


How to Repeatedly Execute a Python Function Without a Cron Job or Resource Exhaustion?
How to Execute a Function Repeatedly Without Cron
Python enthusiasts often face the challenge of perpetually executing a function at specified intervals without the need for complex cron setups. One straightforward approach involves utilizing a simple while loop.
while True: # Code executed here time.sleep(60)
This code appears to serve the purpose of continuously executing a function every 60 seconds. However, potential pitfalls exist within this approach. As the loop keeps running endlessly, there's a risk of resource exhaustion, causing sluggish performance or system crashes.
To gracefully resolve these issues, consider employing an event scheduler such as the sched module in Python.
import sched, time def do_something(scheduler): # schedule the next call first scheduler.enter(60, 1, do_something, (scheduler,)) print("Doing stuff...") # then do your stuff my_scheduler = sched.scheduler(time.time, time.sleep) my_scheduler.enter(60, 1, do_something, (my_scheduler,)) my_scheduler.run()
The sched module enables the scheduling of tasks with intricate control over their execution times. In this scenario, do_something() functions as a callback function that reschedules itself every 60 seconds and simultaneously performs the intended task. This mechanism ensures continuous execution of the function at the specified interval without obstructing the program's responsiveness.
In essence, leveraging event schedulers provides a more robust and resource-efficient solution for repeatedly executing functions in Python without the limitations of while loops.
The above is the detailed content of How to Repeatedly Execute a Python Function Without a Cron Job or Resource Exhaustion?. For more information, please follow other related articles on the PHP Chinese website!
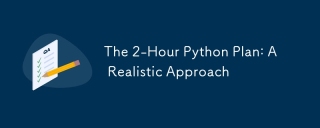
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
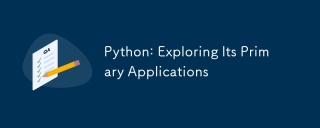
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
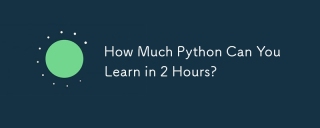
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
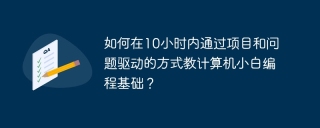
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
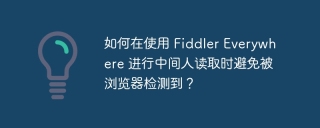
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
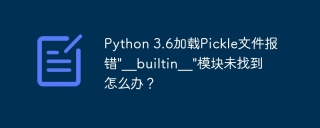
Error loading Pickle file in Python 3.6 environment: ModuleNotFoundError:Nomodulenamed...
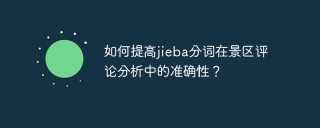
How to solve the problem of Jieba word segmentation in scenic spot comment analysis? When we are conducting scenic spot comments and analysis, we often use the jieba word segmentation tool to process the text...

How to use regular expression to match the first closed tag and stop? When dealing with HTML or other markup languages, regular expressions are often required to...


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

MantisBT
Mantis is an easy-to-deploy web-based defect tracking tool designed to aid in product defect tracking. It requires PHP, MySQL and a web server. Check out our demo and hosting services.
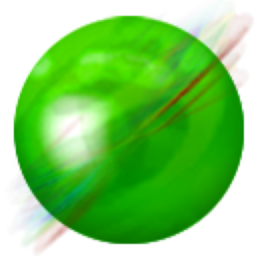
ZendStudio 13.5.1 Mac
Powerful PHP integrated development environment

SublimeText3 Chinese version
Chinese version, very easy to use

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool

SecLists
SecLists is the ultimate security tester's companion. It is a collection of various types of lists that are frequently used during security assessments, all in one place. SecLists helps make security testing more efficient and productive by conveniently providing all the lists a security tester might need. List types include usernames, passwords, URLs, fuzzing payloads, sensitive data patterns, web shells, and more. The tester can simply pull this repository onto a new test machine and he will have access to every type of list he needs.