


Default Values for Struct Fields
Go initializes struct fields with default values based on their data type. For example, integer fields are initialized to 0. However, this default value can sometimes be a valid value, making it challenging to distinguish between fields that have not been explicitly set and those that have been initialized by default.
Example:
type test struct { testIntOne int testIntTwo int } func main() { s := test{testIntOne: 0} // Initializes testIntOne to 0 // How can we determine whether testIntOne has been set explicitly or initialized to 0? }
inability to distinguish values
Unfortunately, Go has no built-in way to distinguish between uninitialized fields and fields initialized to a default value.
Solutions:
To solve this problem, consider several alternative approaches:
1. Using Pointers
Pointers have a null value nil, which is different from 0. By initializing pointer fields, we can easily check whether they have been set.
type test struct { testIntOne *int testIntTwo *int } func main() { s := test{testIntOne: new(int)} // new() возвращает указатель, инициализированный до нуля fmt.Println("testIntOne set:", s.testIntOne != nil) // Выведет true, так как testIntOne инициализирован fmt.Println("testIntTwo set:", s.testIntTwo != nil) // Выведет false, так как testIntTwo не инициализирован }
2 . Using Methods
You can also use methods to control the initialization of fields. In this case, we can track whether a flag has been set indicating whether the field was manually initialized.
type test struct { testIntOne int testIntTwo int oneSet, twoSet bool // Флаги для отслеживания инициализации } func (t *test) SetOne(i int) { t.testIntOne, t.oneSet = i, true // Установка поля и флага } func (t *test) SetTwo(i int) { t.testIntTwo, t.twoSet = i, true // Установка поля и флага } func main() { s := test{} s.SetOne(0) // Вызов метода для инициализации fmt.Println("testIntOne set:", s.oneSet) // Выведет true fmt.Println("testIntTwo set:", s.twoSet) // Выведет false }
The above is the detailed content of How Can We Distinguish Between Explicitly Set and Default-Initialized Struct Fields in Go?. For more information, please follow other related articles on the PHP Chinese website!
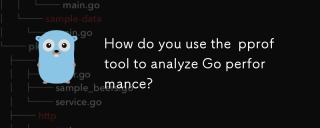
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
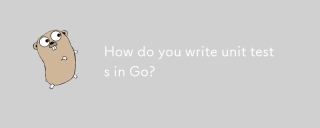
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
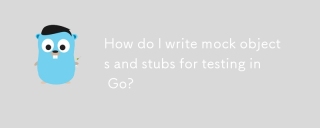
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
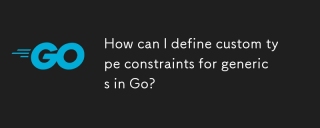
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
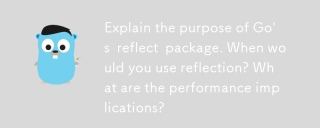
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
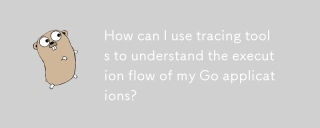
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
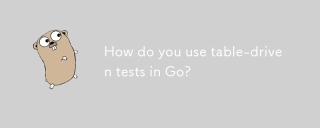
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
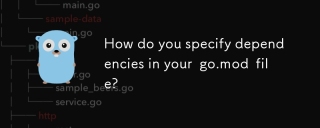
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

SublimeText3 English version
Recommended: Win version, supports code prompts!

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),

Notepad++7.3.1
Easy-to-use and free code editor

PhpStorm Mac version
The latest (2018.2.1) professional PHP integrated development tool
