


Reading a Single Character from User Input Cross-Platform
In various programming scenarios, it becomes necessary to read a single character from user input without buffering or echoing. This functionality is similar to the renowned getch() function available in Windows, but a universal approach that works across multiple platforms is desired.
Solution:
To achieve this cross-platform character reading, you can utilize a community-driven Python recipe from the ActiveState Recipes site: "getch()-like unbuffered character reading from stdin on both Windows and Unix." This recipe provides a class-based implementation, catering to both Windows and Unix-based systems.
Implementation:
The following code snippet demonstrates the implementation of the cross-platform character reading class:
class _Getch: """Gets a single character from standard input. Does not echo to the screen.""" def __init__(self): try: self.impl = _GetchWindows() except ImportError: self.impl = _GetchUnix() def __call__(self): return self.impl() class _GetchUnix: def __init__(self): import tty, sys def __call__(self): import sys, tty, termios fd = sys.stdin.fileno() old_settings = termios.tcgetattr(fd) try: tty.setraw(sys.stdin.fileno()) ch = sys.stdin.read(1) finally: termios.tcsetattr(fd, termios.TCSADRAIN, old_settings) return ch class _GetchWindows: def __init__(self): import msvcrt def __call__(self): import msvcrt return msvcrt.getch() getch = _Getch()
To utilize this class, simply instantiate the _Getch class and call it. The result will be the single character typed by the user, without any buffering or echoing. For instance:
result = getch()
Conclusion:
This cross-platform character reading approach is widely applicable in situations where immediate user input is required, such as capturing keystrokes in terminal applications or implementing interactive user interfaces.
The above is the detailed content of How to Read a Single Character from User Input Across Different Platforms?. For more information, please follow other related articles on the PHP Chinese website!
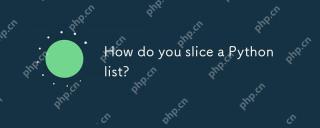
SlicingaPythonlistisdoneusingthesyntaxlist[start:stop:step].Here'showitworks:1)Startistheindexofthefirstelementtoinclude.2)Stopistheindexofthefirstelementtoexclude.3)Stepistheincrementbetweenelements.It'susefulforextractingportionsoflistsandcanuseneg

NumPyallowsforvariousoperationsonarrays:1)Basicarithmeticlikeaddition,subtraction,multiplication,anddivision;2)Advancedoperationssuchasmatrixmultiplication;3)Element-wiseoperationswithoutexplicitloops;4)Arrayindexingandslicingfordatamanipulation;5)Ag
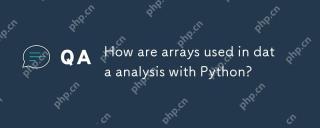
ArraysinPython,particularlythroughNumPyandPandas,areessentialfordataanalysis,offeringspeedandefficiency.1)NumPyarraysenableefficienthandlingoflargedatasetsandcomplexoperationslikemovingaverages.2)PandasextendsNumPy'scapabilitieswithDataFramesforstruc
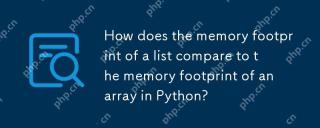
ListsandNumPyarraysinPythonhavedifferentmemoryfootprints:listsaremoreflexiblebutlessmemory-efficient,whileNumPyarraysareoptimizedfornumericaldata.1)Listsstorereferencestoobjects,withoverheadaround64byteson64-bitsystems.2)NumPyarraysstoredatacontiguou
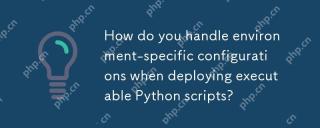
ToensurePythonscriptsbehavecorrectlyacrossdevelopment,staging,andproduction,usethesestrategies:1)Environmentvariablesforsimplesettings,2)Configurationfilesforcomplexsetups,and3)Dynamicloadingforadaptability.Eachmethodoffersuniquebenefitsandrequiresca
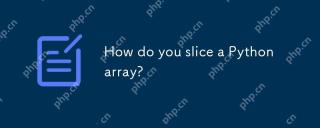
The basic syntax for Python list slicing is list[start:stop:step]. 1.start is the first element index included, 2.stop is the first element index excluded, and 3.step determines the step size between elements. Slices are not only used to extract data, but also to modify and invert lists.
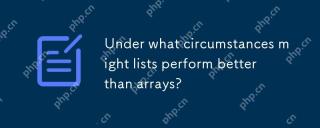
Listsoutperformarraysin:1)dynamicsizingandfrequentinsertions/deletions,2)storingheterogeneousdata,and3)memoryefficiencyforsparsedata,butmayhaveslightperformancecostsincertainoperations.
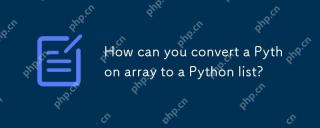
ToconvertaPythonarraytoalist,usethelist()constructororageneratorexpression.1)Importthearraymoduleandcreateanarray.2)Uselist(arr)or[xforxinarr]toconvertittoalist,consideringperformanceandmemoryefficiencyforlargedatasets.


Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

DVWA
Damn Vulnerable Web App (DVWA) is a PHP/MySQL web application that is very vulnerable. Its main goals are to be an aid for security professionals to test their skills and tools in a legal environment, to help web developers better understand the process of securing web applications, and to help teachers/students teach/learn in a classroom environment Web application security. The goal of DVWA is to practice some of the most common web vulnerabilities through a simple and straightforward interface, with varying degrees of difficulty. Please note that this software

Safe Exam Browser
Safe Exam Browser is a secure browser environment for taking online exams securely. This software turns any computer into a secure workstation. It controls access to any utility and prevents students from using unauthorized resources.

mPDF
mPDF is a PHP library that can generate PDF files from UTF-8 encoded HTML. The original author, Ian Back, wrote mPDF to output PDF files "on the fly" from his website and handle different languages. It is slower than original scripts like HTML2FPDF and produces larger files when using Unicode fonts, but supports CSS styles etc. and has a lot of enhancements. Supports almost all languages, including RTL (Arabic and Hebrew) and CJK (Chinese, Japanese and Korean). Supports nested block-level elements (such as P, DIV),
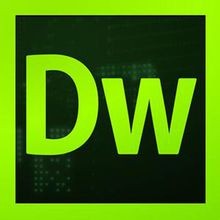
Dreamweaver CS6
Visual web development tools

SAP NetWeaver Server Adapter for Eclipse
Integrate Eclipse with SAP NetWeaver application server.
