples | 10/6/2016 | Bob | 7 | | Apples | 10/6/2016 | Bob | 8 | | Apples | 10/6/2016 | Mike | 9 | | Apples | 10/7/2016 | Steve | 10 | | Apples | 10/7/2016 | Bob | 1 | | Oranges | 10/7/2016 | Bob | 2 | | Oranges | 10/6/2016 | Tom | 15 | | Oranges | 10/6/2016 | Mike | 57 | | Oranges | 10/6/2016 | Bob | 65 | | Oranges | 10/7/2016 | Tony | 1 | | Grapes | 10/7/2016 | Bob | 1 | | Grapes | 10/7/2016 | Tom | 87 | | Grapes | 10/7/2016 | Bob | 22 | | Grapes | 10/7/2016 | Bob | 12 | | Grapes | 10/7/2016 | Tony | 15 |
Goal: Calculate the Sum of Fruit Purchases Grouped by Name
We aim to calculate the total number of fruits purchased by each individual, grouping the data by both the fruit (Fruit) and the person's name (Name).
Solution: Using GroupBy.sum()
To achieve this, we employ the groupby() function with the columns for grouping:
result = df.groupby(['Fruit', 'Name']).sum()
The sum() method applied to the grouped data automatically aggregates the values in the specified column (in this case, Number represents the number of fruits purchased).
Output:
The output of the code provides us with the aggregated values:
| | Number | |----------------|--------| | Fruit | Name | | Apples | Bob | 16 | | | Mike | 9 | | | Steve | 10 | | Grapes | Bob | 35 | | | Tom | 87 | | | Tony | 15 | | Oranges | Bob | 67 | | | Mike | 57 | | | Tom | 15 | | | Tony | 1 |
Here, we can observe the total number of fruits purchased by each individual within each fruit category. For instance, in the 'Bob' group, the total number of 'Apples' purchased is 16, and the total 'Grapes' purchased is 35.
The above is the detailed content of How Can Pandas' `groupby()` Function Calculate the Sum of Values Within Groups?. For more information, please follow other related articles on the PHP Chinese website!
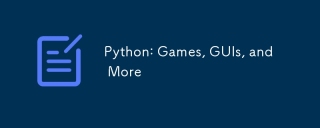
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
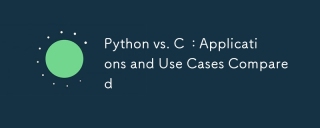
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
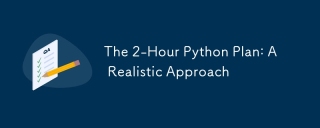
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
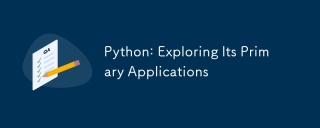
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
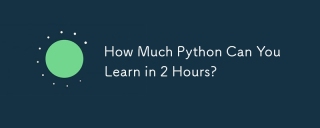
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
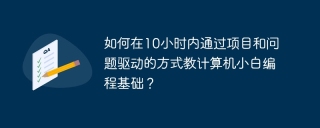
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
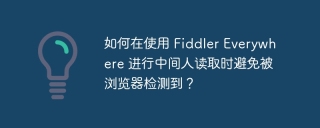
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
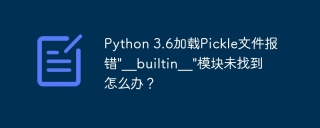
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 Chinese version
Chinese version, very easy to use

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software
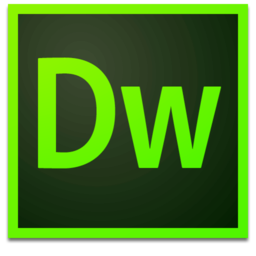
Dreamweaver Mac version
Visual web development tools

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.