PyGame - Void Display Mystery
When creating a visual application using PyGame, you may encounter instances where nothing is rendered on the screen despite drawing commands. To resolve this, it's crucial to understand the concept of display updates.
In PyGame, you draw on a Surface object associated with the display. However, these changes only become visible after updating the display using functions like pygame.display.update() or pygame.display.flip().
While pygame.display.flip() updates the entire display, pygame.display.update() can target specific areas.
To ensure the smooth operation of a PyGame application, a typical loop involves:
- Event Handling: Get user input using pygame.event.get() or pygame.event.pump().
- Game State and Object Updates: Adjust game states and object positions based on input and time.
- Display Clearing: Clear the previous frame's contents using DISPLAY.fill(0).
- Scene Rendering: Draw all necessary objects onto the canvas.
- Display Update: Update the visible display using pygame.display.update() or pygame.display.flip().
- Frame Rate Control: Limit the frames per second to avoid excessive CPU usage using pygame.time.Clock().
An example of a corrected PyGame loop:
import pygame from pygame.locals import * pygame.init() DISPLAY = pygame.display.set_mode((800,800)) pygame.display.set_caption("thing") clock = pygame.time.Clock() run = True while run: # Handle events for event in pygame.event.get(): if event.type == QUIT: run = False # Clear display DISPLAY.fill(0) # Draw scene pygame.draw.rect(DISPLAY, (200, 200, 200), pygame.Rect(0, 400, 800, 400)) # Update display pygame.display.flip() # Limit frames per second clock.tick(60) pygame.quit() exit()
This should resolve the issue of an empty screen and ensure proper rendering in PyGame.
The above is the detailed content of Why Is My PyGame Display Blank?. For more information, please follow other related articles on the PHP Chinese website!
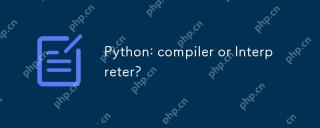
Python is an interpreted language, but it also includes the compilation process. 1) Python code is first compiled into bytecode. 2) Bytecode is interpreted and executed by Python virtual machine. 3) This hybrid mechanism makes Python both flexible and efficient, but not as fast as a fully compiled language.
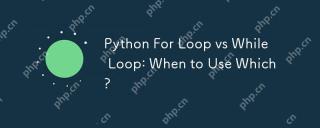
Useaforloopwheniteratingoverasequenceorforaspecificnumberoftimes;useawhileloopwhencontinuinguntilaconditionismet.Forloopsareidealforknownsequences,whilewhileloopssuitsituationswithundeterminediterations.
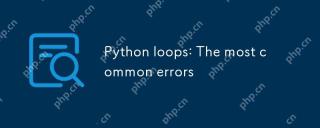
Pythonloopscanleadtoerrorslikeinfiniteloops,modifyinglistsduringiteration,off-by-oneerrors,zero-indexingissues,andnestedloopinefficiencies.Toavoidthese:1)Use'i
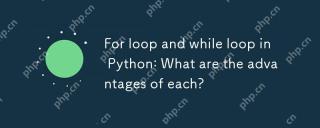
Forloopsareadvantageousforknowniterationsandsequences,offeringsimplicityandreadability;whileloopsareidealfordynamicconditionsandunknowniterations,providingcontrolovertermination.1)Forloopsareperfectforiteratingoverlists,tuples,orstrings,directlyacces
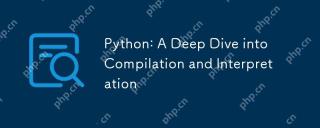
Pythonusesahybridmodelofcompilationandinterpretation:1)ThePythoninterpretercompilessourcecodeintoplatform-independentbytecode.2)ThePythonVirtualMachine(PVM)thenexecutesthisbytecode,balancingeaseofusewithperformance.
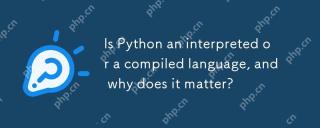
Pythonisbothinterpretedandcompiled.1)It'scompiledtobytecodeforportabilityacrossplatforms.2)Thebytecodeistheninterpreted,allowingfordynamictypingandrapiddevelopment,thoughitmaybeslowerthanfullycompiledlanguages.
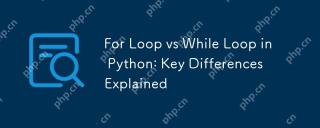
Forloopsareidealwhenyouknowthenumberofiterationsinadvance,whilewhileloopsarebetterforsituationswhereyouneedtoloopuntilaconditionismet.Forloopsaremoreefficientandreadable,suitableforiteratingoversequences,whereaswhileloopsoffermorecontrolandareusefulf
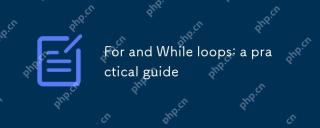
Forloopsareusedwhenthenumberofiterationsisknowninadvance,whilewhileloopsareusedwhentheiterationsdependonacondition.1)Forloopsareidealforiteratingoversequenceslikelistsorarrays.2)Whileloopsaresuitableforscenarioswheretheloopcontinuesuntilaspecificcond


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools
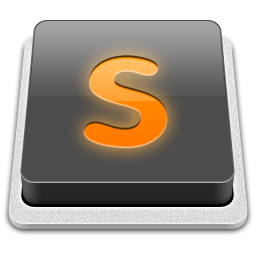
SublimeText3 Mac version
God-level code editing software (SublimeText3)
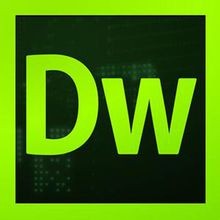
Dreamweaver CS6
Visual web development tools
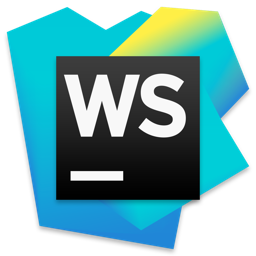
WebStorm Mac version
Useful JavaScript development tools

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
